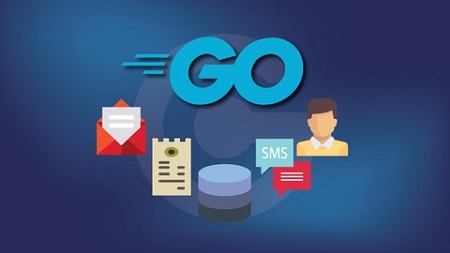
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 123 lectures (10h 44m) | 3.72 GB
Build highly available, scalable, resilient distributed applications using Go
For a long time, web applications were usually a single application that handled everything—in other words, a monolithic application. This monolith handled user authentication, logging, sending email, and everything else. While this is still a popular (and useful) approach, today, many larger scale applications tend to break things up into microservices. Today, most large organizations are focused on building web applications using this approach, and with good reason.
Microservices, also known as the microservice architecture, are an architectural style which structures an application as a loosely coupled collection of smaller applications. The microservice architecture allows for the rapid and reliable delivery of large, complex applications. Some of the most common features for a microservice are:
- it is maintainable and testable;
- it is loosely coupled with other parts of the application;
- it can deployed by itself;
- it is organized around business capabilities;
- it is often owned by a small team.
In this course, we’ll develop a number of small, self-contained, loosely coupled microservices that will will communicate with one another and a simple front-end application with a REST API, with RPC, over gRPC, and by sending and consuming messages using AMQP, the Advanced Message Queuing Protocol. The microservices we build will include the following functionality:
- An Authentication service, with a Postgres database;
- A Logging service, with a MongoDB database;
- A Listener service, which receives messages from RabbitMQ and acts upon them;
- A Broker service, which is an optional single point of entry into the microservice cluster;
- A Mail service, which takes a JSON payload, converts into a formatted email, and send it out.
All of these services will be written in Go, commonly referred to as Golang, a language which is particularly well suited to building distributed web applications.
We’ll also learn how to deploy our distributed application to a Docker Swarm and Kubernetes, and how to scale up and down, as necessary, and to update individual microservices with little or no downtime.
What you’ll learn
- Learn what Microservices are and when to use them
- Develop loosely coupled, single purpose applications that work together
- Communicate between services using JSON, Remote Procedure Calls, and gRPC
- Learn how to implement service discovery using etcd
- Learn how to push events to microservices using the Advanced Message Queuing Protocol (AMQP) using RabbitMQ
Table of Contents
Introduction
1 Introduction
2 About me
3 Installing Go
4 Installing Visual Studio Code
5 Installing Make
6 Installing Docker
7 Asking for help
8 Mistakes. We all make them.
Building a simple front end and one Microservice
9 What we’ll cover in this section
10 Setting up the front end
11 Reviewing the front end code
12 Our first service the Broker
13 Building a docker image for the Broker service
14 Adding a button and JavaScript to the front end
15 Creating some helper functions to deal with JSON and such
16 Simplifying things with a Makefile (Mac & Linux)
17 Simplifying things with a Makefile (Windows)
Building an Authentication Service
18 What we’ll cover in this section
19 Setting up a stub Authentication service
20 Creating and connecting to Postgres from the Authentication service
21 A note about PostgreSQL
22 Updating our docker-compose.yml for Postgres and the Authentication service
23 Populating the Postgres database
24 Adding a route and handler to accept JSON
25 Update the Broker for a standard JSON format, and conect to our Auth service
26 Updating the front end to authenticate thorough the Broker and trying things out
Building a Logger Service
27 What we’ll cover in this section
28 Getting started with the Logger service
29 Setting up the Logger data models
30 Finishing up the Logger data models
31 Setting up routes, handlers, helpers, and a web server in our logger-service
32 Adding MongoDB to our docker-compose.yml file
33 Add the logger-service to docker-compose.yml and the Makefile
34 Adding a route and handler on the Broker to communicate with the logger service
35 Update the front end to post to the logger, via the broker
36 Add basic logging to the Authentication service
37 Trying things out
Building a Mail Service
38 What we’ll cover in this section
39 Adding Mailhog to our docker-compose.yml
40 Setting up a stub Mail microservice
41 Building the logic to send email
42 Building the routes, handlers, and email templates
43 Challenge Adding the Mail service to docker-compose.yml and the Makefile
44 Solution to challenge
45 Modifying the Broker service to handle mail
46 Updating the front end to send mail
47 A note about mail and security
Building a Listener service AMQP with RabbitMQ
48 What we’ll cover in this section
49 Creating a stub Listener service
50 Adding RabbitMQ to our docker-compose.yml
51 Connecting to RabbitMQ
52 Writing functions to interact with RabbitMQ
53 Adding a logEvent function to our Listener microservice
54 Updating main.go to start the Listener function
55 Change the RabbitMQ server URL to the Docker address
56 Creating a Docker image and updating the Makefile
57 Updating the broker to interact with RabbitMQ
58 Writing logic to Emit events to RabbitMQ
59 Adding a new function in the Broker to log items via RabbitMQ
60 Trying things out
Communicating between services using Remote Procedure Calls (RPC)
61 What we’ll cover in this section
62 Setting up an RPC server in the Logger microservice
63 Listening for RPC calls in the Logger microservice
64 Calling the Logger from the Broker using RPC
65 Trying things out
Speeding things up (potentially) with gRPC
66 What we’ll cover in this section
67 Installing the necessary tools for gRPC
68 Defining a Protocol for gRPC the .proto file
69 Generating the gRPC code from the command line
70 Getting started with the gRPC server
71 Listening for gRPC connections in the Logger microservice
72 Writing the client code
73 Updating the front end code
74 Trying things out
Deploying our Distributed App using Docker Swarm
75 What we’ll cover in this section
76 Building images for our microservices
77 Creating a Docker swarm deployment file
78 Initalizing and starting Docker Swarm
79 Starting the front end and hitting our swarm
80 Scaling services
81 Updating services
82 Stopping Docker swarm
83 Updating the Broker service, and creating a Dockerfile for the front end
84 Solution to the Challenge
85 Adding the Front end to our swarm.yml deployment file
86 Adding Caddy to the mix as a Proxy to our front end and the broker
87 Modifying our hosts file to add a backend entry and bringing up our swarm
88 Challenge correcting the URL to the broker service in the front end
89 Solution to challenge
90 Updating Postgres to 14.2 – why monitoring is important!
91 Spinning up two new servers on Linode
92 Setting up a non-root account and putting a firewall in place.
93 Installing Docker on the servers
94 Setting the hostname for our server
95 Adding DNS entries for our servers
96 Adding a DNS entry for the Broker service
97 Initializing a manager, and adding a worker
98 Updating our swarm.yml and Caddy dockerfile for production
99 Trying things out, and correcting some mistakes
100 Populating the remote database using an SSH tunnel
101 Enabling SSL certificates on the Caddy microservice
Deploying our Distributed App to Kubernetes
102 What we’ll cover in this section
103 Installing minikube
104 Installing kubectl
105 Initializing a cluster
106 Bringing up the k8s dashboard
107 Creating a deployment file for Mongo
108 Creating a deployment file for RabbitMQ
109 Creating a deployment file for the Broker service
110 When things go wrong…
111 Creating a deployment file for MailHog
112 Creating a deployment file for the Mail microservice
113 Creating a deployment file for the Logger service
114 Creating a deployment file for the Listener service
115 Running Postgres on the host machine, so we can connect to it from k8s
116 Creating a deployment file for the Authentication service
117 Trying things out by adding a LoadBalancer service
118 Creating a deployment file for the Front End microservice
119 Adding an nginx Ingress to our cluster
120 Trying out our Ingress
121 Scaling services
122 Updating services
123 Deploying to cloud services
Resolve the captcha to access the links!