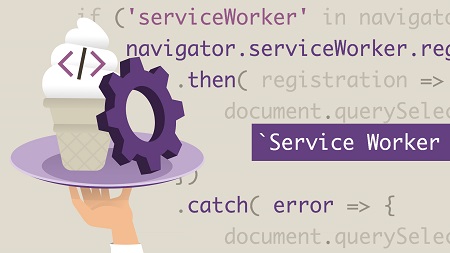
English | MP4 | AVC 1280×720 | AAC 48KHz 2ch | 3h 36m | 738 MB
Service workers are background scripts that enable features like offline storage, push notifications, and background syncing. They are an important tool for the modern web developer who wants to create faster, more interactive experiences with offline browsing and messaging. This course shows how to implement service workers in vanilla JavaScript—leaving frameworks behind in order to understand the technology’s full potential. Learn how to install and register service workers, handle events, and manage updates. Then find how to implement several use cases for service workers: intercepting and routing network requests as proxy server, storing files in an offline cache, sending and receiving messages, and synchronizing background data. Plus, get tips and tricks to optimize the performance of your applications using service workers.
Topics include:
- Service worker life cycle
- Registering service workers
- Handling service worker events
- Updating service workers
- Acting as a network proxy
- Configuring cache storage
- Communicating with clients
- Optimizing web performance
Table of Contents
1 Implement service workers in JavaScript
2 What you should know
3 Hello, service workers
4 Abilities
5 What we can do
6 Requirements and compatibility
7 Service workers in action
8 Understand the life cycle
9 See all service worker registrations
10 Understand the scope
11 Learn vocabulary and concepts
12 Register a service worker
13 Change the default scope
14 Handle service worker events
15 Debug with Developer Tools in Chrome
16 Debug with other browsers
17 Load external files
18 Work with the registration
19 Update the service worker
20 Manage updates in your code
21 Unregister the service worker
22 Capture the fetch event
23 Synthesize a response
24 The Response object in detail
25 The Request object in detail
26 Work with exact routes
27 Work with dynamic routes
28 Clone before reading data
29 Understand the new Cache API
30 Prepare the project
31 Prefetch files on install
32 Visualize your cache
33 Implement a cache-first policy
34 Implement a network-first policy
35 Cache new elements after install
36 Use stale-while-revalidate
37 Deliver alternate content when offline
38 Keep your storage clean
39 Summary of cache strategies
40 What’s available in the service worker
41 Send messages from pages
42 Receive messages in the service worker
43 Broadcast messages to clients
44 Receive messages from the controller
45 Sync data in the background
46 Readable streams
47 Navigation preload
48 Defer service worker registration
49 Make your service worker useful quickly
50 Next steps
Resolve the captcha to access the links!