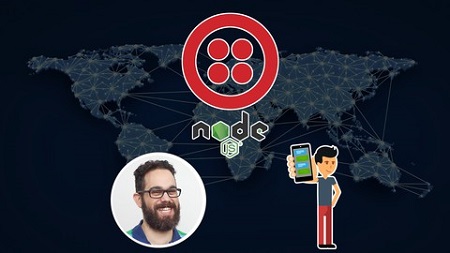
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 3 Hours | 1.62 GB
Build a complete SMS booking system using Twilio and Node.Js that receives and send messages to create a new appointment
Twilio the world’s leading cloud communications platform as a service (CPaaS) that enables you to develop SMS solutions, WhatsApp, Voice, Video, email, and even IoT. Twilio powers communications for more than 190,000 businesses and enables nearly 932 billion human interactions every year.
This course will leverage some of those capabilities to create a fully functional SMS booking system. Here is how it works, the customer interested in booking an appointment send an SMS to a Twilio number; our Node.JS backend application understand the message and send an SMS back to the customer saying:
Hi, do you want to book an appointment to:
- see the gym
- book a personal trainer
- book a massage
After that, we wait for a user reply, such as:
I want to book a massage
After that, our Node application will send an SMS to the user asking:
What date do you want to see the masseur
The customer will reply with a preferred day, such as:
Monday, please
In this case, we will reply with the valid times available on Monday
Do you want to book it on Monday: 10 am, 11 am, 1 pm or 4 pm
After the customer selects the best time for the booking, they will reply saying:
At 11 am is good for me
After collecting all the data, the NodeJS application will send a confirmation to the customer saying:
Your appointment is booked to see the masseur on Monday at 11 am. See you than
Please note all the data above is fictional and hardcoded to the application. The course focuses on sending and receiving the SMSes, leaving all the logic of checking the actual dates and times and finalizing the booking for you to implement.
Said that new Chapters are coming, and as a student, you can help me decide what comes next. So far few ideas I have on a road map are:
- Add the logic to read/write on the database been able to validate bookings and available times.
- Add a relational database to the application as a Docker container and add the current application to a container with easier development and deployment.
- Add an option to cancel a booking.
- Add an option to make a payment via SMS using stripe.
- Move the NodeJS code to a Serverless function and host it in Twilio.
- Any amazing idea you may have.
What you’ll learn
- How to create a complete SMS booking system using Twilio and Node
- How to send SMSes
- How to receive SMSes
- How to use Twilio to send SMS
- How to use NodeJs to manage send and receive SMS
Table of Contents
Introduction
1 Before we signup to Twilio
2 Creating a Twilio Account
3 Buying a Twilio Number (for free)
4 Creating the API key and secrete to access Twilio safely
5 Initialising the Node.js backend with Twilio, Express and dotEnv (.env)
6 Setting up Twilio and dotEnv (.env)
Sending SMS
7 Sending our first SMS
Understand the express server backend
8 Setting up the express server
9 Nodemon understanding devDependancies vs Global dependancies
LocalTunnel, your localhost open to the world
10 Installing and understanding LocalTunnel
Understanding Weebhooks
11 Configuring Twilio Webhooks
Receiving SMS
12 Receiving our first SMS message
Tracking the SMS conversation
13 Setting up cookies and the reply message
14 Verifying a new phone number with Twilio
15 Keeping track of our messages
Gathering all the booking details
16 Counting the steps
17 Booking an appointment type
18 Getting the day for the booking
19 Testing the application and fixing small mistakes
20 Creating a RegEx for to get the time requested
21 Getting the appoint time
22 Confirming the appointments
Refactoring, it is the same code, but much nicer
23 Refactoring part 1
24 Refactoring part 2
25 Using guard to make our code easier to understand
Where to go from here
26 Where to go from here
Appendix 1
27 Installing VSCode modules
28 Configuring VSCode Prettier
29 Emmet and speed up coding
30 Add extension tools for chrome
31 Installing Docker Extension
32 Connecting to MongoDB inside Docker using Compass
33 Using MongoDB Compass – Update, Delete, Clone, Drop Collections and Databases
Appendix 1.1 Mac Specific Setup, Configurations, Installations and etc
34 Installing Node, Chrome, VSCode, Yarn
35 Installing Brew on Mac
36 Installing Deno and Denon on Mac
37 Installing Docker
38 Install Postman
39 MongoDB Compass
Appendix 1.2 Windows Specific Setup, Configurations, Installations and etc
40 Installing Visual Code Insiders
41 Installing Chrome Canary
42 Installing Node
43 Installing Postman
44 Installing Compass
45 Installing Docker
46 Installing Hyper-V for Docker (optional)
47 Installing Linux Kernel Update Package (WSL 2) on windows for Docker (optional)
Resolve the captcha to access the links!