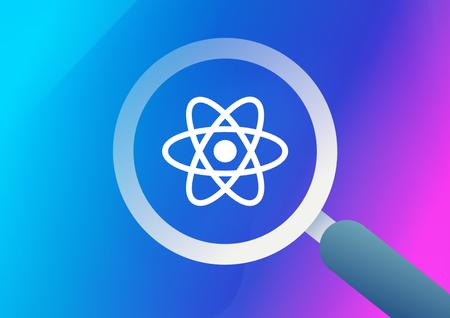
English | MP4 | AVC 1920×1080 | AAC 44KHz 2ch | 84 Lessons (6h 48m) | 1.40 GB
A comprehensive A-Z guide to testing React apps with React Testing Library and Vitest / Jest.
Clear. Concise. Comprehensive.
Tired of piecing together disconnected tutorials or dealing with rambling, confusing instructors? This course is for you! It’s perfectly structured into a series of bite-sized, easy-to-follow videos that cover both theory and practice.
What You’ll Learn
- Master the fundamentals of testing React applications with React Testing Library.
- Write maintainable, robust, and trustworthy tests that consistently deliver value.
- Efficiently mock API responses with Mock Service Worker (MSW).
- Mock data using @mswjs/data and @faker-js.
- Simulate user events in a test environment.
- Dive into advanced testing techniques involving authentication, state management, routing, etc.
- Master refactoring techniques that pros use to make their code more readable and maintainable.
- Learn from real-world examples and exercises that prepare you for the job.
- Use ESLint to catch code quality issues early.
Table of Contents
1 Introduction
2 Prerequisites
3 Course Structure
4 How to Take this Course
5 Setting Up the Development Environment
6 Setting Up the Starter Project
7 Setting Up Vitest
8 Setting Up React Testing Library
9 Introduction
10 What to Test
11 Testing Rendering
12 Simplifying Test Setup
13 Exercise- Testing UserAccount
14 Testing Lists
15 xercise- Testing ProductImageGallery
16 Testing User Interactions
17 Exercise- Testing ExpandableText
18 Simplifying Tests
19 Exercise- Testing SearchBox
20 Testing Asynchronous Code
21 Exercise- Testing ToastDemo
22 Working with Component Libraries
23 Exercise- Simplifying Code
24 Exercise- Testing OrderStatusSelector
25 Is Unit Testing Worth It
26 Catching Common Issues with ESLint
27 Introduction
28 Setting Up Mock Service Worker
29 Testing Data Fetching
30 Exercise- Testing Data Fetching
31 Generating Fake Data
32 Mocking Data
33 Exercise- Mocking Data
34 Testing Errors
35 Exercise- Testing Errors
36 Testing the Loading State
37 Exercise- Testing the Loading State
38 Refactoring- Using React Query
39 Wrapping Components for Testing
40 Exercise- Using React Query
41 Exercise- Testing BrowseProductsPage
42 Exercise- Testing Loading Skeletons
43 Exercise- Testing Error Handling
44 Exercise- Testing Data Rendering
45 Exercise- Refactoring Tests
46 Exercise- Testing Filtering
47 Exercise- Refactoring Tests
48 Code Coverage
49 Exercise- Refactoring with React Query
50 Exercise- Extracting CategorySelect
51 Exercise- Extracting ProductTable
52 Introduction
53 What to Test
54 Testing Rendering
55 Exercise- Testing Initial Data
56 Exercise- Refactoring Tests
57 Exercise- Testing Focus
58 Testing Validation Rules
59 Parameterizing Tests
60 Exercise- Testing Validation Rules
61 Exercise- Extracting a Function for Filling Forms
62 Resolving the Act Warning
63 Exercise- Testing Form Submission
64 Testing Form Feedback
65 Introduction
66 What to Test
67 Exercise- Testing Components that Use Context
68 Exercise- Testing Quantity Selector
69 Exercise- Extracting Utility Function
70 Exercise- Testing Components that Use Redux
71 Exercise- Replacing Redux with React Query
72 Introduction
73 What to Test
74 Mocking the Authentication Status
75 Exercise- Testing AuthStatus
76 Introduction
77 What to Test
78 Testing Routes
79 Extracting navigateTo
80 Exercise- Testing Routes with Parameters
81 Exercise- Testing Invalid Routes
82 Testing Protected Routes
83 Exercise- Testing ProductDetailPage
84 Course Wrap Up
Resolve the captcha to access the links!