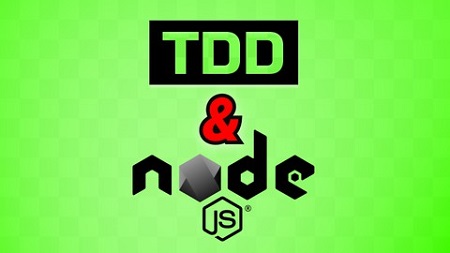
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 22 Hours | 10.6 GB
Build a fully functional backend rest application with express.js by testing nodejs with jest
In this course we will be building a fully functional backend service with express js. we will call our application as hoaxify.
while building this application, we will learn
- how we can build a restful web service with express js, with all necessary functionalities like validation, internationalization, static
- resource serving, caching, json manipulation, interacting with external services
- how we can handle database operations with orm package, sequelize.
- how we can manage database version history and migrations
and we will see
- how test driven development works.
- how it’s affecting our code quality, reusability
- how it’s giving us the confidence about refactoring our implementation
- how we can deploy the application to heroku
- how we can create an instance in google cloud and deploy our application to it
- and how we can automate the deployment with github actions.
This course is purely built on practice. Each code piece we write, will be for our actual application implementation.
In each section we will gradually build our application. We will not jump ahead and add functionality not needed for that moment. We will implement one requirement at a time. Each implementation will bring the next requirement to us.
And following this practice, will help you to get a solid foundation about overall rest web services requirements and how to implement one of them with node js by following test driven development methodology.
What you’ll learn
- Test Driven Development
- Express js
- Node js
- Jest
- Sequelize
- RestFul API
Table of Contents
Introduction
1 Introduction
2 Tools
3 Methodology
4 Creating Project
5 Development Environment Dependencies
6 Web Client
7 Project Source Code
First Rest Endpoint Sign Up
8 First Test Sign Up Request
9 Saving User to Database
10 Password Hashing
11 Refactor
Configuration and Environment
12 Configuration and Environment
Validation
13 Validation
14 Validatior Middleware
15 Express Validator
16 Dynamic Tests
17 Remaining Validations
18 Custom Validation Unique Email Validation
Internationalization – i18n
19 Internationalization – i18n
Account Activation
20 Account Activation Intro
21 Activation Email
22 Email Transport Failures
23 Refactoring Tests
24 Activating Account
25 Testing on Web Client
Error Handler
26 Error Handler
27 Error Body
Loading Users
28 User Page Response
29 Page Content
30 Total Page Info
31 Changing Page
32 Changing Page Size
33 Get User
Authentication – Part 1
34 Authentication
35 Authentication Failure
36 Refactoring Tests
User Update – Part 1
37 Update User – Authentication Failure
38 Update User Success
39 Authorization Aware User List
Authentication – Part 2
40 JWT Token Generation
41 Authorization
42 JWT Practices
43 Opaque Token
44 Logout
45 Delete User
46 Token User Relationship
47 Expiring Token
48 Token Cleanup
Account Recovery
49 Password Reset Request
50 Password Reset Success Response
51 Sending Password Reset Email
52 Refactoring Rest Endpoint
53 Updating Password – Error Cases
54 Updating Password – Success
55 Testing on Client
User Update – Part 2
56 User Profile Image
57 Image in Responses
58 Upload Folder
59 Storing Images in Folder
60 Serving Static Resources
61 Cleaning up the Folders
62 Replacing Old Image
63 Username Validation
64 File Size Validation
65 File Type Validation
66 Testing on Client
Production Preparation
67 Migration Demo
68 Creating Latest Migration Scripts
69 Running Tests Against Shared Database
70 Refactoring Configuration
71 Using PostgreSQL
72 Organizing NPM Scripts
73 Logger
74 Logging App Version
Deployment
75 Production Configuration
76 Deploying to Heroku
77 Preparing VM on Google Cloud
78 Deploying App to VM
79 Automating Deployment
Hoax
80 Submitting Hoax
81 Saving Hoax to Database
82 Validation
83 Hoax Migration Scripts
84 Hoax User Relationship
85 Listing Hoaxes
86 Listing Hoaxes of a User
87 Running Tests with PostgreSQL
88 Deployment
Hoax Attachment
89 Attachment Folder
90 Storing Attachment info in DB
91 Storing Attached File
92 Serving Attachment Folder
93 Storing File Type
94 File Size Limit
95 Attachment Hoax Relationship
96 Attachment in Hoax Listing
97 Migration Scripts
98 Scheduled Service for Unused Attachments
Clean Up
99 Hoax Delete – Error Cases
100 Hoax Delete – Success Cases
101 Hoax Delete – Cascade
102 Migrating Constraint
103 User Delete
Final
104 Updating Dependencies
105 Deployment
Resolve the captcha to access the links!