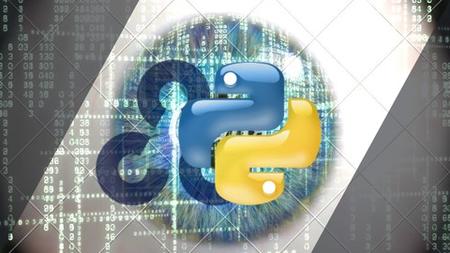
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 115 lectures (8h 58m) | 7.89 GB
Get started with Computer Vision and become a real-time processing Wizard with OpenCV & Python with fully working games
This course will start your Computer Vision journey. You will learn how a computer extracts high-level understanding of what happens in a video. This will all be done by combining theory directly with hands on projects to speed up your learning curve.
Computer Vision is one of most interesting areas in computer science. For obvious reasons:
- How can a computer understand what happens in an image or a video?
- It is simple for you and I to understand what happens in an image or a video
- …but it is not trivial for computers to gain that understanding
At the end of this course you will create two interactive Computer Vision games that extract high level understanding from a real-time webcam flow. All this will be achieved with no prior Computer Vision knowledge. We learn and built along the way. Combining Computer Vision theory immediately by implementing it in useful scenarios.
This is a entertaining way to learn Computer Vision with practical projects at each stage in your learning journey.
Most Computer Vision courses focus on covering a broad basis, with the cost of given a overload of information, which the student will not fully master. This course focuses on learning what is needed to make full interactive games, and it will cover the theory when needed to keep the student engaged and applying the concepts immediately. This will ensure the best learning experience.
When you master something in depth, it will be easier to expand your basis to make more complex projects later. This is the best way to learn a new area. To make fully working projects based on a full understanding of the underlying theory. This is what this course gives you.
Why learn Computer Vision with OpenCV and Python?
- If you want to use the strongest Computer Vision library supported by broad set of languages and most platforms
- OpenCV is a Computer Vision library and is highly optimized with focus on real-time applications.
- OpenCV integrates with C++, Python and Java interfaces on Linux, MacOS, Windows, iOS, and Android
- Python combines the power of being easy to learn and leaves the heavy processing in libraries (like OpenCV)
The best learning practices applied in this course
New concepts need to be applied immediately after you learn them, otherwise you will forget them
You need to understand why you need new concepts in order to be engaged in the learning process
This course has short learning cycles with motivated concepts that are immediately applied in projects
…finally, if you want to build something entertaining, then you are highly motivated
How will you benefit from this course?
- You will master Computer Vision approaches for real-time video applications.
- Have full projects with OpenCV in Python using your webcam
- Master real-time processing of a video stream with OpenCV and Python
- Practical programming experience on how Computer Vision extracts high level understanding of a live webcam stream
- How to extract moving parts from a frame
If you want to become a comfortable with Computer Vision you need to have some basic understanding of the underlying concepts. This course will teach you the main principles in real-time Computer Vision and you will create two interactive games with your webcam stream.
In this course we will cover all concepts for real-time application, like noise tolerant motion detection, inserting objects, interact with objects from webcam to the frames, and combining that to interactive games.
This course covers the following.
- Update or install the newest Python and PyCharm (one of the best environment to develop Python code in).
- Install OpenCV and ensure you have correct version running.
- Understand how webcam can be configured and the limitations.
- Measure Frames-per-seconds and understand the process flow from webcam to screen.
- Understand how Python interacts with OpenCV and keeps processing speed high.
- Learn how frames are represented in Numpy and how they are processed.
- Basic Numpy understandning for OpenCV needs.
- Modifying frames: resize, gray scale, Gaussian blur.
- Working with region of interest (ROI) and inserting objects in frames
- How motion detection works.
- Implementing a simple and noise tolerant motion detection.
- Optimizing processing for noise tolerant motion detection.
- Creating games where you interact through the webcam.
The course is structured in an easy understandable way
- Starting with the simple webcam processing flow with OpenCV and Python
- Adding concepts and processing as we go along with each example having visual explanation and coding examples
- Structure the code to easily expand the concepts and make more advanced processing
- Adding pieces together in a simple way – focus on keeping things understandable
You code along – you only learn by trying yourself – 40 coding lectures
- At each step you make the implementation along with me.
- You implement it in all stages to increase your understanding of Computer Vision with OpenCV and Python.
- Basically, we learn along the way with 40 coding lectures that adds further knowledge at each step.
What is needed to fully understand this course?
- You have basic understand of Python (see prerequisite for full requirements).
- An idea of the Object Oriented Programming concept – only needed in the end and is not high level.
Who is this course for?
- This course is for you, if you want to learn and get started with Computer Vision in a fun way.
- If you like to learn concepts and theory while making projects.
- Those who want to learn the depth of each lesson by programming examples to fully understand it.
What you’ll learn
- Start your Computer Vision journey with OpenCV using Python
- Master real-time video processing with webcam streams
- Create multiple interactive games with your webcam
- Know how to create a motion detection from scratch – also how to make it noise tolerant
- Learn how to resize, gray scale, threshold, Gaussian blur, dilate, and more in a live webcam stream
- How you insert image objects in live webcam stream
- Understand hardware limitations of the webcam
- Identify moving objects and adding boxes around them in a live webcam stream
- Experience using circular buffer for efficient processing
- How to use object oriented programming to have multiple objects moving around in a live webcam stream
Table of Contents
Introduction
1 Overview What will we cover in this course
2 You Learning Process in this course
3 Python skills needed to start this course
4 About me and my experience
Project Create Your First Game
5 Introduction to project Create your first game
6 Code together Finalize the game
7 You code Finalize the game
8 Code together Fine tuning the game
9 You code Fine tuning the game
10 Additional content A different approach – get the background first
11 Additional content You code A different approach – might work better
12 Code together Refactor your code
13 You code Refactor your code
14 Code together Insert an object
15 You code Insert an object
16 Code together Make the object fall from the sky
17 You code Falling objects
18 Code together Crete a score and update it
19 You code Create a score and update it
Project A More Advanced Game
20 Code together Starting a new game
21 You code Adding another tracker and finalize the game
22 You code Starting a new game
23 Code together Refactoring the code
24 You code Refactoring the code
25 Code together Adding a goal to score point
26 You code Adding a goal to score point
27 Code together Adding a tracker to hunt you down
28 You code Adding a tracker to hunt you down
29 Code together Adding another tracker and finalize the game
Python and PyCham (Installation on Mac and Windows)
30 Install Python on Mac (Ensure you have newest version)
31 Install PyCharm on Mac (This is where you do the coding)
32 Create the project in PyCharm on Mac
33 Install Python on Windows (Ensure you have newest version)
34 Install PyCharm on Windows (this is where you do the coding)
35 Create the project in PyCharm on Windows
OpenCV – Let’s get started
36 What is OpenCV and is Python the correct language to use
37 The simple flow from webcam to your monitor using OpenCV
38 Install OpenCV in PyCharm Environment
39 You code You install OpenCV
40 Code together The simple webcam flow
41 You code The simple webcam flow
42 Code together Flip and resize the frame
43 You code Flip and resize the frame
Understand Frames-per-Second and how the webcam limits it
44 Introduction to Frames-per-second
45 Code together Frames per second
46 You code Frames-per-second
How to configure your webcam with OpenCV and the limitations
47 Introduction to Webcam as a hardware
48 Code together Getting and setting Frames-per-second on Webcam
49 You code Getting and setting Frames-per-second
50 Code together Get and set Width and Height on webcam
51 You code Get and set Width and Height on webcam
52 Code together Find all supported webcam resolutions on your webcam
53 You code Find all supported webcam resolutions
Webcam Flow of Processing and NumPy
54 Webcam flow Preprocessing and processing
55 Gray scale
56 Code together Gray scale
57 You code Gray scale
58 Code together The webcam flow structure
59 You code The webcam flow structure
60 What is a Frame and how is it represented
61 Code together How a frame is represented
62 You code How a frame is represented
63 What is NumPy
64 Code together NumPy examples of Frames
65 You code NumPy examples of Frames
First project Insert logo in live webcam stream
66 Introduction to project
67 You code Threshold and masks
68 ROI (Region of Interest) explained
69 Code together ROI
70 You code ROI
71 Code together Putting it all together
72 You code Putting it all together
73 Lossless and Lossy data compression
74 Code together Lossy data compression
75 You code Lossy data compression
76 Gray scale images
77 Code together Gray scale images
78 You code Gray scale images
79 Threshold and masks
80 Code together Threshold and masks
Project Motion Detection from live Webcam stream
81 Introduction to Project Motion Detection
82 You code Using absolute differences
83 Using Gaussian blur (remove more noise)
84 Code together Using Gaussian Blur
85 You code Using Gaussian Blur
86 Moving differences
87 Code together Moving differences
88 You code Moving differences
89 Dilate (make more visible)
90 Code together Dilate
91 You code Dilate
92 Process frames in gray scale
93 Contours (boxes around moving objects)
94 Code together Inserting boxes around moving objects – finalize motion detection
95 You code inserting boxes around moving parts
96 Code together Flip frame, resize frame and make gray scale
97 You code Flip frame, resize frame and make gray scale
98 Using Threshold
99 Code together Using threshold
100 You code Using threshold
101 Using absolute differences Solve the white noise problem (most of it)
102 Code together Using absolute differences
Motion Detection Noise Tolerant
103 Introduction to Noise Tolerant Motion Detection
104 Code together Calculate average efficiently
105 You code Calculate the average efficiently
106 Additional content Background Subtraction in OpenCV
107 Additional content The code if you like to play around with it.
108 Code together Resize the process line
109 You code Resize the process line
110 Circular buffer
111 Code together Refactor code to use a class
112 You code Refactor code to use a class
113 Code together Use deque as Circular Buffer
114 You code Use deque as Circular Buffer
115 Calculate average efficient
Resolve the captcha to access the links!