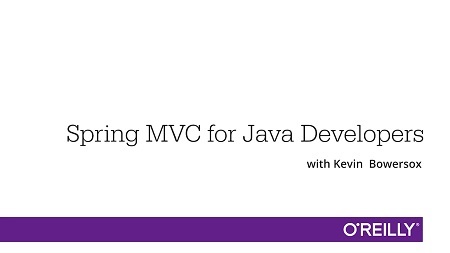
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 9h 14m | 2.92 GB
This screencast is designed to teach novice-level Java developers how to efficiently build well structured Java web applications with the Spring model view controller (MVC) module. The MVC module of the Spring framework provides a simple and lightweight web framework that allows developers to address common web application concerns using an established approach. Students will learn how to configure an advanced set of framework components to build predictable and highly maintainable applications that benefit from being constructed using this well documented technology. Participants should be familiar with the basics of the Java programming language and the concepts underlying the Spring Framework.
- Learn how to work with Spring’s support for web services
- Gain experience working with the Reactive Stack
- Understand how to perform request handling with controllers
- Learn to facilitate web service development with Spring web services
- Understand how to leverage reactive streams
Table of Contents
1 Welcome to the Course
2 About the Author
3 Spring MVC Introduction
4 Model View Controller Overview
5 Model View Controller Benefits
6 Spring MVC Features
7 JDK Installation
8 Spring Tool Suite Installation
9 Spring MVC Demonstration
10 Spring MVC Java Configuration
11 Configuration History
12 Project Setup
13 Logging Configuration
14 DispatcherServlet Registration with Java
15 Expedited Dispatcher Configuration
16 Context Heirarchies
17 View Resolution Configuration
18 Boot Configuration
19 Boot Basics
20 Configuration Overrides
21 Thymeleaf Template Engine
22 WAR Packaging
23 Request Processing Overview
24 Course Project Walkthrough
25 Handling Requests with Controllers
26 Establishing Request Mappings
27 Building Models
28 Autowiring Controller Services
29 Variables in Paths
30 Working with Flexible Handler Arguments
31 Binding Request Data
32 Spring MVC Tags Overview
33 URL Tag
34 Form Tag
35 Input Tag
36 Select Tag
37 Checkboxes and Radio Buttons
38 Textarea Tag
39 Advanced Controllers Overview
40 Databinding Composite Objects
41 Databinding Lists
42 Working with ModelAttributes
43 Working with SessionAttributes
44 SessionStatus
45 @ResponseBody
46 @RequestBody
47 Validation and Exception Handling Chapter Overview
48 Validators Part 1
49 Validators Part 2
50 Bean Validation
51 Form Errors
52 @ExceptionHandler
53 HandlerExceptionResolver
54 Validation and Exception Handling Chapter Overview
55 Chaining View Resolvers
56 Content Negotiation
57 Redirects
58 RedirectAttributes
59 FlashAttributes
60 Advanced Components Chapter Overview
61 Handler Interceptors
62 Bean Scopes
63 JSON Support
64 Controller Advice
65 Databinding With Converters
66 Databinding Arguments with Converters
67 File Upload Support
68 Security Features
69 Postman Installation
70 Global CORS Configuration
71 Granular CORS Configuration
72 CSRF Protection
73 Spring MVC Testing
74 Test Case Configuration
75 Introducing the TestRestTemplate
76 Posts with TestRestTemplate
77 Context Only Testing
78 Fluent API
79 Limited Context Testing
80 Async and Streaming Chapter Overview
81 Async with Deferred Results
82 Async with Callables
83 Streaming with ResponseBodyEmitter
84 Streaming with Server Sent Events
85 Spring Web Services Chapter Overview
86 Building an XSD
87 Class Generation from XSD
88 Service Configuration
89 Endpoints
90 Consumer Configuration
91 WebServiceTemplate
92 Reactive Apps with WebFlux
93 Reactive Concepts
94 Spring WebFlux Overview
95 Project Reactor Basics
96 Mono
97 Flux
98 RouterFunction
99 HandlerFunction
100 Course Wrap Up
Resolve the captcha to access the links!