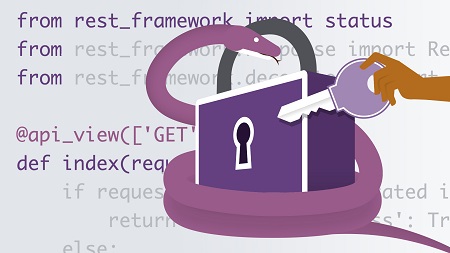
English | MP4 | AVC 1280×720 | AAC 48KHz 2ch | 1h 31m | 236 MB
Learn how to develop more secure Python applications. In this course, instructor Ronnie Sheer reviews the most common vulnerabilities in Python apps and explains how to set up a coding environment that helps you develop code with security in mind. Learn how to avoid common pitfalls associated with loose typing and assertions and find out how to deserialize Pickle data. Then explore the security features—such as code generation and secrets management—in Django, a popular Python framework. Ronnie also explains how to secure a RESTful API in Django using permissions, data serialization, and automated testing, and closes the course with some tips for securing applications written with Flask, a powerful micro web framework.
Topics include:
- Secure coding standards
- Setting up a secure Python coding environment
- Dynamic typing with Python
- Securing endpoints
- Securing Django
- Securing RESTful APIs
- Testing Python apps
- Securing Flask
Table of Contents
1 Developing securely
2 What you should know
3 What are secure coding, CERT, and other standards
4 What is OWASP Top 10
5 Installing software with due caution
6 Installing pipenv, Python, Django, Flask, and Django REST framework
7 Common vulnerabilities and exposures checks
8 A few words about encryption and injection
9 Dynamic typing with Python
10 Explicit assertions with Python
11 Don’t get yourself into a Pickle
12 Challenge Secure the end point
13 Solution Secure the end point
14 Using a separate Python environment for isolation
15 The batteries included approach in Django
16 Generating new projects
17 The Django settings module, keeping secrets, and the dangers of debug mode
18 Safe serializing
19 Permissions
20 Testing and security
21 Challenge Run the test, fix the code
22 Solution Run the test, fix the code
23 The challenge of securing Flask
24 Flask secrets
25 Password hashing with Flask
26 Next steps Secure coding
Resolve the captcha to access the links!