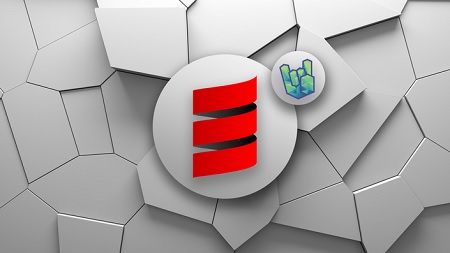
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 42 Lessons (13h 48m) | 1.47 GB
Become a Scala programmer. Dive head-first and hands-on into the fundamentals of Scala and functional programming.
Think differently.
If you’re reading this, you probably know already: Scala is one of the most powerful languages on the JVM and is behind some of the best libraries and frameworks for big data processing (Spark), distributed & fault-tolerant computing (Akka), streaming (Kafka, Akka Streams), microservices (Lagom), web apps (Play) and many others.
Scala has seen huge demand in recent years, has some of the best-paid engineering positions, and is just plain fun. I know you can’t verify the fun for yourself right now, but I personally hardly know anyone who has tasted Scala and then came back to Java out of their own free will. I also have a stadium of 41000 students who took this course and say the same thing.
After this course, you’ll be able to:
- understand Scala in production environments and in any project
- expand your existing object-oriented concepts to a new mindset with immutable values
- think code differently with functional programming
- write powerful code very quickly: 1-2 lines of Scala code enough for 50 lines of Java
- contribute to products and open-source projects written in Scala
- write applications in Scala from scratch
- quickly learn any Scala framework and library: Spark, Akka, Play, Lagom etc
Skills you’ll get:
- Lasting comfort with the Scala language
- think code differently with values and expressions
- use blocks of code as expressions
- write compact code with recursion
- master the 2 recursion techniques
- use the 3 string interpolation techniques in different scenarios
- use the compiler’s type inference effectively
- Extended object-oriented concepts
- immutable values
- the 3 method notations for expressive code
- singleton patterns in one line of code
- inheritance in Scala
- abstract classes and traits
- anonymous classes
- generics (which are generally poorly understood)
- exception handling
- Functional programming
- using functions as values
- anonymous functions (lambdas)
- the underscore notation and alternative syntax
- higher-order functions
- collections
- map, flatMap, filter
- Scala-only features
- pattern matching
- variance (introduction)
- for-comprehensions
all with live runnable examples and practiced with exercises.
Table of Contents
1 Welcome
2 Values and Types
3 Expressions
4 Functions
5 Type Inference
6 Recursion
7 Call by Name and Call by Value
8 Default and Named Arguments
9 String Operations and Interpolations
10 Object-Oriented Basics
11 Object-Oriented Basics: Exercises
12 Method Notations
13 Inheritance
14 Access Modifiers
15 Preventing Inheritance
16 Scala Objects
17 Abstract Classes and Traits
18 Inheritance Exercises and Starting Our Own List
19 Introduction to Generics
20 Anonymous Classes
21 Exercise: Extending MyList
22 Case Classes
23 Enums
24 Handling Exceptions
25 Imports and Exports
26 What’s a Function, Really?
27 Anonymous Functions
28 Higher-Order Functions and Currying
29 HOFs & Currying: Exercises
30 HOFs & Currying: Exercises, part 2
31 Map, flatMap, filter and for-comprehensions
32 Linear Collections: Seq, List, Array, Vector, Set, Range
33 Tuples and Maps
34 Tuples & Maps: Exercises
35 Handling Absence: Option
36 Handling Failure: Try
37 Pattern Matching
38 ALL the Patterns!
39 Patterns Everywhere
40 Hot Takes: Braceless Syntax
41 Hot Takes: Imperative Programming
42 You Rock!
Resolve the captcha to access the links!