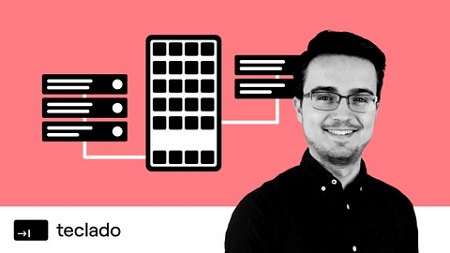
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 122 lectures (11h 59m) | 2.05 GB
Build professional REST APIs with Python, Flask, Docker, Flask-Smorest, and Flask-SQLAlchemy
Are you tired of boring, outdated, incomplete, or incorrect tutorials? I say no more to copy-pasting code that you don’t understand.
Welcome to the bestselling REST API course on Udemy! I’m Jose. I’m a software engineer, here to help you truly understand and develop your skills in web and REST API development with Python, Flask, and Docker.
Production-ready REST APIs with Flask
This course will guide you in creating simple, intermediate, and advanced REST APIs including authentication, deployments, databases, and much more.
We’ll start with a Python refresher that will take you from the very basics to some of the most advanced features of Python—that’s all the Python you need to complete the course.
Using Flask and popular extensions Flask-Smorest, Flask-JWT-Extended, and Flask-SQLAlchemy we will dive right into developing complete, solid, production-ready REST APIs.
We will also look into essential technologies like Git and database migrations with Alembic.
You’ll be able to…
- Create resource-based, production-ready REST APIs using Python, Flask, and popular Flask extensions;
- Handle secure user registration and authentication with Flask.
- Using SQLAlchemy and Flask-SQLAlchemy to easily and efficiently store resources to a database; and
- Understand the complex intricacies of deployments of Flask REST APIs.
- Use Docker to simplify running and deploying your REST APIs.
But what is a REST API anyway?
A REST API is an application that accepts data from clients and returns data back. For example, a REST API could accept text data from the client, such as a username and password, and return whether that is a valid user in the database.
When developing REST APIs, our clients are usually web apps or mobile apps. That’s in contrast to when we make websites, where the clients are usually the users themselves.
Together we’ll develop a REST API that not only allows clients to authenticate but also to store and retrieve any data you want from a database. Learning this will help you develop any REST API that you need for your own projects!
What you’ll learn
- Connect web or mobile applications to databases and servers via REST APIs
- Create secure and reliable REST APIs which include authentication, deployments, and database migrations
- Understand the different layers of a web server and how web applications interact with each other
- Handle seamless user authentication with advanced features like token refresh
- Handle log-outs and prevent abuse in your REST APIs with JWT blacklisting
- Develop professional-grade REST APIs with expert instruction
Table of Contents
Welcome!
1 How to take this course
2 Python on Windows
3 Python on Mac
A Full Python Refresher
4 Introduction to this section
5 Access the code for this section here
6 Variables in Python
7 Solution to coding exercise Variables
8 String formatting in Python
9 Getting user input
10 Writing our first Python app
11 Lists, tuples, and sets
12 Advanced set operations
13 Solution to coding exercise Lists, tuples, sets
14 Booleans in Python
15 If statements
16 The ‘in’ keyword in Python
17 If statements with the ‘in’ keyword
18 Loops in Python
19 Solution to coding exercise Flow control
20 List comprehensions in Python
21 Dictionaries
22 Destructuring variables
23 Functions in Python
24 Function arguments and parameters
25 Default parameter values
26 Functions returning values
27 Solution to coding exercise Functions
28 Lambda functions in Python
29 Dictionary comprehensions
30 Solution to coding exercise Dictionaries
31 Unpacking arguments
32 Unpacking keyword arguments
33 Object-Oriented Programming in Python
34 Magic methods __str__ and __repr__
35 Solution to coding exercise Classes and objects
36 @classmethod and @staticmethod
37 Solution to coding exercise @classmethod and @staticmethod
38 Class inheritance
39 Class composition
40 Type hinting in Python 3.5+
41 Imports in Python
42 Relative imports in Python
43 Errors in Python
44 Custom error classes
45 First-class functions
46 Simple decorators in Python
47 The ‘at’ syntax for decorators
48 Decorating functions with parameters
49 Decorators with parameters
50 Mutability in Python
51 Mutable default parameters (and why they’re a bad idea)
Your first REST API
52 Access the course e-book here
53 Overview of the project we’ll build
54 Initial set-up for a Flask app
55 Your first REST API endpoint
56 What is JSON
57 How to interact with and test your REST API
58 How to create stores in our REST API
59 How to create items in each store
60 How to get a specific store and its items
Introduction to Docker
61 What are Docker containers and images
62 How to run a Flask app in a Docker container
63 In-depth Docker tutorial notes
Flask-Smorest for more efficient development
64 Data model improvements for our API
65 General improvements to our first REST API
66 New endpoints for our first REST API
67 How to run the API in Docker with automatic reloading and debug mode
68 How to use Blueprints and MethodViews in Flask
69 How to write marshmallow schemas for our API
70 How to perform data validation with marshmallow
71 Decorating responses with Flask-Smorest
Store data in a SQL database with SQLAlchemy
72 Overview and why use SQLAlchemy
73 How to code a simple SQLAlchemy model
74 How to write one-to-many relationships using SQLAlchemy
75 How to configure Flask-SQLAlchemy with your Flask app
76 How to insert data into a table using SQLAlchemy
77 How to find models in the database by ID or return a 404
78 How to update models with SQLAlchemy
79 How to retrieve list of all models
80 How to delete models with SQLAlchemy
81 Conclusion of this section
Many-to-many relationships with SQLAlchemy
82 Changes in this section
83 One-to-many relationship between stores and tags
84 Many-to-many relationship between items and tags
User authentication with Flask-JWT-Extended
85 Changes in this section
86 What is a JWT
87 Who uses the JWT
88 How to set up Flask-JWT-Extended with our app
89 Coding the User model and schema
90 How to add a register endpoint to the REST API
91 How to add a login endpoint to the REST API
92 Protect endpoints by requiring a JWT
93 JWT claims and authorization
94 How to add logout to the REST API
95 Request chaining with Insomnia
96 Token refreshing with Flask-JWT-Extended
Database migrations with Alembic and Flask-Migrate
97 Why use database migrations at all
98 How to add Flask-Migrate to our Flask app
99 Initialize your database with Flask-Migrate
100 Change SQLAlchemy models and generate a migration
101 Manually review and modify database migrations
Git Crash Course
102 What are Git repositories and commits
103 Initialize a Git repository for our project
104 Writing Markdown for documents and commits
105 Remote repositories and how to use them
106 Git branches and merging
107 Merge conflicts and how to resolve them
108 Overview of the final e-book chapters
Deployments with Render.com
109 Overview of this section
110 Creating a Render.com web service
111 How to run Flask with gunicorn in Docker
112 Get a deployed PostgreSQL database
113 Use PostgreSQL locally and in production
114 Test the finished production app
Task Queues with rq and sending emails
115 How to send emails with Python and Mailgun
116 How to send emails when users register
117 What is a task queue and setting up a Redis database
118 How to Populate and consume the task queue with rq
119 How to process background tasks with the rq worker
120 How to send HTML emails using Mailgun and Python
121 How to deploy a background worker to render.com
Bonus Section
122 Bonus lecture other courses and next steps
Resolve the captcha to access the links!