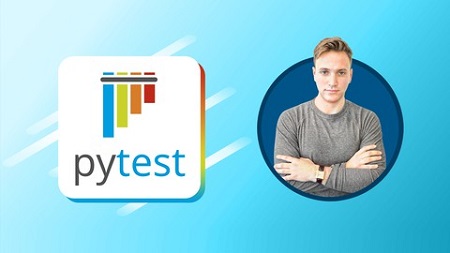
English | MP4 | AVC 1280×720 | AAC 48KHz 2ch | 7 Hours | 4.91 GB
Learn Pytest by building a full Django application with a Continuous Integration system, software testing best practices
This is a MUST course for anyone who cares about testing.
I teach the ins and outs of pytest while building a real world django application (including a continuous integration system in bitbucket).
In this course we will build a very simple django server, I will teach just enough django so that we will be able to build the application, and then focus on testing it.
We will together test the application from all angels – Unit tests, integration tests,API tests, end to end tests ,performance tests with (a total of over 40 tests)
You will end up with a complete CI system that integrates bitbucket cloud pipelines, slack messaging and allure reporting.
Every time we will make a push, the CI system will run our tests and will notify us if the build passed/failed
In this course we go in depth and we will even implement together (from scratch) some of pytest’s features so that we will know what happening under the hood when pytest is running our tests.
This is not an entry-level course, basic knowledge of python is needed
You will learn:
Pytest features (in depth)
- Fixtures
- Markers
- Parametrize
- Skip, xfail
- Pytest.ini
- Pytest-django
- Pytest-cov
- pytest-xdist
- unittest library, mocks
- Requests library
Django (just enough to build a web server)
- Rest API
- Models, Migrations
- Views
- Serializers
- SQLite3 DB
- Email backends
Continuous Integration (in depth)
- Bitbucket pipelines
- Bitbucket environment variables
- Parallel steps
- Docker
- Slack messaging integration
- Allure Reporting
Testing (In depth)
- Unit tests
- Mocking. Patching, Stubs
- Integration tests
- Performance tests
- Testing environments
Python best practice
- Virtual environments: pipenv
- Pipfile
- Type hinting
- Black formatter
- .env File
What you’ll learn
- Learn the ins and outs of Pytest
- Build a real world Django web server
- Build a continuous integration system
- Testing Best Practices
- Build high-performing, reliable automated test suites
- Allure Reporting
- Slack messaging
- Unit, Integration, E2E, performance tests
- Mocking and Patching
Table of Contents
Introduction Course topics, learning tips
1 Course Topics
2 About the instructor (Corona Virus Edition)
3 How get the best of Udemy
4 Short explanation of course sections
5 Course Goals- What Is In It For You
6 How I suggest watching the course – Watch this Before moving to the next section
7 Course Telegram Channel
Pytest Quick Start Tutorial
8 Pytest Quick Overview
9 The GIST of pytest in 20 minuets – Markers
10 The GIST of pytest in 20 minuets – Fixtures, Parametrize
Unit tests VS Integration tests
11 Unit tests VS Integration tests
Setting Up Your Development Environment
12 Creating a bitbucket project
13 Installing pipenv and required packages
Creating and testing our awesome Django project
14 What are we going to build
15 Django project setup
16 Creating our Django Models
17 Creating our REST API
18 Manually testing our project’s features
Pytest automatic testing for our Django application
19 Writing our first pytests
20 API Test classes (unittest style)
21 Pytest skip and xfail markers
22 Pytest tests that assert Exceptions
23 Pytest tests that assert Logs
24 Pytest test runner
25 Testing Django Applications
26 Refactoring our tests to pytest native
27 Summery Pytest VS unittest
Continuous Integration
28 Intro to Continuous Integration
29 Hands on CI- creating bitbucket pipelines to run our tests
30 Hands on CI- Bitbucket environment variables
Django emailing service
31 Adding an email sending service
32 Testing our email service
33 Refactoring our Tests to Pytest
Exploring Pytest with Fibonacci
34 Intro to fibonacci tests
35 Pytest Parametrize
36 Implementing from scratch Pytests’s parametrize feature
37 Caching
38 Running CI steps in paralell
39 Pytest fixture- Time tracking
40 Using dynamic programming to solve fiobnacci
Performance Testing
41 Implementing a pytest performance validator
42 Why not to use pytest-timeout
Pytest Assert Magic
43 Pytest Assert Magic
Endpoint Assignment
44 Assignment
Pytest-xdist
45 Running our tests on multiple threads with pytest-xdist
Slack CI Integration
46 Setting a slack notification when bitbucket CI pipeline fails passes
Conftest.py files and .env file
47 conftest.py
48 env file (auto reloading environment variables)
Advanced Fixtures
49 Fixtures Theory
50 Fixtures with arguments
51 Executing parametrized fixtures
Server Agnostic API Testing (requests library)
52 Server Agnostic API Testing with requests library (part 1)
53 Server Agnostic API Testing with requests library (part 2)
54 Mocking Requests to Isolate 3rd party dependencies in test functions (Theory)
55 Mocking Requests to Isolate 3rd party dependencies in test functions (Hands On)
Allure Report Tool
56 What is Allure reporting
57 End To end Allure Integration – Test Report Webserver
Cool Plugins For pytest
58 Pytest-sugar
Mocking in Python
59 Python Mocking Theory
60 How to Patch Like a PRO
61 Patch is Hijacking Target’s memory address
Troubleshooting Section
62 What is the troubleshooting section for
63 Configure Pycharm Default Test Runner to Pytest and set global Pytets Env Vars
64 Frequently Asked Import Errors (In depth explanation + resolution)
65 Frequently Asked INSTALLED APPS RuntimeError (explanation + resolution)
66 Clone The Ready to go Django Project (If you are just interested in pytest)
Congratulations – Software Developer Certificate
67 Congratulations – Software Developer Certificate
Resolve the captcha to access the links!