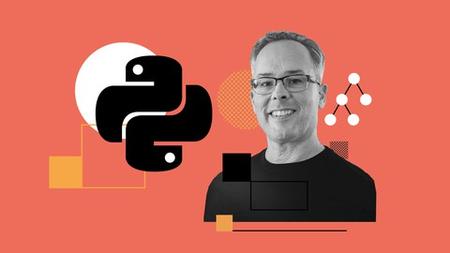
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 110 lectures (8h 4m) | 1.14 GB
DSA for Cracking the Coding Interview. Animated Examples for Faster Learning and Deeper Understanding.
Welcome to Data Structures & Algorithms in Python
This course makes learning to code fun and makes hard concepts easy to understand.
How did I do this? By using animations!
Animating the Data Structures & Algorithms makes everything more visually engaging and allows students to learn more material – in less time – with higher retention (a pretty good combination).
I will use these visuals to guide you, step-by-step, through the entire course.
The course also includes dozens-and-dozens of Coding Exercises. So you can immediately put everything into practice as soon as you learn it (a very important step).
All of this will help you to feel more confident and prepared when you walk into a coding interview.
What you will get in this course…
Here are the topics we will cover:
Technical
- Big O notation
Data Structures
- Lists
- Linked Lists
- Doubly Linked Lists
- Stacks & Queues
- Binary Trees
- Hash Tables
- Graphs
Algorithms
- Sorting
- Bubble Sort
- Selection Sort
- Insertion Sort
- Merge Sort
- Quick Sort
Searching
- Breadth First Search
- Depth First Search
What you’ll learn
- 90 Coding Exercises with Detailed Explanations
- Enhance Your Learning with Animated Examples of DSA
- Gain Expertise in Data Structures and Algorithms
- Ace Technical Interviews with Confidence
- Understand Time and Space Complexity of Data Structures and Algorithms
- Elevate Your Development Skills to the Next Level
- Boost Your Problem-Solving Ability
Table of Contents
Start Here
1 Overview (Please Watch)
2 Code Editor
Big O
3 Big O Intro
4 Big O Worst Case
5 Big O O(n)
6 Big O Drop Constants
7 Big O O(n^2)
8 Big O Drop Non-Dominants
9 Big O O(1)
10 Big O O(log n)
11 Big O Different Terms for Inputs
12 Big O Lists
13 Big O Wrap Up
Classes & Pointers
14 Classes
15 Pointers
Linked Lists
16 Linked List Intro
17 LL Big O
18 LL Under the Hood
19 LL Constructor
20 Coding Exercises (Important)
21 LL Print List
22 LL Append
23 LL Pop Intro
24 LL Pop Code
25 LL Prepend
26 LL Pop First
27 LL Get
28 LL Set
29 LL Insert
30 LL Remove
31 LL Reverse
LL Coding Exercises
LL Interview Leetcode Exercises
Doubly Linked Lists
32 DLL Constructor
33 DLL Append
34 DLL Pop
35 DLL Prepend
36 DLL Pop First
37 DLL Get
38 DLL Set
39 DLL Insert
40 DLL Remove
DLL Coding Exercises
DLL Interview Leetcode Exercises
Stacks & Queues
41 Stack Intro
42 Stack Constructor
43 Stack Push
44 Stack Pop
45 Queue Intro
46 Queue Constructor
47 Queue Enqueue
48 Queue Dequeue
S & Q Coding Exercises
S & Q Interview Leetcode Exercises
Trees
49 Trees Intro & Terminology
50 Binary Search Trees Example
51 BST Big O
52 BST Constructor
53 BST Insert – Intro
54 BST Insert – Code
55 BST Contains
BST Coding Exercises
Hash Tables
56 Hash Table Intro
57 HT Collisions
58 HT Constructor
59 HT Set
60 HT Get
61 HT Keys
62 HT Big O
63 HT Interview Question
HT Coding Exercises
HT Interview Leetcode Exercises
64 Introduction to Sets
Graphs
65 Graph Intro
66 Graph Adjacency Matrix
67 Graph Adjacency List
68 Graph Big O
69 Graph Add Vertex
70 Graph Add Edge
71 Graph Remove Edge
72 Graph Remove Vertex
Graph Coding Exercises
Recursion
73 Recursion Intro
74 Call Stack
75 Factorial
Recursive Binary Search Trees
76 rBST Contains
77 rBST Insert
78 rBST Delete Intro
79 rBST Delete Code (1 of 3)
80 rBST Delete Code (2 of 3)
81 rBST Minimum Value
82 rBST Delete Code (3 of 3)
rBST Coding Exercises
Basic Sorts
83 Bubble Sort Intro
84 Bubble Sort Code
85 Selection Sort Intro
86 Selection Sort Code
87 Insertion Sort Intro
88 Insertion Sort Code
89 Insertion Sort Big O
Basic Sorts Coding Exercises
Basic Sorts Interview Leetcode Exercises
Merge Sort
90 Merge Sort Overview
91 Merge Intro
92 Merge Code
93 Merge Sort Intro
94 Merge Sort Code
95 Merge Sort Big O
Merge Sort Coding Exercises
Merge Interview Leetcode Exercise
Quick Sort
96 Quick Sort Intro
97 Pivot Intro
98 Pivot Code
99 Quick Sort Code
100 Quick Sort Big O
Quick Sort Coding Exercises
Tree Traversal
101 Tree Traversal Intro
102 BFS (Breadth First Search) Intro
103 BFS Code
104 DFS (Depth First Search) PreOrder – Intro
105 DFS PreOrder – Code
106 DFS PostOrder – Intro
107 DFS PostOrder – Code
108 DFS InOrder – Intro
109 DFS InOrder – Code
BST Traversal Coding Exercises
BST Traversal Interview Leetcode Exercises
Other Interview Leetcode Exercises
Coding Exercises
110 CODING EXERCISES HAVE MOVED!
Resolve the captcha to access the links!