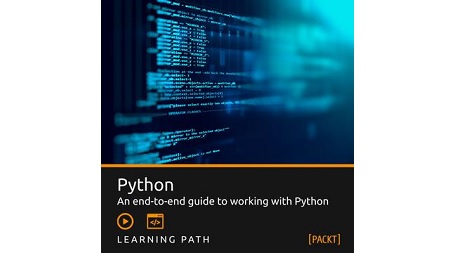
English | 2016 | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 7h 15m | 1.34 GB
Learn about Python from start to finish
Rising in popularity alongside data science, it’s easy to forget that Python has a wide range of applications outside of crunching numbers and outputting graphs. This Learning Path will take you through a tour of the language from the fundamentals right up to more advanced patterns that help make your code more expressive, efficient and readable.
This path navigates across the following products (in sequential order):
- Beginning Python (4h 20m)
- Mastering Python (2h 35m)
Table of Contents
Beginning Python
1.The Course Overview and Installing Python
2.Setting Up a Programming Environment
3.Variables
4.Introduction to Types
5.Basic Operators
6.Introduction to Strings
7.String Functions
8.Advanced String Manipulation
9.String Formatting
10.User Input
11.Introduction to Lists
12.List Methods
13.Advanced List Methods
14.Built-in List Functions
15.2D Arrays and Array References
16.List Slicing
17.MySQL and Basic SQL Syntax
18.Comparison Operators
19.Else and Elif
20.and, or, and not
21.Conditional Examples
22.Mini Program
23.For Loop
24.While Loop
25.Iterables
26.Loops and Conditionals
27.Prime Number Checker
28.Function Basics
29.Parameters and Arguments
30.Return versus Void Functions
31.Working with Examples
32.Advanced Examples
33.Recursion
34.Recursion Examples
35.Import, as, and from
36.Python API and Modules
37.Creating Modules
38.Modules and Testing
39.Installing PIL-Pillow
40.Basics of Using PIL-Pillow
41.Picture Manipulations
42.Custom Picture Manipulation
43.Wrapping Up
Mastering Python
44.The Course Overview
45.Downloading and Installing Python
46.Using the Command Line and the Interactive Shell
47.Installing Packages with pip
48.Finding Packages in the Python Package Index
49.Creating an Empty Package
50.Adding Modules to the Package
51.Importing One of the Package-s Modules from Another
52.Adding Data Files to the Package
53.PEP 8 and Writing Readable Code
54.Using Version Control
55.Using venv to Create a Stable and Isolated Work Area
56.Getting the Most Out of docstrings Part 1 – PEP 257 and Sphinx
57.Getting the Most Out of docstrings Part 2 – doctest
58.Making a Package Executable via python – m
59.Handling Command-line Arguments with argparse
60.Text-mode Interactivity
61.Executing Other Programs
62.Using Shell Scripts or Batch Files to Launch Programs
63.Using concurrent.futures
64.Using Multiprocessing
65.Understanding Why Asynchronous I-O Isn-t Like Parallel Processing
66.Using the asyncio Event Loop and Coroutine Scheduler
67.Futures
68.Making Asynchronous Tasks Interoperate
69.Communicating across the Network
70.Using Function Decorators
71.Using Function Annotations
72.Using Class Decorators
73.Using Metaclasses
74.Using Context Managers
75.Using Descriptors
76.Understanding the Principles of Unit Testing
77.Using unittest
78.Using unittest.mock
79.Using unittest-s Test Discovery
80.Using Nose for Unified Test Discovery and Reporting
Resolve the captcha to access the links!