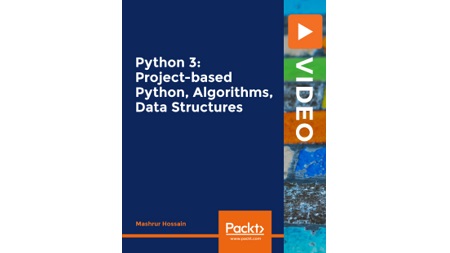
English | MP4 | AVC 1920×1080 | AAC 48KHz 2ch | 14h 26m | 3.90 GB
Learn to program with Python 3, visualize algorithms and data structures, and implement them in Python projects
This course is one of the most comprehensive and beginner-friendly courses on learning to code with Python—one of the top programming languages in the World—and using it to build algorithms and data structures with projects from scratch.
We will walk you step-by-step through the fascinating world of Python programming using visualizations of programs as they execute, algorithms as they run, and data structures as they are constructed. Nothing is left to the imagination; you’ll see it all and then build it all.
Since it caters to a broad spectrum of students, the course is split into two parts: part 1 focusing on the Python programming language and part 2 focusing on Algorithms, data structures, performance analysis, and larger-scale projects.
Part 1: Python and programming fundamentals
- Text – Strings
- Numbers – ints and floats
- Execution flow control – branching with if/elif/else
- Compound data types – lists, dictionaries, tuples, and sets
- Iterables and iteration with generators, for and while loops, and more!
- Functions, execution context and frames, and building custom functions
- List comprehension
- Lambda expressions
- Generators and creating your own generators with yield
- Objects and building classes, methods, and special methods
- Reading from and writing to files using context managers
- Visualization with each topic and more!
Part 2: Algorithms, Data Structures, and Performance Analysis
- Sorting algorithms (basic) – bubble sort, selection sort, and insertion sort
- Sorting algorithms (advanced) – merge sort and quick sort
- Big O notation, complexity analysis, divide and conquer, and math visualizations
- Recursion in-depth with examples
- Searching algorithms – bisection search and hashing
- Data structures with linked lists, stacks, queues, trees, and binary search trees
- Operations with data structures – insert, search, update, and delete
- Multiple projects with increasing levels of complexity to tie concepts together
- Visualizations of all algorithms, data structure, operations, and more!
What You Will Learn
- Learn Python 3 from scratch, in-depth
- Understand the fundamentals of programming languages
- Learn to visualize algorithms, data structures, program executions, and information flows
- Learn to use Python to build projects
Table of Contents
Introduction
1 Introduction
2 Course structure and content overview
Development environment setup
3 Section intro and overview
4 Download and install Python
5 Setup Atom as text editor (setup used in this course)
6 Exploring Jupyter Notebooks interface (optional)
Python in-depth
7 Section intro and overview
8 Command line Terminal basics
9 Strings, variables, top down execution flow
10 Strings – concatenation, indexing, slicing, python console
11 String methods, functions and import statements
12 Print formatting and special characters
13 Numbers, math, type casting and input
14 Introduction to branching (if, elif, else) and conditionals
15 Building if, elif, else blocks incrementally
16 Lists, dicts, sets and tuples – Intro to compound data types in Python
17 Lists – an in-depth look 1
18 Lists – an in-depth look 2
19 Dictionaries, sets and tuples
20 Iterators, for loops, generators, list comprehension
21 While loops, enumerate, zip
22 Functions – an introductory look
23 Functions – implementation step by step
24 Functions – execution context, frames, mutable vs. immutable arguments in-depth
25 Classes and objects – an introductory look
26 Building a custom Student class and intro to special methods
27 Add some methods to the class
28 Special methods and what they are
29 Reading from and writing to files
30 Add read functionality and utilize special and static methods
31 Inheritance, subclasses and complete example class
32 Lambda expressions and map function
33 Generators – under the hood
34 Build your own generators using yield
Algorithms – Sort, performance, complexity and big O notation
35 Introduction to section 4 and overview of the material covered in it
36 Bubble sort demonstration and complexity analysis
37 Bubble sort implementation
38 Selection sort demonstration and complexity analysis
39 Selection sort implementation
40 Insertion sort demonstration and assignment handoff
41 Insertion sort programmatic execution step by step
42 Performance measures – deep dive with a programmatic view
43 O(nlog(n)) performance and algorithm prerequisites
44 Analyze log(n), visualize the math behind it and how it relates to algorithms
45 Merge sort visualization and complexity analysis
46 Implement merge function – part 1
47 Implement merge function – part 2
48 Implement merge function – part 3
49 A look at the recursive divide function
50 In-depth look at execution context of recursive divide function
51 Recursion mini-project 1 – Countdown timer
52 Recursion mini-project 2 – Factorial
53 Recursion mini-project 3 – Fibonacci series
54 Complete merge sort algorithm and analyze updated execution context
55 Quicksort demo
56 Quicksort implementation
57 Section final project objective and motivation
58 Project specs and runtime execution intro
59 Project phase 1 – Build random int list generator
60 Project phase 2 – Get input from user for size and range
61 Project phase 3 – Add functions, calculate and analyze runtime
62 Project phase 4 – Extract redundancies, create function and cleanup code
63 Project phase 5 – Add multiple run functionality and perform additional testing
Algorithms – Search and abstract data structures
64 Introduction to section 5
65 Intro to search – Linear, Bisection Binary search
66 Bisection Binary search – Iterative implementation
67 Bisection search – recursive implementation
68 Project handoff – Bringing it together
69 Project conclusion walkthrough
70 Hashmaps and O(1) search complexity
71 Hash project 1 – Define and set up class blueprint with init and str
72 Hash project 2 – Set up insert and hashing functionality for data structure
73 Hash project 3 – Add update functionality
74 Hash project 4 – Build search method
75 Project – Use hash structure in a practical exercise – Quote finder
76 Project – Complete quote finder using hash table
77 Intro to linear data structures – Linked Lists
78 Build a custom linked list
79 Recursively reverse a linked list
80 Visualize Stacks and Queues, and their operations
81 Introduction to Trees and Binary Search Trees
82 In-order traversal of a Binary Search Tree
83 Build a Binary Search Tree from scratch – Insert
84 BST from scratch – In-order traversal
85 BST from scratch – Search
86 BST from scratch – Delete demo
87 BST – Deleting leaf nodes
88 BST – Deleting nodes with 1 child node
89 BST – Deleting nodes with 2 children
90 Project – Job Scheduler using Binary Search Trees – Introduction
91 Project – Job Scheduler execution flow
92 Project – Job Scheduler implementation tips and notes
93 Thank you for taking the course and next steps
Resolve the captcha to access the links!