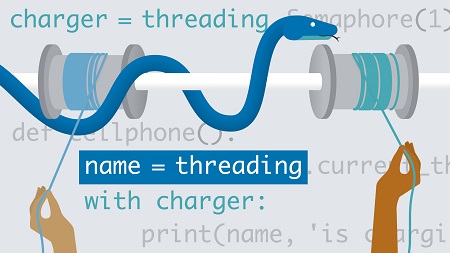
English | MP4 | AVC 1280×720 | AAC 48KHz 2ch | 2h 19m | 581 MB
Parallel programming is key to writing faster and more efficient applications. This course, the second in a series from instructors Barron and Olivia Stone, introduces more advanced techniques for parallel and concurrent programming in Python. Barron and Olivia explain concepts like condition variables, semaphores, barriers, and thread pools in a fun and informative way, relating them to everyday activities you perform in the kitchen. They also explain how to evaluate your code’s performance and design more efficient parallel programs from the start with techniques like partitioning. To cement the ideas, they demo them in action using Python—closing the course with a variety of coding challenges. Each lesson is short and practical, driving home the theory with hands-on techniques.
Topics include:
- Working with condition variables
- Exploring the producer-consumer problem
- Controlling the order of operations with barriers
- Reusing threads with thread pools
- Adding placeholders with futures
- Measuring speedup, latency, and throughput
- Designing parallel programs
- Combining tasks
- Mapping tasks
Table of Contents
Synchronization
1 Condition variable
2 Condition variable Python demo
3 Producer-consumer
4 Producer-consumer threads Python demo
5 Producer-consumer processes Python demo
6 Semaphore
7 Semaphore Python demo
Barriers
8 Race condition
9 Race condition Python demo
10 Barrier
11 Barrier Python demo
Asynchronous Tasks
12 Computational graph
13 Thread pool
14 Thread pool Python demo
15 Process pool Python demo
16 Future
17 Future Python demo
18 Divide and conquer
19 Divide and conquer Python demo
Evaluating Parallel Performance
20 Speedup, latency, and throughput
21 Amdahl’s law
22 Measure speedup
23 Measure speedup Python demo
Designing Parallel Programs
24 Partitioning
25 Communication
26 Agglomeration
27 Mapping
Challenge Problems
28 Challenge Matrix multiply in Python
29 Solution Matrix multiply in Python
30 Challenge Merge sort in Python
31 Solution Merge sort in Python
32 Challenge Download images in Python
33 Solution Download images in Python
Conclusion
34 Additional resources
Resolve the captcha to access the links!