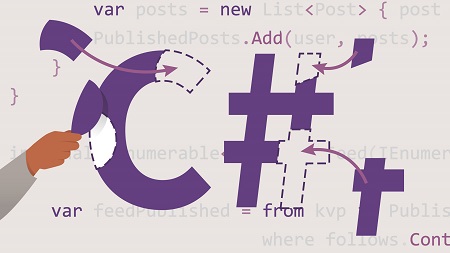
English | MP4 | AVC 1280×720 | AAC 48KHz 2ch | 4h 07m | 621 MB
Learn how to use object-oriented programming (OOP) principles in C# to help simplify some of your more complex work. In this course, Anton Delsink explores the many features of C# that support object-oriented programming, including abstract classes, interfaces, and generic types. To help lend a real-world context to these concepts, Anton demonstrates how to use these features by example as he examines existing code and writes some new code. Throughout the course, he covers a wide range of scenarios—including examples from .NET, Windows UI, the web, and even board games—and discusses whether or not he adhered to OOP principles in different instances.
Topics include:
- Abstract classes
- Processing text with StreamReader
- IEnumerable and yield return
- Windows Forms controls
- Windows Forms with and without the designer
- Adding, organizing, and testing forms
- Creating classes
- Inheritance
Table of Contents
1 The wide world of object-oriented programming (OOP) with C#
2 What you should know
3 Theory vs. practice
4 When a square is not a rectangle
5 When a carpet is art
6 Conclusion
7 Introduction
8 IEnumerable
9 IEnumerable and foreach
10 File.Open and .Close
11 File.Close in the finally block
12 Keyword using
13 Opening FileStream with StreamReader
14 Processing text with StreamReader
15 IEnumerable and yield return
16 TextReader as base of StreamReader and StringReader
17 Conclusion
18 Introduction
19 Windows Forms controls
20 Windows Forms without the designer
21 Application.Run and .Exit
22 Inheriting from class Form
23 Windows Forms with the designer
24 Event handler for control
25 Windows Forms MVC
26 Adding and organizing forms
27 Create and test a second form
28 Windows Presentation Foundation (WPF) XAML
29 WPF button and event handler
30 Conclusion
31 Introduction
32 Chess game model and controller
33 Chess game events
34 Chess game view
35 Apply chess moves
36 Update chess model
37 Add chess pieces
38 Summary of Chess MVC
39 Board game ChessMoves
40 Create class ChessMove
41 Add members of class ChessMove
42 Implement method ChessMove.Try
43 Invoke ChessMove.Try
44 Summary of ChessMoves
45 Introduction
46 Create classes User, Post, and Content
47 Write test for use case 1
48 Implement Content.Publish (use case 1)
49 Implement SocialGraph.Follow (use case 2)
50 Write test for use case 3
51 Implement SocialGraph.GetFollows
52 Implement Content.GetFeed
53 Implement Content.Like and .Share
54 Update .GetFeed for likes and shares
55 Summary
56 Next steps
Resolve the captcha to access the links!