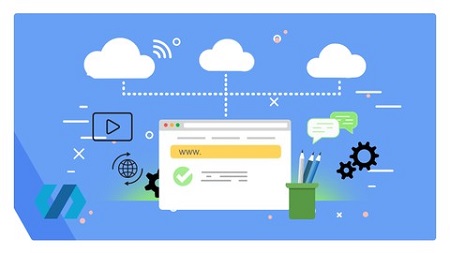
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 483 lectures (51h 55m) | 13.1 GB
The most up-to-date JS resource online! Master Javascript by building a beautiful portfolio of projects!
This course is divided into two parts. The first half of the course focuses on teaching you the basic syntax of Javascript. Colt will walk you through core topics effortlessly, imparting jewels of JS wisdom along the way. Included in the first half of the course are many programming exercises and small projects, so you can test your new-found knowledge out. Each of these videos can be easily referenced in the future, so you can always come back and brush up on some topic whenever needed.
The second half of the course is focused on building some amazing projects. Stephen will show you how to build some production-ready Javascript applications, including a fully-featured E-Commerce web app! These projects are all styled to be absolutely beautiful, visually stunning apps that you will be proud to feature on your own personal portfolio. The main goal of these projects is to highlight design patterns, and show you the ‘right’ and ‘wrong’ ways of writing code. By the end, you’ll be confident enough to work on your own personal projects with speed and finesse.
What You’ll Learn
This is a long course, with just about every fact about Javascript you could ever hope to know. Here’s a brief subset of the topics you’ll cover:
- Master the basics of the language, easily understanding variables, objects, arrays, and functions
- Understand how to design the structure of the code you write, leading to beautiful and easy-to-read programs
- Leverage Javascript’s built-in methods to increase your productivity regardless of what libraries or frameworks you use
- Develop practical skills around higher-order functions that you will utilize for years to come
- Observe how the Javascript and browser work together, and how to increase the performance of JS code
- Build awesome projects to fill your personal portfolio
- Build command line tools from scratch using Node JS
- Fetch and manage information from third-party API’s
- Build a fully-featured E-Commerce application from scratch – including production-grade authentication!
This is the ultimate Javascript course. There are many resources online for learning Javascript, but this is the only one that covers everything you need to know, from ‘A’ to ‘Z’, and a couple letters after that. Master the basics with Colt, then build awesome projects with Stephen. We’ve taught a million other engineers how to code, and now it is your turn!
Table of Contents
Introduction
1 How This Course Works
2 JS, ECMA, TC39 What Do They Mean
3 The Tools You Need
4 Customizing VSCode & Extensions
5 A Quick Note About MDN
JS Values & Variables
6 Goals & Primitives
7 Running Code in the JS Console
8 Introducing Numbers
9 NaN & Infinity
10 Numbers Quiz
11 Variables & Let
12 Unary Operators
13 Introducing Const
14 The Legacy of Var
15 Variables Quiz
How to Model Data Efficiently
16 Booleans Intro
17 Strings
18 String Indices
19 String Methods
20 More String Methods
21 Strings Quiz
22 String Escape Characters
23 String Template Literals
24 Null & Undefined
25 The Math Object & Random Numbers
26 typeof operator
27 parseInt & parseFloat
Controlling Program Logic and Flow
28 Making Decisions in JS
29 Comparison Operators
30 Double Equals (==)
31 Triple Equals (===)
32 Running Code From a Script
33 If Statements
34 Else If
35 Else
36 Nesting Conditionals
37 Truthy & Falsy Values
38 Logical AND (&&)
39 Logical OR ()
40 NOT Operator (!)
41 Operator Precedence
42 The Switch Statement
43 Ternary Operator
Capture Collections of Data with Arrays
44 Creating Arrays
45 Array Indices
46 Modifying Arrays
47 Push and Pop
48 Shift and Unshift
49 Concat
50 Includes and IndexOf
51 Reverse and Join
52 Slice
53 Splice
54 Sorting (Part 1)
55 Intro to Reference Types
56 Using Const with Arrays
57 Working with Nested Arrays
Objects – The Core of Javascript
58 Intro to Objects
59 Creating Object Literals
60 Accessing Object Properties
61 Adding and Updating Properties
62 Nested Arrays & Objects
63 Objects and Reference Types
64 ArrayObject Equality
The World of Loops
65 Intro to Loops
66 For Loops
67 Infinite Loops!
68 For Loops & Arrays
69 Nested For Loops
70 Intro to While Loops
71 More While Loops
72 Break Keyword
73 For…Of Intro
74 Comparing For and For…Of
75 For…Of with Objects
76 For…In Loops
Writing Reusable Code with Functions
77 Our First Function!
78 Dice Roll Function
79 Introducing Arguments
80 Functions With Multiple Args
81 The Return Statement
82 More on Return Values
83 Function Challenge 1 passwordValidator
84 Function Challenge 2 Average
85 Function Challenge 3 Pangrams
86 Function Challenge 4 Get Playing Card
An Advanced Look at Functions
87 Function Scope
88 Block Scope
89 Lexical Scope
90 Function Expressions
91 Higher Order Functions
92 Functions as Arguments
93 Functions as Return Values
94 Callbacks
95 Hoisting
Apply Functions to Collections of Data
96 Intro to Array Callback Methods
97 forEach
98 Map
99 Arrow Functions Intro
100 Arrow Functions Implicit Returns
101 Array.find
102 Filter
103 Some & Every
104 Revisiting Sort!
105 Reduce Intro
106 Reduce Pt. 2
107 Even More Reduce!
A Few Miscellaneous JS Features
108 New JS Features Intro
109 Default Parameters
110 Spread for Function Calls
111 Spread in Array Literals
112 Spread in Object Literals
113 The Arguments Object (not new)
114 Rest Parameters (new!)
115 Destructuring Arrays
116 Destructuring Objects
117 Nested Destructuring
118 Destructuring Parameters
Object Methods and the ‘This’ Keyword
119 Shorthand Object Properties
120 Computed Properties
121 Adding Methods to Objects
122 Method Shorthand Syntax
123 Intro to Keyword THIS
124 Using THIS in Methods
125 THIS Invocation Context
126 Annoyomatic Demo
127 Putting It All Together Deck Of Cards
128 Creating A Deck Factory
JS In the Browser – DOM Manipulation
129 Introduction to the DOM
130 IMPORTANT NOTE HTML & CSS
131 Taste of the DOM
132 Another Fun DOM Example
133 The Document Object
134 getElementById
135 getElementsByTagName
136 getElementsByClassName
137 querySelector & querySelectorAll
Twisting the DOM to Our Will!
138 Working with innerText & textContent
139 innerHTML
140 value, src, href, and more
141 Getting & Setting Attributes
142 Finding ParentChildrenSiblings
143 Changing Multiple Elements
144 Altering Styles
145 getComputedStyle
146 Manipulating Classes
147 Creating Elements
148 Append, Prepend, & insertBefore
149 removeChild & remove
150 NBA Scores Chart Pt1
151 NBA Scores Chart Refactor
Communicating with Events
152 Intro to DOM Events
153 Ways NOT to Add Events
154 addEventListener
155 The Impossible Button Demo
156 Events on Multiple Elements
157 The Event Object
158 Key Events keypress, keyup, & keydown
159 Coin Game Demo
160 Form Events & PreventDefault
161 Input & Change Events
Asynchronous Code, Callbacks & Promises
162 The Call Stack
163 Call Stack Debugging w Dev Tools
164 JS is Single Threaded
165 How Asynchronous Callbacks Actually Work
166 Welcome to Callback Hell
167 Introducing Promises!
168 Returning Promises from Functions
169 ResolvingRejecting w Values
170 The Delights of Promise Chaining
171 Refactoring w Promises
Making HTTP Requests
172 Intro to AJAX
173 JSON & XML
174 XMLHttpRequests The Basics
175 XMLHttpRequests Chaining Requests
176 A Better Way Fetch!
177 Chaining Fetch Requests
178 Refactoring Fetch Chains
179 An Even Better Way Axios
180 Sequential Axios Requests
Async & Await JS Magic
181 A Quick Overview of Async Functions
182 The Async Keyword
183 The Await Keyword
184 Error Handling in Async Functions
185 Multiple Awaits
186 Parallel Vs. Sequential Requests
187 Refactoring with Promise.all
Prototypes, Classes, & The New Operator
188 What on Earth are Prototypes
189 An Intro to OOP
190 Factory Functions
191 Constructor Functions
192 JS Classes – Syntactical Sugar
193 A Bit More Practice with Classes
194 Extends, Super, and Subclasses
Drawing Animations
195 Welcome to Part 2!
196 App Overview
197 Project Setup
198 Event-Based Architecture
199 Class-Based Implementation
200 Binding Events in a Class
201 Reminder on ‘This’
202 Determining the Value of ‘This’
203 Solving the ‘This’ Issue
204 Starting and Pausing the Timer
205 Where to Store Data
206 DOM-Centric Approach
207 Getters and Setters
208 Stopping the Timer
209 Notifying the Outside World
210 OnTick and OnComplete
211 Extracting Timer Code
212 Introducing SVG’s
213 Rules of SVG’s
214 Advanced Circle Properties
215 The Secret to the Animation
216 First Pass on the Animation
217 Smoothing the Animation
218 Adjusting by an Even Interval
219 Using Icons
220 Styling and Wrapup
Application Design Patterns
221 Application Overview
222 Starter Kit Setup
223 Big Challenges
224 Fetching Movie Data
225 Fetching a Single Movie
226 AutoComplete Widget Design
227 Searching the API on Input Change
228 Delaying Search Input
229 Understanding Debounce
230 Implementing a Reusable Debounce
231 Extracting Utility Functions
232 Awaiting Async Functions
233 Rendering Movies
234 Handling Errored Responses
235 Opening a Menu
236 Style of Widget Creation
237 Moving HTML Generation
238 Quick Note
239 Repairing References
240 Handling Broken Images
241 Automatically Closing the Dropdown
242 Handling Empty Responses
243 Handling Movie Selection
244 Making a Followup Request
245 Rendering an Expanded Summary
246 Expanded Statistics
247 Issues with the Codebase
248 Making the Autocomplete Reusable
249 Displaying Multiple Autocompletes
250 Extracting Rendering Logic
251 Extracting Selection Logic
252 Removing Movie References
253 Consuming a Different Source of Data
254 Refreshed HTML Structure
255 Avoiding Duplication of Config
256 Hiding the Tutorial
257 Showing Two Summaries
258 When to Compare
259 How to Compare
260 Extracting Statistic Values
261 Parsing Number of Awards
262 Applying Parsed Properties
263 Updating Styles
264 Small Bug Fix
265 App Wrapup
Javascript with the Canvas API
266 Application Overview
267 Project Setup
268 Matter Terminology
269 Getting Content to Appear
270 Boilerplate Overview
271 Drawing Borders
272 Clicking and Dragging
273 Generating Random Shapes
274 Maze Generation Algorithm
275 More on Maze Generation
276 Configuration Variables
277 Grid Generation
278 Verticals and Horizontals
279 Abstracting Maze Dimensions
280 Guiding Comments
281 Neighbor Coordinates
282 Shuffling Neighbor Pairs
283 Determining Movement Direction
284 Updating Vertical Wall Values
285 Updating Horizontal Wall Values
286 Validating Wall Structure
287 Iterating Over Walls
288 Drawing Horizontal Segments
289 Drawing Vertical Segments
290 Drawing the Goal
291 Drawing the Playing Ball
292 Handling Keypresses
293 Adding Keyboard Controls
294 Disabling Gravity
295 Detecting a Win
296 Adding a Win Animation
297 Stretching the Canvas
298 Understanding the New Unit Variables
299 Refactoring for Rectangular Mazes
300 Adding Fill Colors
301 Displaying a Success Message
Make a Secret-Message Sharing App
302 Application Overview
303 Project Setup
304 Handling Form Submission
305 Base64 Encoding
306 Encoding the Entered String
307 Parts of a URL
308 Generating the URL
309 Toggling Element Visibility
310 Decoding the Message
311 Displaying the Message
312 App Deployment
Create Node JS Command Line Tools
313 JavaScript with Node vs the Browser
314 Executing JavaScript
315 Working with Modules
316 Invisible Node Functions
317 The Require Cache
318 Files Get Required Once!
319 Debugging with Node
320 App Overview
321 Accessing Standard Library Modules
322 The Callback Pattern in Node
323 The Process.cwd Function
324 Running a Node Program as an Executable
325 Linking a Project
326 Is it a File or a Folder
327 A Buggy Initial Implementation
328 Optional Solution #1
329 A Callback-Based Solution
330 Callback-Based Functions Using Promises
331 Issues with Sequential Reads
332 Promise.all-Based Solution
333 Console Logs with Colors
334 Accepting Command Line Arguments
335 Joining Paths
336 App Wrapup
Create Your Own Project Runner
337 App Overview
338 Project Setup
339 Big Application Challenges
340 Watching Files with Chokidar
341 Issues with Add Event
342 Debouncing Add Events
343 Naming Require Statements
344 Handling CLI Tools with Caporal
345 Ensuring Files Exist
346 It Works!
347 More on StdIO
348 [Optional] More on Child Process
349 App Wrapup
Project Start – E-Commerce App
350 App Overview
351 App Architecture
352 Package.json Scripts
353 Creating a Web Server
354 Behind the Scenes of Requests
355 Displaying Simple HTML
356 Understanding Form Submissions
357 Parsing Form Data
358 Middlewares in Express
359 Globally Applying Middleware
Design a Custom Database
360 Data Storage
361 Different Data Modeling Approaches
362 Implementing the Users Repository
363 Opening the Repo Data File
364 Small Refactor
365 Saving Records
366 Better JSON Formatting
367 Random ID Generation
368 Finding By Id
369 Deleting Records
370 Updating Records
371 Adding Filtering Logic
372 Exporting an Instance
373 Signup Validation Logic
Production-Grade Authentication
374 Cookie Based Authentication
375 Creating User Records
376 Fetching a Session
377 Signing Out a User
378 Signing In
379 Hashing Passwords
380 Salting Passwords
381 Salting + Hashing Passwords
382 Comparing Hashed Passwords
383 Testing the Full Flow
Structuring Javascript Projects
384 Project Structure
385 Structure Refactor
386 HTML Templating Functions
387 HTML Reuse with Layouts
388 Building a Layout File
389 Adding Better Form Validation
390 Validation vs Sanitization
391 Receiving Validation Output
392 Adding Custom Validators
393 Extracting Validation Chains
394 Displaying Error Messages
395 Validation Around Sign In
396 Password Validation
397 Template Helper Functions
398 Adding Some Styling
399 Exposing Public Directories
400 Next Steps
401 Product Routes
402 The Products Repository
403 Code Reuse with Classes
404 Creating the Products Repository
405 Building the Product Creation Form
406 Some Quick Validation
Image and File Upload
407 Exploring Image Upload
408 Understanding Mutli-Part Forms
409 Accessing the Uploaded File
410 [Optional] Different Methods of Image Storage
411 Saving the Image
412 A Subtle Middleware Bug
413 Better Styling
414 Reusable Error Handling Middleware
415 Products Listing
416 Redirect on Success Actions
417 Requiring Authentication
418 Template Update
419 Ids in URLs
420 Receiving URL Params
421 Displaying an Edit Form
Building a Shopping Cart
422 Editing a Product
423 Fixing the HandleErrors Middleware
424 Edit Form Template
425 Deleting Products
426 Starting with Seed Data
427 User-Facing Products
428 Products Index
429 Merging More Styling
430 Understanding a Shopping Cart
431 Solving Problem #1
432 Solving Problem #2
433 Shopping Cart Boilerplate
434 Submission Options
435 Creating a Cart, One Way or Another
436 Adding Items to a Cart
437 Displaying Cart Items
438 Rendering the List
439 Totaling Cart Items
440 Removing Cart Items
441 Redirect on Remove
The Basics of Testing
442 Testing Overview
443 A Simple Function to Test
444 A No-Frills Testing Implementation
445 Test Driven Development
446 Fixing Three Issues
447 The Assert Module
448 Using Mocha
449 App Setup
450 Reminder on This App
451 Why is Test Setup Difficult
452 Mocha in the Browser
453 Displaying the Autocomplete
454 Verifying the Dropdown State
455 Writing Assertions
456 Fake DOM Events
457 Holding Up Assertions
458 Implementing WaitFor
459 Asserting Records Fetched
Building a Testing Framework From Scratch
460 Test Framework Requirements
461 Project Setup
462 Implementation Steps
463 Walking a Directory Structure
464 Implementing Breadth First Search
465 Collecting Test Files
466 Running Test Files
467 A Quick Test Harness
468 Implementing ‘beforeEach’ and ‘it’
469 Adding Basic Reporting
470 Adding Colors
471 Better Formatting
472 Ignoring Directories
473 Running Browser-Based JS
474 A Sample Web App
475 Why JSDOM
476 Building a Render Function
477 HTML Element Assertions
478 An Incorrectly Passing Test
479 Another Small Issue
480 Script Execution Delay
481 Implementing a Delay
482 Fixing a Test
Bonus!
483 Bonus!
Resolve the captcha to access the links!