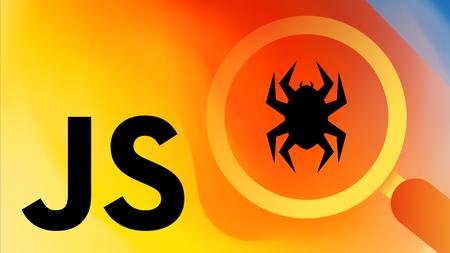
English | MP4 | AVC 1920×1080 | AAC 44KHz 2ch | 55 Lessons (3h 51m) | 687 MB
A comprehensive, beginner-friendly guide covering everything from the basics to advanced techniques
Tired of piecing together disconnected tutorials or dealing with rambling, confusing instructors? This course is for you! It’s perfectly structured into a series of bite-sized, easy-to-follow videos that cover both theory and practice.
What You’ll Learn
- Explore the fundamentals of unit testing and its benefits.
- Master core unit testing techniques and best practices.
- Craft maintainable, robust, and trustworthy tests that deliver value.
- Learn to execute and navigate tests effectively in VSCode.
- Perform positive, negative, and boundary testing for comprehensive coverage.
- Evaluate code coverage to ensure comprehensive testing.
- Create parameterized tests for versatile testing scenarios.
- Isolate code with mocks and learn when to use them.
- Spy on functions to gain insights into their behavior.
- Improve code quality using static analysis tools.
- Utilize Prettier to format your code consistently.
- Implement ESLint to catch code quality issues early.
- Prevent type errors in your code using TypeScript.
- Automate code quality checks using Husky.
Table of Contents
1 Welcome
2 Prerequisites
3 Course Structure
4 How to Take This Course
5 Setting Up the Testing Environment
6 Starter Project
7 Introduction
8 What is Unit Testing
9 Types of Tests
10 Choosing a Testing Framework
11 Setting Up Vitest
12 Writing Your First Tests
13 Exercise Testing fizzBuzz
14 Test-driven Development
15 Exercise Testing Factorial
16 Running Tests
17 Navigating Tests in VSCode
18 Code Coverage
19 Introduction – Code with Mosh
20 Characteristics of Good Unit Tests
21 Using Matchers
22 Writing Good Assertions
23 Exercise Testing getCoupons
24 Positive and Negative Testing
25 Exercise Testing validateUserInput
26 Boundary Testing
27 Exercise Testing isValidUsername
28 Exercise Testing canDrive
29 Parameterized Tests
30 Exercise Parameterized Tests
31 Testing Asynchronous Code
32 Setup and Teardown
33 Exercise Testing a Stack
34 Introduction
35 Creating Mock Functions
36 Exercise Working with Mock Functions
37 Mocking Modules
38 Exercise Testing getShippingInfo
39 Interaction Testing
40 Exercise Testing submitOrder
41 Partial Mocking
42 Spying on Functions
43 Clearing, Resetting, and Restoring Mocks
44 To Mock or Not to Mock
45 Mocking Dates
46 Exercise Testing getDiscount
47 Introduction
48 What are Static Analysis Tools
49 Formatting Code with Prettier
50 Linting Code with ESLint
51 Catching Type Errors with TypeScript
52 Running ESLint on TypeScript
53 Automating Code Quality Checks with Husky
54 Running Tests on Push
55 Course Wrap Up
Resolve the captcha to access the links!