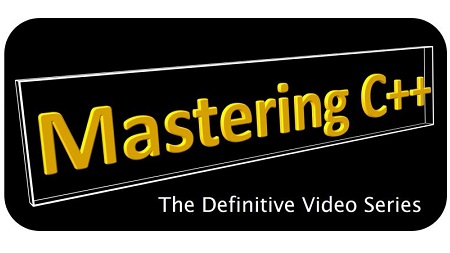
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 8h 22m | 1.19 GB
Go beyond the basics in this C++ video series, which guides you through intermediate and advanced functionality spanning these four categories:
- Frequently used features including Lambda Expressions, Move Semantics, RAII, and Smart Pointers.
- Templates, Generic Programming, and Metaprogramming.
- STL Library including STL Containers and STL Algorithms.
- Concurrency including Threads, Mutex, and Condition Variables.
This series assumes you know the basics of C++. (If you do not yet know the basics, watch this excellent video on C++ Fundamentals) There are 14 video clips in this video series:
- Lambda Expressions Part 1. This first clip in the C++ video series introduces lambda expressions and function objects. Lambda naming is discussed in detail, and lambda expressions are contrasted with functions, function objects, and for loops, and you will learn the use cases for each of these valuable tools.
- Lambda Expressions Part 2. This second clip in the C++ video series covers the technical details of lambda. Learn about the components of the lambda expression, capture lists, parameter lists, return times, and optional mutable specifiers. We discuss the lifetime of the lambda expression and its impact on the correctness and behavior of the program.
- Move Semantics Part 1. This third clip in the C++ video series covers move semantics in detail. Learn all about containers and how move semantics differ from copy semantics. We explore the constructor and assignment implementations. We explain how move semantics are invoked, and when move semantics should be used over copy semantics.
- Move Semantics Part 2. This fourth clip in the C++ video series covers rvalue reference in detail. We explain lvalue, rvalue, lvalue, reference, and rvalue reference. We explain the significance of rvalue reference in invoking move semantics, and show how rvalue reference becomes an lvalue. With this knowledge at hand, we complete the case of moving objects that contain object members.
- RAII and Smart Pointers. This fifth clip in the C++ video series covers the Resource Acquisition Is Initialization (RAII) mechanism of resource management. Problems with mixing resource management and program logic are highlighted along with RAII-based solutions to such problems. Learn where pointer semantics are necessary and when smart pointers should be used. We show how unique_ptr and shared_ptr can provide the basic facilitation of RAII without separating resource management from program logic.
- Templates and Generic Programming Part 1. This sixth clip in the C++ video series explains generic programming and how it differs from type-specific code and meta programming. We explain the role of templates in generic programming. We highlight the two outcomes of generic programming which are templates classes and algorithms. We then program an abstract generic class from a type-specific partial vector implementation to show how we can create templates classes of our own.
- Templates and Generic Programming Part 2. This seventh clip in the C++ video series expands on the topic of generic programming. Learn the process of lifting by programming an algorithm from two specific implementations of the sum (accumulate) function. We explain variadic templates, processing of variadic templates, and the advantages of using variadic templates. We explore Printf and explain when to use variadic templates.
- Constexpr. This eighth clip in the C++ video series explains how constexpr is used for meta programming to perform compile time computations. We discuss what operations are allowed in constexpr and explain the constexpr function and what can and cannot be part of a constexpr function. We conclude with an explanation of the if-constexpr.
- Metaprogramming Part 1. This ninth clip in the C++ video series explains metaprogramming and why it is so important. The two main components of type functions and control structures are explained. We explain the usage of type functions and type predicates and show how type safe runtime code can be generated using these features. We also show how we can write type functions for use in metaprogramming.
- Metaprogramming Part 2. This tenth clip in the C++ video series focuses on the control structures of metaprogramming. Learn about selection, recursion, and conditional (enable-if) compilation in detail using practical examples. We show how these structures can be used to select type-specific functions at compile time. The examples also show how we can implement type parameter requirements through metaprogramming.
- STL Containers. This eleventh clip in the C++ video series explains the design philosophy of the STL library. Learn about the role of container, stack, algorithm, and iterator components. Categories of containers are discussed along with significant containers. We show how containers can be copied and how data can be copied or moved from one container to another container with ease.
- STL Algorithms. This twelfth clip in the C++ video series explains the central idea of STL algorithms. Learn how algorithms can perform simple and frequently required tasks in a generic and extremely efficient way. Examples show how algorithms can easily operate on containers using iterators without actually knowing the type of container. We conclude with an explanation and usage of execution policies that allow us to run parallel versions of STL algorithms.
- Threads. This thirteenth clip in the C++ video series explains the usage of the thread class. The difference between thread objects and system level execution threads are revealed, along with sharing the usage and significance of join and detach calls. Learn about the issues with executing a thread destructor on a running thread and issues with an improper usage of join and detach. Heisenbugs and detach are covered as well.
- Mutex and Condition Variables. This fourteenth clip in the C++ video series explores mutex (mutual exclusion variable) classes (MUTEX, recursive_mutax, timed_mutex, and recursive_timed_mutex) and the functionality of mutex in achieving mutual exclusion with reference to the access of resources. Mutex lock and unlock operations are explained in detail. Performance degradation caused by the improper use of mutex is highlighted. We also explain the issue of deadlock caused by the wrong use of mutex or a group of mutexes. The purposes of lock_guard and unique_lock are explained along with their use. We conclude with a brief explanation of condition variables and how they differ from mutexes.
Table of Contents
01 Lambda Expressions Part 1
02 Lambda Expressions Part 2
03 Move Semantics Part 1
04 Move Semantics Part 2
05 RAII and Smart Pointers
06 Templates and Generic Programming Part 1
07 Templates and Generic Programming Part 2
08 Constexpr
09 Metaprogramming Part 1
10 Metaprogramming Part 2
11 STL Containers
12 STL Algorithms
13 Threads
14 Mutex and Condition Variables
Resolve the captcha to access the links!