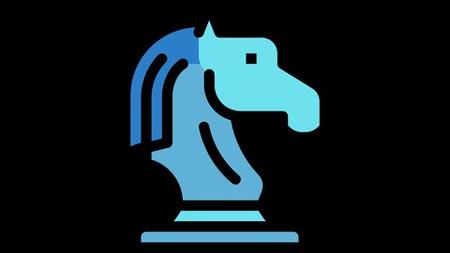
English | MP4 | AVC 1920×1080 | AAC 44KHz 2ch | 249 lectures (36h 11m) | 18.31 GB
Master Java framework Spring 6, AOP, Spring MVC, Spring Boot 3, Thymeleaf, Spring Security 6, Spring JDBC, JPA,REST
‘Master Spring framework, Spring Boot, REST, JPA, Hibernate’ course will help in understanding about Spring framework and how to build web applications, Rest Services using Spring, Spring MVC, SpringBoot, Thymeleaf, Spring JDBC, Spring Data JPA etc. By the end of this course, students will understand all the below topics,
- What is Spring framework ?
- Spring Vs Java EE
- Evolution of Spring and release timeline of Spring
- Different projects inside Spring
- Spring Core Concepts like Inversion of Control (IoC), Dependency Injection (DI) & Aspect-Oriented
- Programming (AOP)
- Different approaches of Beans creation inside Spring framework
- Bean Scopes inside Spring framework
- Autowiring of the Spring Beans
- Lombok library and Annotations
- Introduction to MVC pattern & overview of web apps
- Spring MVC internal architecture & how to create web applications using Spring MVC & Thymeleaf
- Spring MVC Validations
- How to build dynamic web apps using Thymeleaf & Spring
- Thymeleaf integration with Spring, Spring MVC, Spring Security
- Deep dive on Spring Boot, Auto-configuration
- Spring Boot Dev Tools
- Spring Boot H2 Database
- Securing web applications using Spring Security
- Authentication , Authorization, Role based access
- Cross-Site Request Forgery (CSRF) & Cross-Origin Resource Sharing (CORS)
- Database create, read, update, delete operations using Spring JDBC
- Introduction to ORM frameworks & database create, read, update, delete operations using Spring Data
- JPA/Hibernate
- Derived Query methods in JPA
- OneToOne, OneToMany, ManyToOne, ManyToMany mappings inside JPA/Hibernate
- Sorting, Pagination, JPQL inside Spring Data JPA
- Building Rest Services inside Spring
- Consuming Rest Services using OpenFeign, Web Client, RestTemplate
- Spring Data Rest & HAL Explorer
- Logging inside Spring applications
- Properties Configuration inside Spring applications
- Profiles inside Spring Boot applications
- Conditional Bean creation using Profiles
- Monitoring Spring Boot applications using SpringBoot Actuator & Spring Boot Admin
- Deploying SpringBoot App into cloud using AWS Elastic Beanstalk
Table of Contents
Introduction to Spring Framework
1 Introduction to the course Agenda of the course
2 Details of Source Code PDF Content other instructions for the course
3 What is Spring
4 Jakarta EE Vs Spring
5 Introduction to Spring Core
6 Introduction to Inversion of Control IoC Dependency Injection DI
7 Demo of Inversion of Control IoC Dependency Injection DI
8 Advantages of Inversion of Control Dependency Injection
9 Introductions to Beans Context and SpEL
10 Introduction to Spring IoC Container
Creating Beans inside Spring Context
11 Installation of Maven
12 Creating Maven Project
13 Creating Beans using Bean annotation
14 Understanding NoUniqueBeanDefinitionException in Spring
15 Providing a custom name to the bean
16 Understanding Primary Annotation inside Spring
17 Creating Beans using Component annotation
18 Stereotype Annotations in Spring
19 Comparison between Bean Vs Component
20 Understanding PostConstruct Annotation
21 Understanding PreDestroy Annotation
22 Creating Beans programmatically using registerBean
23 Creating Beans using XML Configurations
24 Why should we use frameworks
25 Introduction to Spring Projects Part 1
26 Introduction to Spring Projects Part 2
Wiring Beans using Autowiring
27 Introduction to wiring autowiring inside Spring
28 Wiring Beans using method call
29 Wiring Beans using method parameters
30 Wiring Beans using Autowired on class fields
31 Wiring Beans using Autowired on setter method
32 Wiring Beans using Autowired on constructor
33 Deep dive of Autowiring inside Spring Theory
34 Deep dive of Autowiring inside Spring Coding example
35 Understanding and Avoiding Circular dependencies
36 Problem Statement for Assignment related to Beans Autowiring and DI
37 Solution for Assignment related to Beans Autowiring and DI
Beans scope inside Spring framework
38 Introduction to Bean Scopes inside Spring
39 Deepdive on Singleton Bean scope
40 What is a Race Condition
41 Usecases of Singleton Bean scope
42 Deepdive of Eager and Lazy instantiation of Singleton scope
43 Demo of Eager and Lazy instantiation of Singleton bean
44 Eager Initialization Vs Lazy Initialization
45 Deepdive of Prototype Bean scope
46 Singleton Beans Vs Prototype Beans
Aspect Oriented Programming AOP inside Spring framework
47 Introduction to Aspect Oriented Programming AOP
48 Understanding the problems inside web applications with out AOP
49 Understanding Running the Application with out AOP
50 AOP Jargons
51 Weaving inside AOP
52 Type of Advices inside AOP
53 Configuring Advices inside AOP Theory
54 Configuring Around advice
55 Configuring Before advice
56 Configuring AfterThrowing and AfterReturning advices
57 Configuring Advices inside AOP with Annotations approach
58 Demo of Configuring Advices inside AOP with Annotations approach
Building Web Applications using SpringBoot and Spring MVC
59 Quick Introduction about Web Applications
60 Role of Servlets inside Web Applications
61 Evolution of Web Apps inside Java ecosystem
62 Types of Web Apps we can build with Spring
63 Introduction to Spring Boot The Hero of Spring framework
64 Spring Boot Important features
65 Creating simple web application using Spring Boot
66 Running simple web application using Spring Boot
67 Changing the default server port context path of SpringBoot Web application
68 Random server port number inside SpringBoot
69 Demo of SpringBoot Autoconfiguration
70 Quick recap
Adapting Thymeleaf for building dynamic content inside Spring MVC Web Apps
71 Quick Tip Mapping multiple paths inside Spring Web Application
72 Introduction to Thymeleaf
73 Building dynamic content using Thymeleaf
74 Disabling Thymeleaf template caching
75 Introduction to Spring Boot DevTools
76 Implemetation Demo of Spring Boot DevTools
77 Building Home Page of EazySchool Web Application
78 Understanding the Home Page source code of EazySchool
79 Deep Dive of Spring MVC Internal architecture
80 Separation of Header and Footer code using Thymeleaf replace tag
81 Building Courses Web Page of Eazy School Web Application
82 Quick Tip Resolving Build Cache issues inside maven projects
83 Building About Page of Eazy School Web Application
84 Building Contact Page of Eazy School Web Application
85 Submit information from Contact page using RequestParam
86 Submit information from Contact page using POJO object
87 Define actions for all the links in the Home Footer page
88 Building Holidays Page of Eazy School Web Application
Deep dive of Lombok library
89 Introduction to Lombok library
90 Implementing Lombok inside Eazy School Web App
91 Demo of Slf4j annotation from Lombok library
Processing Query Params Path Variables inside Spring
92 Accepting Query Params using RequestParam annotation Theory
93 Accepting Query Params using RequestParam annotation Coding
94 Accepting Path Params using PathVariable annotation Theory
95 Accepting Path Params using PathVariable annotation Coding
Validating the input using Java Bean Hibernate Validators
96 Importance of Validations inside Web Applications
97 Introduction to Java Bean Validations
98 Adding Bean Validation annotations inside Contact POJO class
99 Adding Bean Validation related changes inside EazySchool Web Application
100 Demo of Bean Validations inside Contact form Page
Beans Web scopes inside Spring framework
101 Introduction to Spring Web Scopes
102 Use Cases of Spring Web Scopes
103 Demo of RequestScope inside Eazy School Web Application
104 Demo of SessionScope inside Eazy School Web Application
105 Demo of ApplicationScope inside Eazy School Web Application
Implement security inside Web App Spring Security Part 1
106 Introduction to Spring Security
107 Deepdive of Authentication Vs Authorization
108 Demo of Spring Security inside Eazy School Web App with default behavior
109 Configure custom credentials inside Spring Security
110 Understanding default security configurations inside Spring Security framework
111 Configure permitAll inside Web App using Spring Security
112 Configure denyAll inside Web App using Spring Security
113 Configure custom security configurations using Spring Security
114 Demo of CSRF protection CSRF Disable inside Spring Security framework
115 Configure multiple users using inMemoryAuthentication of Spring Security
116 Implement Login Logout inside Web App Part 1
117 Implement Login Logout inside Web App Part 2
118 Implement Login Logout inside Web App Part 3
119 Demo of integration between ThymeLeaf Spring Security
Exception Handling using ControllerAdvice ExceptionHandler
120 Introduction to ControllerAdvice ExceptionHandler annotations
121 Demo of ControllerAdvice ExceptionHandler annotations
Implement CSRF fix inside Web App Spring Security Part 2
122 Deep dive of CSRF attack
123 Solution for CSRF attack Theory
124 Solution for CSRF attack Coding
Deep dive on Spring Boot H2 Database Spring JDBC framework
125 Introduction to inmemory H2 Database of Spring Boot
126 Setup H2 Database inside a Spring Boot web application
127 Introduction to JDBC problems with it
128 Introduction to Spring JDBC
129 Deep dive on usage of JdbcTemplate
130 Saving Contact Message into DB using JdbcTemplate Insert operation
131 Display Contact messages from DB using JdbcTemplate select operation Part 1
132 Display Contact messages from DB using JdbcTemplate select operation Part 2
133 Update Contact messages status using JdbcTemplate update operation
134 Implementing AOP inside Eazy School Web Application
135 Display list of Holidays from H2 Database using JdbcTemplate
Setup MySQL DB in AWS migrating from H2 DB
136 Setup MYSQL DB inside AWS Part 1
137 Setup MYSQL DB inside AWS Part 2
138 Migrate from H2 Database to MYSQL Database
139 Demo of MYSQL Database changes inside Eazy School Web App
Introduction to Spring Data Spring Data JPA
140 Problems with Spring JDBC how ORM frameworks solve these problems
141 Introduction to Spring Data
142 Deepdive on RepositoryCrudRepositoryPagingAndSortingRepositoryJpaRepository
143 Introduction to Spring Data JPA
144 Migrate from Spring JDBC to Spring Data JPA Part 1
145 Migrate from Spring JDBC to Spring Data JPA Part 2
146 Migrate from Spring JDBC to Spring Data JPA Part 3
147 Migrate from Spring JDBC to Spring Data JPA Part 4
148 Deep dive on derived query methods inside Spring Data JPA
Auditing support by Spring Data JPA inside Web Applications
149 Introduction of Auditing Support by Spring Data JPA
150 Implement automatic auditing support with Spring Data JPA Part 1
151 Implement automatic auditing support with Spring Data JPA Part 2
Building Custom Validations inside Spring MVC
152 Building new user registration web page inside Eazy School Web App
153 Building Custom validations for new user registration page Part 1
154 Building Custom validations for new user registration page Part 2
155 Building Custom validations for new user registration page Part 3
156 Building Custom validations for new user registration page Part 4
Deep dive on OneToOne Relationship Fetch Types Cascade Types in ORM frameworks
157 Creating new tables required for new user registration process
158 Spring Data JPA configurations for Person Address Roles tables and entities
159 Introduction to One to One Relationship inside ORM frameworks
160 Making One to One Relationship configurations inside entity classes Theory
161 Deep dive on Fetch Types and Cascade Types in ORM frameworks
162 Making One to One Relationship configurations inside entity classes Coding
Spring Security custom Authentication using DB Password Hashing
163 Understanding Spring Security configurations for custom authentication logic
164 Implement Spring Security changes for custom authentication logic Part 1
165 Implement Spring Security changes for custom authentication logic Part 2
166 Problems with Authentication logic using plain text passwords
167 Deep dive on Encoding Encryption and Hashing for password management
168 Deep dive on PasswordEncoder BCryptPasswordEncoder
169 Implementing password hashing with BCryptPaswordEncoder Part 1
170 Implementing password hashing with BCryptPaswordEncoder Part 2
171 Quick Tip To Disable the javax validations in Spring Data JPA
Building Profile web page inside Eazy School Web App
172 Displaying Profile link inside Dashboard web page
173 Displaying Profile Web Page on click of profile link in Dashboard
174 Fetch data from DB and display on the Profile web page
175 Save Address Data into DB from Profile Page
Deep dive on OneToMany ManyToOne Relationships in ORM frameworks
176 Introduction to new enhancements related to OnetoMany ManytoOne ManytoMany
177 Displaying Classes Courses link inside Dashboard web page
178 Introduction to OneToMany ManyToOne mappings
179 Implement OneToMany ManyToOne configurations inside Entity classes
180 Displaying new Web Page on click of classes link in Dashboard
181 Add Delete Classes enhancement inside Eazy School Web App
182 Display Add Delete Students enhancement inside Eazy School Web App Part 1
183 Display Add Delete Students enhancement inside Eazy School Web App Part 2
Deep dive on ManyToMany Relationship Configurations inside ORM frameworks
184 Introduction to ManyToMany relationship in ORM frameworks
185 Implement ManyToMany configurations inside Entity classes
186 Display Add Courses enhancement inside Eazy School Web App Part 1
187 Display Add Courses enhancement inside Eazy School Web App Part 2
188 Display Add Students enhancement inside Course Web Page
189 Delete Student enhancement inside Course Web Page
190 Implement Student Dashboard related enhancements inside Eazy School Web App
Sorting Pagination inside Spring Data JPA
191 Introduction to Sorting inside Spring Data JPA
192 Implement Demo of Static Sorting
193 Implement Demo of Dynamic Sorting
194 Introduction to Pagination inside Spring Data JPA
195 Implement Demo of Pagination Dynamic Sorting Part 1
196 Implement Demo of Pagination Dynamic Sorting Part 2
Writing Custom Queries inside Spring Data JPA
197 Introduction to custom queries using QueryNamedQueryNamedNativeQuery JPQL
198 Writing Custom Queries using Query Annotation
199 Writing Custom Update Queries using QueryModifyingTransactional Annotations
200 Deep dive on NamedQueryNamedNativeQuery inside Spring Data JPA
201 Writing Custom Queries using NamedQueryNamedNativeQuery Annotations
Building REST Services using Spring framework
202 Introduction to REST Services
203 Build REST services using Spring MVC style ResponseBody annotation Theory
204 Implement REST service using Spring MVC style ResponseBody Part 1
205 Implement REST service using Spring MVC style ResponseBody Part 2
206 Deep dive Demo of RequestBody annotation
207 Implement REST Services using RestController annotation
208 Demo of save operation using Rest Service ResponseEntity
209 Demo of delete operation using Rest Service RequestEntity
210 Demo of update operation using Rest Service recap of all Rest annotations
211 Implement global error logic for Rest Services using RestControllerAdvice
212 Deep dive on CROSSORIGIN RESOURCE SHARING CORS CrossOrigin annotation
213 Sending Response in XML format in Rest Services
214 Demo of Content filter inside Rest Services using JsonIgnore annotation
Consuming Rest Services using Spring framework
215 Introduction to Consuming Rest Services inside Web Applications
216 Consuming Rest Services using OpenFeign Theory
217 Consuming Rest Services using OpenFeign Coding
218 Consuming Rest Services using RestTemplate
219 Consuming Rest Services using WebClient
Deep dive on Spring Data Rest HAL Explorer
220 Introduction to Spring Data Rest HAL Explorer
221 Deep dive of Spring Data Rest exploring Rest APIs Part 1
222 Deep dive of Spring Data Rest exploring Rest APIs Part 2
223 Exploring Rest APIs of Spring Data Rest using HAL Explorer
224 Securing Spring Data Rest APIs HAL Explorer
225 Quick Tips around Spring Data Rest
Logging Configurations inside SpringBoot
226 Introduction to Logging inside SpringBoot
227 Logging configurations for SpringBoot framework code
228 Logging configurations for Application code
229 Store log statements into a custom file and folder
Properties Configuration Profiles inside SpringBoot
230 Introduction to Externalized properties inside SpringBoot Web Applications
231 Reading properties using Value annotation
232 Reading properties using Environment interface
233 Reading properties using ConfigurationProperties Theory
234 Reading properties using ConfigurationProperties Coding
235 Introduction to Profiles in Spring
236 Implementation Demo of Profiles inside Eazy School Web App
237 Various approaches to activate Profiles inside Spring
238 Creating beans conditionally based on active profile
Deep Dive on Spring Boot Actuator Spring Boot Admin
239 Introduction to Spring Boot Actuator
240 Implement and Secure Actuator inside Eazy School Web App
241 Deepdive of Actuator endpoints
242 Exploring Actuator data using Spring Boot Admin
Deploying SpringBoot App into AWS Cloud
243 Introduction to Cloud Deployment AWS EC2 AWS Elastic Beanstalk
244 Packaging Spring Boot application for AWS Deployment
245 Deploying Spring Boot app into AWS Elastic Beanstalk
246 Switching DB inside AWS Elastic Beanstalk
247 Deleting AWS Beanstalk DB resources
Thank You Congratulations
248 Thank You Congratulations
249 Bonus lectures
Resolve the captcha to access the links!