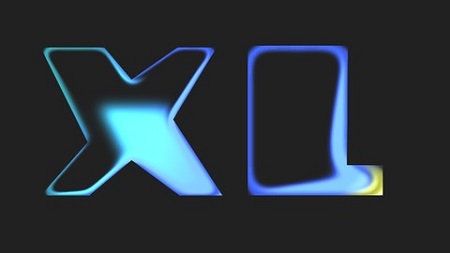
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 33 Hours | 9.69 GB
Learn concepts in linear algebra and matrix analysis, and implement them in MATLAB and Python.
You need to learn linear algebra!
Linear algebra is perhaps the most important branch of mathematics for computational sciences, including machine learning, AI, data science, statistics, simulations, computer graphics, multivariate analyses, matrix decompositions, signal processing, and so on.
You need to know applied linear algebra, not just abstract linear algebra!
The way linear algebra is presented in 30-year-old textbooks is different from how professionals use linear algebra in computers to solve real-world applications in machine learning, data science, statistics, and signal processing. For example, the “determinant” of a matrix is important for linear algebra theory, but should you actually use the determinant in practical applications? The answer may surprise you, and it’s in this course!
If you are interested in learning the mathematical concepts linear algebra and matrix analysis, but also want to apply those concepts to data analyses on computers (e.g., statistics or signal processing), then this course is for you! You’ll see all the maths concepts implemented in MATLAB and in Python.
Unique aspects of this course
- Clear and comprehensible explanations of concepts and theories in linear algebra.
- Several distinct explanations of the same ideas, which is a proven technique for learning.
- Visualization using graphs, numbers, and spaces that strengthens the geometric intuition of linear algebra.
- Implementations in MATLAB and Python. Com’on, in the real world, you never solve math problems by hand! You need to know how to implement math in software!
- Beginning to intermediate topics, including vectors, matrix multiplications, least-squares projections, eigendecomposition, and singular-value decomposition.
- Strong focus on modern applications-oriented aspects of linear algebra and matrix analysis.
- Intuitive visual explanations of diagonalization, eigenvalues and eigenvectors, and singular value decomposition.
- Improve your coding skills! You do need to have a little bit of coding experience for this course (I do not teach elementary Python or MATLAB), but you will definitely improve your scientific and data analysis programming skills in this course. Everything is explained in MATLAB and in Python (mostly using numpy and matplotlib; also sympy and scipy and some other relevant toolboxes).
Benefits of learning linear algebra
- Understand statistics including least-squares, regression, and multivariate analyses.
- Improve mathematical simulations in engineering, computational biology, finance, and physics.
- Understand data compression and dimension-reduction (PCA, SVD, eigendecomposition).
- Understand the math underlying machine learning and linear classification algorithms.
- Deeper knowledge of signal processing methods, particularly filtering and multivariate subspace methods.
- Explore the link between linear algebra, matrices, and geometry.
- Gain more experience implementing math and understanding machine-learning concepts in Python and MATLAB.
Why I am qualified to teach this course:
I have been using linear algebra extensively in my research and teaching (in MATLAB and Python) for many years. I have written several textbooks about data analysis, programming, and statistics, that rely extensively on concepts in linear algebra.
What you’ll learn
- Understand theoretical concepts in linear algebra, including proofs
- Implement linear algebra concepts in scientific programming languages (MATLAB, Python)
- Apply linear algebra concepts to real datasets
- Ace your linear algebra exam!
- Apply linear algebra on computers with confidence
- Gain additional insights into solving problems in linear algebra, including homeworks and applications
- Be confident in learning advanced linear algebra topics
- Understand some of the important maths underlying machine learning
- Manually corrected closed-captions
Table of Contents
Introductions
1 What is linear algebra
2 Linear algebra applications
3 An enticing start to a linear algebra course!
4 How best to learn from this course
5 Maximizing your Udemy experience
6 Using MATLAB, Octave, or Python in this course
Vectors
7 Exercises + code
8 Algebraic and geometric interpretations of vectors
9 Vector addition and subtraction
10 Vector-scalar multiplication
11 Vector-vector multiplication the dot product
12 Dot product properties associative, distributive, commutative
13 Code challenge dot products with matrix columns
14 Vector length
15 Dot product geometry sign and orthogonality
16 Code challenge dot product sign and scalar multiplication
17 Code challenge is the dot product commutative
18 Vector Hadamard multiplication
19 Outer product
20 Vector cross product
21 Vectors with complex numbers
22 Hermitian transpose (a.k.a. conjugate transpose)
23 Interpreting and creating unit vectors
24 Code challenge dot products with unit vectors
25 Dimensions and fields in linear algebra
26 Subspaces
27 Subspaces vs. subsets
28 Span
29 Linear independence
30 Basis
Introduction to matrices
31 Exercises + code
32 Matrix terminology and dimensionality
33 A zoo of matrices
34 Matrix addition and subtraction
35 Matrix-scalar multiplication
36 Code challenge is matrix-scalar multiplication a linear operation
37 Transpose
38 Complex matrices
39 Diagonal and trace
40 Code challenge linearity of trace
41 Broadcasting matrix arithmetic
Matrix multiplications
42 Exercises + code
43 Introduction to standard matrix multiplication
44 Four ways to think about matrix multiplication
45 Code challenge matrix multiplication by layering
46 Matrix multiplication with a diagonal matrix
47 Order-of-operations on matrices
48 Matrix-vector multiplication
49 D transformation matrices
50 Code challenge Pure and impure rotation matrices
51 Code challenge Geometric transformations via matrix multiplications
52 Additive and multiplicative matrix identities
53 Additive and multiplicative symmetric matrices
54 Hadamard (element-wise) multiplication
55 Code challenge symmetry of combined symmetric matrices
56 Multiplication of two symmetric matrices
57 Code challenge standard and Hadamard multiplication for diagonal matrices
58 Code challenge Fourier transform via matrix multiplication!
59 Frobenius dot product
60 Matrix norms
61 Code challenge conditions for self-adjoint
62 What about matrix division
Matrix rank
63 Exercises + code
64 Rank concepts, terms, and applications
65 Computing rank theory and practice
66 Rank of added and multiplied matrices
67 Code challenge reduced-rank matrix via multiplication
68 Code challenge scalar multiplication and rank
69 Rank of A^TA and AA^T
70 Code challenge rank of multiplied and summed matrices
71 Making a matrix full-rank by shifting
72 Code challenge is this vector in the span of this set
73 Course tangent self-accountability in online learning
Matrix spaces
74 Exercises + code
75 Column space of a matrix
76 Column space, visualized in code
77 Row space of a matrix
78 Null space and left null space of a matrix
79 Columnleft-null and rownull spaces are orthogonal
80 Dimensions of columnrownull spaces
81 Example of the four subspaces
82 More on Ax=b and Ax=0
Solving systems of equations
83 Exercises + code
84 Systems of equations algebra and geometry
85 Converting systems of equations to matrix equations
86 Gaussian elimination
87 Echelon form and pivots
88 Reduced row echelon form
89 Code challenge RREF of matrices with different sizes and ranks
90 Matrix spaces after row reduction
Matrix determinant
91 Exercises
92 Determinant concept and applications
93 Determinant of a 2×2 matrix
94 Code challenge determinant of small and large singular matrices
95 Determinant of a 3×3 matrix
96 Code challenge large matrices with row exchanges
97 Find matrix values for a given determinant
98 Code challenge determinant of shifted matrices
99 Code challenge determinant of matrix product
Matrix inverse
100 Exercises + code
101 Matrix inverse Concept and applications
102 Computing the inverse in code
103 Inverse of a 2×2 matrix
104 The MCA algorithm to compute the inverse
105 Code challenge Implement the MCA algorithm!!
106 Computing the inverse via row reduction
107 Code challenge inverse of a diagonal matrix
108 Left inverse and right inverse
109 One-sided inverses in code
110 Proof the inverse is unique
111 Pseudo-inverse, part 1
112 Code challenge pseudoinverse of invertible matrices
Projections and orthogonalization
113 Exercises + code
114 Projections in R^2
115 Projections in R^N
116 Orthogonal and parallel vector components
117 Code challenge decompose vector to orthogonal components
118 Orthogonal matrices
119 Gram-Schmidt procedure
120 QR decomposition
121 Code challenge Gram-Schmidt algorithm
122 Matrix inverse via QR decomposition
123 Code challenge Inverse via QR
124 Code challenge Prove and demonstrate the Sherman-Morrison inverse
125 Code challenge A^TA = R^TR
Least-squares for model-fitting in statistics
126 Exercises + code
127 Introduction to least-squares
128 Least-squares via left inverse
129 Least-squares via orthogonal projection
130 Least-squares via row-reduction
131 Model-predicted values and residuals
132 Least-squares application 1
133 Least-squares application 2
134 Code challenge Least-squares via QR decomposition
Eigendecomposition
135 Exercises + code
136 What are eigenvalues and eigenvectors
137 Finding eigenvalues
138 Shortcut for eigenvalues of a 2×2 matrix
139 Code challenge eigenvalues of diagonal and triangular matrices
140 Code challenge eigenvalues of random matrices
141 Finding eigenvectors
142 Eigendecomposition by hand two examples
143 Diagonalization
144 Matrix powers via diagonalization
145 Code challenge eigendecomposition of matrix differences
146 Eigenvectors of distinct eigenvalues
147 Eigenvectors of repeated eigenvalues
148 Eigendecomposition of symmetric matrices
149 Eigenlayers of a matrix
150 Code challenge reconstruct a matrix from eigenlayers
151 Eigendecomposition of singular matrices
152 Code challenge trace and determinant, eigenvalues sum and product
153 Generalized eigendecomposition
154 Code challenge GED in small and large matrices
Singular value decomposition
155 Exercises + code
156 Singular value decomposition (SVD)
157 Code challenge SVD vs. eigendecomposition for square symmetric matrices
158 Relation between singular values and eigenvalues
159 Code challenge U from eigendecomposition of A^TA
160 Code challenge A^TA, Av, and singular vectors
161 SVD and the four subspaces
162 Spectral theory of matrices
163 SVD for low-rank approximations
164 Convert singular values to percent variance
165 Code challenge When is UV^T valid, what is its norm, and is it orthogonal
166 SVD, matrix inverse, and pseudoinverse
167 Condition number of a matrix
168 Code challenge Create matrix with desired condition number
Quadratic form and definiteness
169 Exercises + code
170 The quadratic form in algebra
171 The quadratic form in geometry
172 The normalized quadratic form
173 Code challenge Visualize the normalized quadratic form
174 Eigenvectors and the quadratic form surface
175 Application of the normalized quadratic form PCA
176 Quadratic form of generalized eigendecomposition
177 Matrix definiteness, geometry, and eigenvalues
178 Proof A^TA is always positive (semi)definite
179 Proof Eigenvalues and matrix definiteness
Bonus section
180 Bonus lecture
Resolve the captcha to access the links!