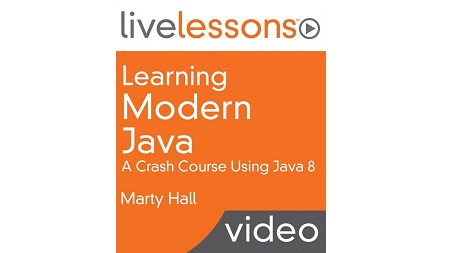
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 17h 46m | 4.89 GB
Java is the world’s most popular and widely applied programming language, but it is large, complex, and sometimes difficult to get started with. These LiveLessons supply a practical, hands-on introduction to programming with Java 8, the latest version of the language.
The course provides thorough coverage of the foundational Java topics: basic syntax, object-oriented programming, handling exceptions, core data structures, and generic types. It also gives fast-moving coverage of some of the most important libraries: concurrent programming with Java threads, parallel programming with fork/join, network programming, file I/O, and serialization. Finally, it gives detailed explanation of the syntax and usage of lambda expressions and streams, the most important new features in Java 8.
In each section, it gives details on the most important topics, surveys more advanced or lesser-used topics, stresses best practices, and provides plenty of working examples.
If you are new to Java and want to quickly learn the full range of Java programming, but in the context of the latest version (Java 8), continue on: you are in the right place. If, however, you are already comfortable with previous Java versions and want to learn only the new Java 8 features, see Java 8 Lambda Expressions and Streams instead.
In these LiveLessons, expert Java developer, instructor, and author Marty Hall gives a crash course on Java programming. This fast-moving video series is aimed at developers who have used other languages, but who have little or no Java experience.
The first section looks at installing Java and Eclipse, making projects, and understanding loops, conditionals, and other basic Java syntax.
The second section covers object-oriented programming using the Java 8 style. Topics include classes, methods, constructors, interfaces, abstract classes, enums, and lots of guidance on style and best OOP practices.
The third section looks at exception handling, lists and maps, generic types, printf, inner classes, and unit testing with JUnit.
The fourth section looks at asynchronous event handling, concurrent programming with Java threads, and parallel programming using Java’s fork/join framework.
The fifth section looks at the syntax and usage of lambda expressions and streams, with particular emphasis on best practices and the use of parallel streams.
The final section looks at file and network I/O: Java 8 stream-based file reading, network clients, network servers, and serialization.
The source code repository for this LiveLesson can be downloaded from courses.coreservlets.com/Course-Materials/java.html
What You Will Learn
- Installing Java
- Installing and using the Eclipse development environment
- Loops and conditionals
- Strings, arrays, and other basic Java syntax
- Classes
- Instance variables (data members, fields)
- Methods (member functions)
- Overloading
- Inheritance
- OOP best practices
- Use of JavaDoc
- Encapsulation
- Interfaces using the Java 8 style
- Abstract classes
- Enums
- The toString method
- Try/catch blocks
- The @Override annotation
- Lists and maps
- Making your own methods and classes that support generic types
- printf
- The Arrays utility class
- Unit testing with JUnit
- Asynchronous event handling
- Inner classes
- Concurrent programming with Java threads
- Recognizing and dealing with race conditions
- Parallel programming with the Java fork/join framework
- Best practices for parallel applications
- Lambda expressions
- Method references
- Lambda building blocks in the java.util.function package
- Methods that return functions
- Java 8 streams
- Creating streams from lists, arrays, and individual values
- Outputting streams as lists or arrays
- Core stream methods
- The new Optional class
- Lazy evaluation and short-circuit stream operations
- Streams specialized for numbers
- Reduction operations
- Parallel streams
- Infinite (unbounded) streams
- Grouping and partitioning stream elements
- Java 8 stream-based file I/O
- Network programming: clients
- Network programming: servers
- Multithreaded network servers
- Object serialization
Table of Contents
1 Learning Modern Java – Introduction
2 Topics
3 1.1 Installing Java
4 1.2 Installing and Using Eclipse
5 Summary
6 Topics
7 2.1 Setup, Execution, and Most Basic Syntax
8 2.2 The + Operator, Array Basics, and Command Line Args
9 2.3 Loops
10 2.4 Class Structure and Formatting
11 2.5 Conditionals
12 2.6 Strings
13 2.7 More on Arrays
14 2.8 Math Routines
15 2.9 Reading Input
16 Summary
17 Topics
18 3.1 Overview
19 3.2 Basics
20 3.3 Instance Variables
21 3.4 Methods
22 3.5 Constructors
23 3.6 Interactive Example – Person Class
24 Summary
25 Topics
26 4.1 Overloading
27 4.2 OOP Best Practices – Encapsulation and JavaDoc
28 4.3 Inheritance
29 4.4 Inheritance Example – Person Class
30 4.5 The toString Method
31 Summary
32 Topics
33 5.1 Sample Problem
34 5.2 Attempted Problem Solutions
35 5.3 Abstract Classes
36 5.4 Interfaces
37 5.5 @Override
38 5.6 Visibility Modifiers
39 5.7 Enums
40 5.8 JavaDoc Options
41 5.9 The Classpath
42 Summary
43 Topics
44 6.1 Motivation
45 6.2 Security Restrictions
46 6.3 Basic Applet Structure
47 6.4 Drawing in Applets – Basics
48 6.5 The Value of @Override
49 6.6 Applet Life Cycle Methods
50 6.7 Other Applet Methods
51 6.8 Attributes of the HTML Applet Tag
52 6.9 Applet Parameters – Letting the HTML Author Supply Data
53 6.10 Drawing in Applets – More Details
54 6.11 Loading and Drawing Images
55 6.12 Basic Try_Catch Blocks
56 Summary
57 Topics
58 7.1 Lists
59 7.2 List Operation Performance
60 7.3 Using Generic Types
61 7.4 Autoboxing
62 7.5 Maps
63 7.6 Building Genericized Methods and Classes
64 7.7 The Arrays Utility Class
65 7.8 printf
66 7.9 Varargs
67 7.10 StringBuilder
68 Summary
69 Topics
70 8.1 Static Imports
71 8.2 JUnit Overview
72 8.3 Modern Approach
73 8.4 Traditional Approach
74 Summary
75 Topics
76 9.1 Overview
77 9.2 Using Seperate Listener Classes – Basics
78 9.3 Using Seperate Listener Classes – Drawing
79 9.4 Implementing a Listener Interface
80 9.5 Using Named Inner Classes
81 9.6 Using Anonymous Inner Classes
82 9.7 Preview – Using Java 8 Lambdas
83 Summary
84 Topics
85 10.1 Overview
86 10.2 Basic Steps for Concurrent Programming
87 10.3 Approach One – Separate Class that Implements Runnable
88 10.4 Approach Two – Main App Implements Runnable
89 10.5 Approach Three – Inner Class that Implements Runnable
90 10.6 Preview of Approach Four – Lambda Expressions
91 10.7 Summary of Approaches with Pros and Cons
92 10.8 Making a Reusable Multithreaded Server – Template
93 10.9 Race Conditions – Problem
94 10.10 Race Conditions – Solution
95 10.11 Helpful Thread-Related Methods
96 10.12 Brief Summary of Advanced Topics
97 10.13 Tricky Synchronization Problem
98 Summary
99 Topics
100 11.1 Overview
101 11.2 General Fork_Join Approach
102 11.3 Aside – Reusable Timing Code
103 11.4 Summing Large Array of Doubles – Version 1
104 11.5 Summing Large Array of Doubles Version 1 – Verification Step
105 11.6 Making Reusable Parallel Array Processor
106 11.7 Summing Large Array of Doubles – Version 2
107 11.8 Computing Minimum of Expensive Operation
108 11.9 Generating Large Prime Numbers
109 Summary
110 Topics
111 12.1 Motivation and Overview
112 12.2 Lambdas – Most Basic Form
113 12.3 Type Inferencing
114 12.4 Expression for Body – Implied Return Values
115 12.5 Omitting Parens
116 12.6Summary – Making Lambdas More Succinct
117 12.7 Example – Numerical Integration
118 12.8 Making a Reusable Timing Utility
119 12.9 A Few More Samples
120 Summary
121 Topics
122 13.1 The @FunctionalInterface Annotation
123 13.2 Method References
124 13.3 Lambda Variable Scoping in Lambdas
125 13.4 Effectively Final Local Variables
126 Summary
127 Topics
128 14.1 Overview – Lambda Building Blocks
129 14.2 Simply-Typed Building Blocks
130 14.3 Generic Building Blocks – Predicate
131 14.4 Generic Building Blocks – Function
132 14.5 Other Generic Building Blocks
133 Summary
134 Topics
135 15.1 Java 8 Interfaces – Static Methods
136 15.2 Java 8 Interfaces – Default Methods
137 15.3 Resolving Conflicts with Default Methods
138 15.4 Higher Order Functions – Methods that Return Functions
139 15.5 Higher Order Functions in Predicate
140 15.6 Higher Order Functions in Function
141 15.7 Higher Order Functions in Consumer
142 15.8 Higher Order Functions in Your Own Code
143 Summary
144 Topics
145 16.1 Overview of Streams
146 16.2 Getting Standard Data Structures into and out of Streams
147 16.3 Core Stream Methods – Overview
148 16.4 forEach – Calling a Lambda on Each Element of a Stream
149 16.5 map – Transforming a Stream by Passing Each Element through a Function
150 16.6 filter – Keeping Only the Elements that Pass a Predicate
151 16.7 findFirst – Returning the First Element of a Stream while Short-Circuiting Earlier Operations
152 16.8 Lazy Evaluation
153 Summary
154 Topics
155 17.1 Operations that Limit the Stream Size – limit, skip
156 17.2 Operations that Use Comparisons – sorted, min, max, distinct
157 17.3 Operations that Check Matches – allMatch, anyMatch, noneMatch, count
158 17.4 Number-Specialized Streams
159 17.5 The reduce Method and Related Reduction Operations
160 Summary
161 Topics
162 18.1 Grouping Stream Elements – Fancy Uses of collect
163 18.2 Parallel Streams
164 18.3 Infinite (Unbounded On-the-Fly) Streams
165 Summary
166 Topics
167 19.1 More on try_catch Blocks
168 19.2 Paths
169 19.3 Simple File Reading
170 19.4 File Reading Examples
171 19.5 Simple File Writing
172 19.6 Exploring Folders
173 19.7 Faster and More Flexible File Writing
174 Summary
175 Topics
176 20.1 Overview
177 20.2 Basic Steps for a Client to Connect to a Server
178 20.3 A Reusable Network Client Base Class
179 20.4 Aside – String Formatting and Parsing
180 20.5 Problems with Blocking IO
181 20.6 Talking to Web Servers – General
182 20.7 Talking to Web Servers – A Java Client
183 Summary
184 Topics
185 21.1 Basics
186 21.2 Simple Warmup – A Single-Threaded Server
187 21.3 A Base Class for a Multithreaded Server
188 21.4 A Simple Multithreaded HTTP Server
189 Summary
190 Topics
191 22.1 Overview
192 22.2 Sending Data
193 22.3 Receiving Data
194 22.4 Example – Sending Entire Window to File or Socket
195 Summary
196 Learning Modern Java – Summary
Resolve the captcha to access the links!