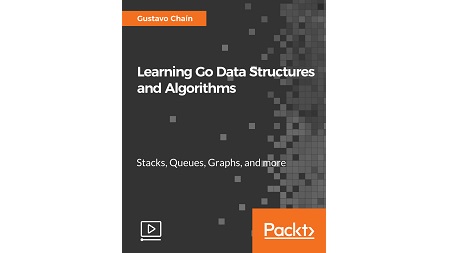
English | MP4 | AVC 1920×1080 | AAC 48KHz 2ch | 2h 28m | 1.35 GB
Delve into Go’s powerful data structures and algorithms with some easy tricks
Golang has been trending in the tech-world for the last 2 years with tremendous improvements to the language. Many developers and organizations are slowly migrating to Golang, adopting its fast, lightweight and inbuilt concurrency features. This brings the need to learn data structures and algorithms with this growing language. This video course will be your companion as it takes you through data structures and algorithms in Go, helping you get up-and-running as a confident Go programmer.
You will begin by understanding the basic Data types and Structures in Go. Moving forward, you will learn the power of linked lists and doubly linked lists in Go and then learn to implement linear data structures such as stacks and queues. Also, implement binary searches and trees and will explore sorting algorithms in detail.
From here, you will learn about graph algorithms and also be introduced to some common data structures used while working with strings. You will learn common techniques and structures used in tasks such as preprocessing, modeling, and transforming data.
By the end of this course, you would have mastered functional and reactive implementations of traditional data structures in an easy and efficient manner
In this course, you will explore data structures and algorithms in Golang with an easy to follow practical method.
What You Will Learn
- Understanding the basics to get started with Golang
- Using various data structures
- Implementing stacks and queues
- Applying algorithms such as binary and trees
- Exploring different concurrency models for data processing
- Build your own tiny distributed search engine
- Implementation of stacks and queues
- Applying algorithms such as Binary and trees
- Exploring different concurrency models for data processing
- Build your own tiny distributed search engine
Table of Contents
Getting Started with Go!#
1 The Course Overview
2 Basics of Go
3 Writing a Hello World Program
Exploring Core Constructs
4 Variables and Elementary Types
5 Applying Functions
6 Forming Control Structures
7 Data Structures – Array, Slices, and Maps
8 Structs and Methods
9 Error Handling
Applying Algorithms
10 Single and Double Linked Lists
11 Implementing Stacks
12 Implementing Queue (Alternative Channel Implementation)
13 Binary Search and Trees
Discovering Concurrency
14 Generating Channels
15 Multiplex and Demultiplex with Fan In Out
16 Implementing Worker Pools
17 Building a (Tiny) Distributed Search Engine
Resolve the captcha to access the links!