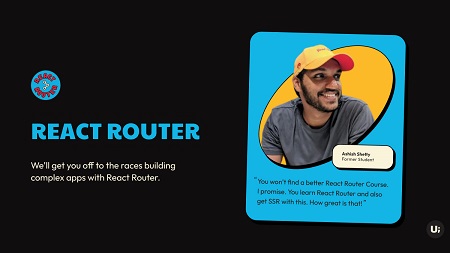
English | MP4 | AVC 1920×1080 | AAC 44KHz 2ch | 41 Lessons (3h 15m) | 541 MB
We’ll get you off to the races building complex apps with React Router.
Fundamentals
In this section you’ll learn the fundamentals of React Router including how to create routes and how navigate users between those routes.
URL Parameters
In this section, you’ll break down the ‘URL Parameters’ example on the React Router documentation to learn how you to leverage URL parameters.
Query Strings
In this section we’ll break down how to implement and parse query strings with React Router.
Customizing Link
Because React Router is just components, composing your own custom Link component is pretty straightforward. In this section we’ll do just that.
Nested Routes
In this section you’ll learn how to utilize nested routes with React Router.
Programmatically Navigating
The goal of this section is to break down the correct approaches to programmatically navigating with React Router.
Protected Routes
In this section, you’ll learn how to create authenticated routes (routes that only certain users can access based on their authentication status) using React Router.
Code Splitting
In this section we’ll take a look at not only what code splitting is and how to do it, but also how to implement it with React Router.
Route Config
If you need it, you can still have a central route config with React Router. In this section you’ll learn how.
Animated Transitions
In this section you’ll learn to how to add animated transitions to an app using React Router.
Protected Routes
In this section, you’ll learn how to create authenticated routes (routes that only certain users can access based on their authentication status) using React Router.
404 Pages
In this section you’ll learn how to implement catch all routes for handling 404 pages in a React app with React Router.
Passing Props to Link
In this section you’ll learn how ot pass props to React Router’s Link component.
Sidebars
When building an app with React Router, often you’ll want to implement a sidebar or breadcrumb navbar. In this section you’ll learn how.
Preventing Transitions
Many times when building an app with React Router, you want to warn the user before they navigate away from a specific route.
Table of Contents
The Fundamentals
(Solution) The Fundamentals
(Project) Introduction
(Project) Initial Routes
URL Parameters
(Solution) URL Parameters
(Project) Home
Query Strings
(Solution) Query Strings
(Project) Team Page
Customizing React Router’s Link Component
(Solution) Customizing Link
(Project) Players
Nested Routes
(Solution) Nested Routes
(Solution) Nested Routes – Outlet
Project – Player
(Project) Team
(Project) Articles
(Project) Loading
Programmatically Navigate
(Solution) Programmatically Navigate (Declarative)
(Solution) Programmatically Navigate (Imperative)
(Project) Navigate
Code Splitting
(Solution) React.lazy
(Project) Code Splitting
Route Configs
(Solution) Route Config
(Project) Config
Protected Routes
(Solution) Protected Routes
Handling 404 Pages
(Solution) Catch All Routes
Passing Props to Link
(Solution) Passing Props to Link
Sidebars
(Solution) Sidebar
Passing Props to React Router Components
Recursive Paths
Outro + Next Steps
Resolve the captcha to access the links!