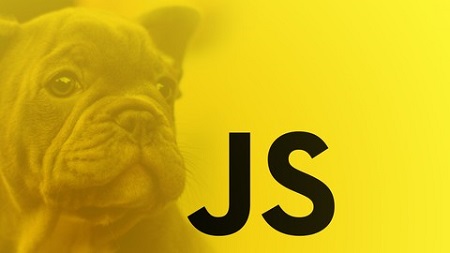
English | MP4 | AVC 1280×720 | AAC 48KHz 2ch | 27 Hours | 11.7 GB
Understand the JavaScript language itself, Node.js, MongoDB, The Web Browser and More To Create Meaningful Applications
Learn the incredibly popular and in demand JavaScript language. This course makes no assumptions of prior computer programming experience. We begin with the very basics and slowly but surely work our way up to writing JavaScript code to power every aspect of an application.
There are countless JavaScript courses in the world; here’s what makes this one unique:
A strong emphasis on the “why” and not just the “how”
As few assumptions as possible; it’s a pet peeve of mine when instructors assume I know something I don’t
As few “just download my existing project to get you up and running” moments as possible. It’s another pet peeve of mine when instructors have you use an existing solution that just “automagically” works and you miss a potential learning experience of setting it up yourself. We do copy-and-paste HTML templates (since the focus of the course is not about HTML) but aside from that I explain things from the ground up.
Here’s what we’ll learn in the course:
- The JavaScript language itself
- The Web Browser Environment
- The Node.js environment
- The MongoDB environment
- The Express framework for creating servers
- User registration & user-generated content
- Authentication (both stateful with sessions and stateless with JSON Web Tokens)
- … and much more!
Table of Contents
Welcome To The Course!
1 Where Do We Begin
The 10 Days of JavaScript The Language Itself
2 Getting Started
3 Miscellaneous Info (Part 1)
4 Miscellaneous Info (Part 2)
5 Building To-Do App (Part 1)
6 Building To-Do App (Part 2)
7 Functions
8 Objects
9 Arrays
10 Making Decisions
11 Higher-Order Functions
12 Returning vs Mutating
13 Scope & Context (Part 1)
14 Scope & Context (Part 2)
Server Basics
15 Why Do We Need a Server
16 Node.js Intro
17 Text Editor Software
18 Our First Basic Server
19 Express Intro (Part 1)
20 Express Intro (Part 2)
21 What’s Next (Big Picture)
Database Basics
22 First Taste of a Database
23 Updating a Database Item (Part 1)
24 Updating a Database Item (Part 2)
25 Deleting a Database Item
26 Create New Item Without Page Reload
27 Client-Side Rendering
28 What About Security
29 Quick Note For Mac Users
30 Pushing Our App Onto The Internet
31 Pushing Future Changes To Your Heroku App
32 Note for Advanced Students
33 CRUD Actually Working With A Database
34 Initial Setup for App #1
35 Save Time Automatic Node App Restarts
36 Quick Note About MongoDB Warning in Command-Line
37 Connecting Node App to Database
38 Installing NPM Packages Without Stopping ServerApp
39 Reading Data from a Database
Starting Our Complex App (App #2)
40 What’s Next
41 Connecting To Database In a Reusable Fashion
42 Best Practice Time Out Environment Variables
43 Quick Note
44 Letting Users Log In
45 What is a Promise (Part 1)
46 What is a Promise (Part 2)
47 Running Multiple Promises Efficiently When Order Doesn’t Matter
48 Hashing User Passwords
49 How Can We Identify or Trust a Request
50 For Those Who Are In a Hurry (Security Note)
51 Let’s Begin App #2
52 Understanding Sessions
53 Letting Users Logout
54 Adding Flash Messages
55 User Registration Improvements (Part 1)
56 User Registration Improvements (Part 2)
57 Adding User Profile Photos
58 Important Note About Package Versions To Save You Frustration
59 What Is A Router
60 What Is A Controller
61 Security Note
62 What Is A Model
63 Adding Validation To Our Model
64 Quick Misc. Clean Up
User Created Posts
65 Letting Users Create Posts (Part 1)
66 View Posts by Author
67 Is the Current Visitor the Owner of the Post
68 The Edit Screen for a Post
69 Updating Posts in Database (Part 1)
70 Updating Posts in Database (Part 2)
71 Miscellaneous Improvements
72 Markdown Safe User Generated HTML
73 Make This Quick Edit To Your Code
74 Let Users Delete a Post
75 Letting Users Create Posts (Part 2)
76 Post Model (Part 1)
77 Post Model (Part 2)
78 Viewing a Post (Part 1)
79 Viewing a Post (Part 2)
80 Performing a Lookup in MongoDB (Part 1)
81 Performing a Lookup in MongoDB (Part 2)
82 User Profile Screen
Live Search Feature
83 Staying Organized Front-End JavaScript
84 Showing and Hiding Search Overlay
85 Responding to Key Press Events
86 Back-End Aspect of Search
87 Generating HTML for Search Results (Part 1)
88 Generating HTML for Search Results (Part 2)
89 Fixing The Month for Post Dates in Search Results
90 Sanitizing User Generated HTML on the Front-End
Letting Users Follow Each Other
91 Letting Users Follow Each Other
92 Saving a Follow Action Into Our Database
93 Stop Following a User (Part 1)
94 Stop Following a User (Part 2)
95 Profile Followers Screen (Part 1)
96 Profile Followers Screen (Part 2)
97 Following Screen (Part 1)
98 Following Screen (Part 2)
99 Homepage Feed (Posts From Those You Follow)
Live Chat (Socket.IO)
100 Beginning Chat Feature
101 Socket.IO (Part 1)
102 Socket.IO (Part 2)
103 Finishing Chat (Part 1)
104 Finishing Chat (Part 2)
105 Quick Misc. Feature Dynamic Title Tag
Live Validation for Registration Form
106 Live Form Validation (Part 1)
107 Live Form Validation (Part 2)
108 Live Form Validation (Part 3)
109 Live Form Validation (Part 4)
What is CSRF (Security)
110 Preventing Cross-Site Request Forgery (CSRF)
111 Adjusting Our App To Use CSRF Token
Let’s Create an API
112 Setting Up The Skeleton for an API
113 JSON Web Tokens (Part 1)
114 JSON Web Tokens (Part 2)
115 Finishing API and Understanding CORS
Deploying Complex App to Heroku
116 Pushing Our Complex App Up Onto The Web
117 Sending Email From Our App
Where Do We Go From Here
118 Next Steps & Career Advice
Resolve the captcha to access the links!