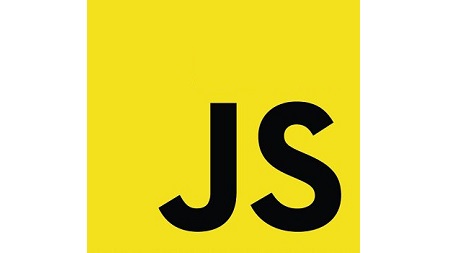
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 21h 26m | 3.09 GB
JavaScript is the most widely deployed language in the world. Whether you’re interested in writing front-end client side code, back-end code for servers, or even game development, you’ll be able to accomplish all of this and more with JavaScript. This learning path includes JavaScript tutorials for both the new programmer looking to get started and the advanced web developer wanting to solidify and enhance their skills.
What you will learn
- Basics of programming and the JavaScript syntax
- Built-in types
- JavaScript operators
- Statements
- Exception handling
- Functions
- Arrays
- Objects
- Object-oriented programming in JavaScript
Pre-requisites
A minimal understanding of basic programming concepts needed to start this path. No previous JavaScript experience needed.
Beginner
If you are new to programming, look no further. These beginning courses will give you a strong foundation in both programming and the JavaScript language.
Intermediate
The courses in this section build off of the foundation set in the beginner area and dive deeper into concepts including objects, scopes, and design patterns.
Advanced
These courses are designed to take your existing JavaScript knowledge and teach you how to leverage patterns and practices to take your JavaScript skills to the next level.
Table of Contents
Beginner
HTML, CSS, and JavaScript The Big Picture
1 Course Overview
2 Introduction
3 A Look into the History of the Web
4 How the Magic of the Web Works
5 Why Learn About HTML, CSS, and JavaScript
6 Things to Remember
7 Introduction
8 Where Did HTML Come From
9 What Is HTML
10 Working with HTML
11 Things to Remember
12 Introduction
13 Where Did CSS Come From
14 What Is CSS
15 Working with CSS
16 Things to Remember
17 Introduction
18 Where Did JavaScript Come From
19 What Is JavaScript
20 Working with JavaScript
21 Things to Remember
22 Introduction
23 Things to Remember
24 Resources to Learn More
JavaScript Getting Started
1 Course Overview
2 Introduction
3 Learning JavaScript in Plunker
4 Hello World
5 Introduction
6 Storing Information in Variables
7 Understanding Code Errors
8 Strings and Numeric Variables
9 Simple Operators
10 Commenting Code
11 Starting Our Blackjack Application
12 Summary
13 Introduction
14 Type Basics – Strings and Numbers
15 Boolean Types
16 undefined and null
17 Storing Multiple Values in Arrays
18 Array Features
19 Adding Arrays to Blackjack
20 Summary
21 Introduction
22 Conditionals Using if()
23 if … else
24 switch and case
25 Looping with for()
26 Looping with while()
27 Adding Loops to Blackjack
28 Summary
29 Introduction
30 Function Basics
31 Passing Information to Functions
32 Function Return Values
33 Function Scope
34 Adding Functions to Blackjack
35 Summary
36 Introduction
37 Object Basics
38 Passing Objects to Functions
39 Arrays of Objects
40 JavaScript Built-in Objects
41 Adding Objects to Blackjack
42 Summary
43 Introduction
44 Setting up a Web Page
45 Changing Text
46 Handling a Button Click
47 Removing and Adding Elements
48 Adding a User Interface to Blackjack
49 Summary
50 Introduction
51 Setting up the Game
52 Shuffling Cards
53 Calculating the Score
54 Taking a Card or Staying
55 Completing the Game
56 Summary
57 Course Summary
JavaScript Fundamentals
Course Overview
1 Course Overview
2 Course Objectives and Overview
3 JavaScript Versions and History
4 Tooling for This Course
5 Setting up a Development Environment
6 Introduction
7 Constants
8 let and var for Variable Declarations
9 Rest Parameters
10 Destructuring Arrays
11 Destructuring Objects
12 Spread Syntax
13 typeof()
14 Common Type Conversions
15 Controlling Loops
16 Summary
17 Introduction
18 Equality Operators
19 Unary Operators
20 Logical (Boolean) Operators
21 Relational Operators
22 Conditional Operator
23 Assignment Operators
24 Operator Precedence
25 Summary
26 Introduction
27 Function Scope
28 Block Scope
29 IIFE’s
30 Closures
31 The this Keyword
32 call and apply
33 bind
34 Arrow Functions
35 Default Parameters
36 Summary
37 Introduction
38 Constructor Functions
39 Prototypes
40 Expanding Objects Using Prototypes
41 JSON – JavaScript Object Notation
42 Array Iteration
43 Summary
44 Introduction
45 Class Basics
46 Constructors and Properties
47 Methods
48 Inheritance
49 Creating a Module
50 Importing a Module
51 Summary
52 Introduction
53 The window Object
54 Timers
55 The location Object
56 The document Object
57 Selecting DOM Elements
58 Modifying DOM Elements
59 Summary
60 Introduction
61 Errors in JavaScript
62 Error Handling Using try and catch
63 finally
64 Developer Defined Errors
65 Creating a Promise
66 Settling a Promise
67 Summary
68 Introduction
69 HTTP Requests Using XHR
70 HTTP Requests Using jQuery
71 HTTP POST Using jQuery
72 Summary
73 Introduction
74 Preventing Form Submission
75 Accessing Form Fields
76 Showing Validation Errors
77 Posting from JavaScript
78 Summary
79 Introduction
80 Chrome Developer Tools and Security
81 Security and the eval() Function
82 Preventing Man-in-the-middle Attacks
83 Cross-site Scripting (XSS)
84 Building Your Application for Production
85 Summary and Course Wrap-up
Intermediate
JavaScript Objects and Prototypes
1 Introduction to JavaScript Objects and Prototypes
2 Getting Started with Plunker
3 Using Object Literals to Create JavaScript Objects
4 The Dynamic Nature of JavaScript
5 Using Constructor Functions to Create JavaScript Objects
6 Using Object.create() to Create JavaScript Objects
7 Using ECMAScript 6 Classes to Create JavaScript Objects
8 Summary
9 Introduction to JavaScript Object Properties
10 Using Bracket Notation to Access JavaScript Properties
11 Using JavaScript Property Descriptors
12 Using the Writable Attribute
13 Using the Enumerable Attribute
14 Using the Configurable Attribute
15 Using Getters and Setters
16 Summary
17 Introduction to Prototypal Inheritance
18 Getting Started with JavaScript Prototypes
19 What Is a Prototype
20 Instance vs. Prototype Properties
21 A Graphical Overview of Prototypes
22 Changing a Functions Prototype
23 Multiple Levels of Inheritance
24 Creating Your Own Prototypal Inheritance Chains
25 Creating Prototypes with Classes
26 Summary
Practical Design Patterns in JavaScript
1 Course Overview
2 Introduction
3 The Problem
4 Design Patterns to the Rescue
5 Physical Design Patterns
6 The Gang of Four
7 So What Is a Design Pattern Anyway
8 The Types of Patterns
9 Introduction
10 Creating Objects in JavaScript
11 Reading and Writing Attributes
12 Demo Task Creation
13 Define Property
14 Demo Define Property
15 Demo Inheritance
16 Summary
17 Introduction
18 Constructor Pattern
19 Demo Constructor Pattern
20 Prototypes
21 Demo Prototypes
22 Demo Constructor Node
23 Demo Classes
24 Demo Constructor Angular
25 Module Pattern
26 Demo Module Pattern
27 Demo Angular Module Pattern
28 Factory Pattern
29 Demo Factory Pattern
30 Singleton Pattern
31 Demo Singleton Node
32 Demo Singleton Angular
33 Summary
34 Introduction
35 Decorator Pattern
36 Demo Simple Decorator
37 Demo More Complicated Decorator
38 Demo Decorating Objects in Angular
39 Demo Decorating Services in Angular
40 Facade Pattern
41 Demo Facade Node
42 Demo Facade Angular
43 Flyweight
44 Demo Flyweight
45 Summary
46 Introduction
47 Observer Pattern
48 Demo Setting up the Environment
49 Demo Creating Our Observers
50 Demo Creating Our Observer List
51 Demo Adding Our Subject
52 Demo Removing Observers
53 Mediator Pattern
54 Demo Mediator Pattern
55 Command Pattern
56 Demo Command Pattern
57 Summary
Advanced
Advanced Techniques in JavaScript and jQuery
1 Introduction
2 Outline
3 Traditional JavaScript Functions
4 Draw Version 1
5 Extending Draw-Two Parameters
6 Draw-Four Parameters
7 Draw-Six Parameters
8 Stub Functions
9 Default Parameters
10 All Default Values
11 Changing to an Object Parameter
12 Legacy Support with Object
13 Default Values in an Object
14 Function Summary
15 Introduction
16 Outline
17 Shorthand Methods
18 Message Function
19 More Elements
20 Propagation
21 No Shorthand
22 Summary
23 Introduction
24 Outline
25 Event Handler Methods
26 Named Functions
27 Namespace
28 Delegation
29 Custom Events
30 Event Parameters
31 Summary
32 Introduction
33 Outline
34 Promise
35 Traditional AJAX Processing
36 Layout
37 Section 1 Content
38 Loading All Content
39 Common Pattern
40 Deferred Object
41 LoadSection Function
42 Negative Testing
43 Deferred Methods
44 Dynamic Pages
45 Creating a Deferred Object
46 Using Deferred Objects
47 Summary
JavaScript Best Practices
1 Course Overview
2 Introduction
3 Don’t Code in a Vacuum
4 Write Code in a Maintainable Way
5 Rules of the Road
6 Introduction
7 Semicolons
8 Automatic Semicolon Insertion
9 Linting
10 Curly Braces
11 Equality
12 Configuring JSHint
13 Variables
14 Functions
15 Summary
16 Introduction
17 Global Variables
18 Strict Mode
19 Read Only Properties
20 Deleting Properties
21 Duplicates
22 Octals and Hexidecimals, Oh My
23 With
24 What Is This Anyway
25 This in New Objects
26 Summary
27 Introduction
28 Callbacks
29 Promises
30 ES6 and Babel
31 Async – Await
32 Summary
33 Introduction
34 NPM Settings
35 Environmental Variables
36 Cross Platform Concerns
37 Simplify Your World
Rapid ES6 Training
1 Course Overview
2 Introduction
3 An ES6 Compatibility Chart
4 Introduction
5 let, const and Block Scoping
6 Arrow Functions
7 Default Function Parameters
8 Rest and Spread
9 Object Literal Extensions
10 for … of Loops
11 Octal and Binary Literals
12 Template Literals
13 Destructuring
14 Advanced Destructuring
15 Summary
16 Introduction and Setup
17 Module Basics
18 Named Exports in Modules
19 Class Fundamentals
20 extends and super
21 Properties for Class Instances
22 Static Members
23 new.target
24 Summary
25 Introduction
26 Symbols
27 Well-known Symbols
28 Object Extensions
29 String Extensions
30 Number Extensions
31 Math Extensions
32 RegExp Extensions
33 Function Extensions
34 Summary
35 Introduction
36 Iterators
37 Generators
38 Yielding in Generators
39 throw and return
40 Promises
41 More Promise Features
42 Summary
43 Introduction
44 Array Extensions
45 ArrayBuffers and Typed Arrays
46 DataView and Endianness
47 Map and WeakMap
48 Set and WeakSet
49 Subclassing
50 Summary
51 Introduction
52 Construction and Method Calls
53 Reflect and Prototypes
54 Reflect and Properties
55 Reflect and Property Extensions
56 Summary
57 Introduction
58 Proxies Defined
59 Available Traps
60 Get by Proxy
61 Calling Functions by Proxy
62 A Proxy as a Prototype
63 Revocable Proxies
64 Summary
Resolve the captcha to access the links!