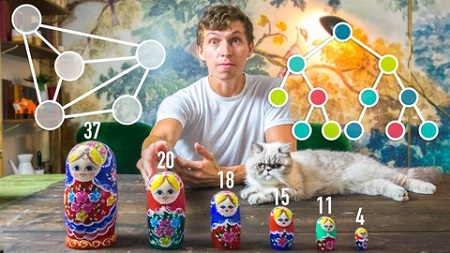
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 249 lectures (21h 52m | 3.98 GB
The Missing Computer Science and Coding Interview Bootcamp
This course crams months of computer science and interview prep material into 20 hours of video. The content is based directly on last semester of my in-person coding bootcamps, where my students go on to land 6-figure developer jobs. I cover the exact same computer science content that has helped my students ace interviews at huge companies like Google, Tesla, Amazon, and Facebook. Nothing is watered down for an online audience; this is the real deal We start with the basics and then eventually cover “advanced topics” that similar courses shy away from like Heaps, Graphs, and Dijkstra’s Shortest Path Algorithm.
I start by teaching you how to analyze your code’s time and space complexity using Big O notation. We cover the ins and outs of Recursion. We learn a 5-step approach to solving any difficult coding problem. We cover common programming patterns. We implement popular searching algorithms. We write 6 different sorting algorithms: Bubble, Selection, Insertion, Quick, Merge, and Radix Sort. Then, we switch gears and implement our own data structures from scratch, including linked lists, trees, heaps, hash tables, and graphs. We learn to traverse trees and graphs, and cover Dijkstra’s Shortest Path Algorithm. The course also includes an entire section devoted to Dynamic Programming.
What you’ll learn
- Learn everything you need to ace difficult coding interviews
- Master dozens of popular algorithms, including 6 sorting algorithms!
- Implement 10+ data structures from scratch
- Improve your problem solving skills and become a stronger developer
Table of Contents
Introduction
1 Curriculum Walkthrough
2 Join The Community!
3 What Order Should You Watch In
4 How I’m Running My Code
Big O Notation
5 Intro to Big O
6 Timing Our Code
7 Counting Operations
8 Visualizing Time Complexities
9 Official Intro to Big O
10 Simplifying Big O Expressions
11 Space Complexity
12 Logs and Section Recap
Analyzing Performance of Arrays and Objects
13 PREREQUISITES
14 Section Introduction
15 The BIG O of Objects
16 When are Arrays Slow
17 Big O of Array Methods
Problem Solving Approach
18 PREREQUISITES
19 Introduction to Problem Solving
20 Step 1 Understand The Problem
21 Step 2 Concrete Examples
22 Step 3 Break It Down
23 Step 4 Solve Or Simplify
24 Step 5 Look Back and Refactor
25 Recap and Interview Strategies
Problem Solving Patterns
26 PREREQUISITES
27 Intro to Problem Solving Patterns
28 Frequency Counter Pattern
29 Frequency Counter Anagram Challenge
30 Anagram Challenge Solution
31 Multiple Pointers Pattern
32 Multiple Pointers Count Unique Values Challenge
33 Count Unique Values Solution
34 Sliding Window Pattern
35 Divide And Conquer Pattern
% OPTIONAL Challenges
36 IMPORTANT NOTE!
37 SOLUTIONS PART 1
38 SOLUTIONS PART 2
39 SOLUTIONS PART 3
Recursion
40 PREREQUISITES
41 Story Time Martin & The Dragon
42 Why Use Recursion
43 The Call Stack
44 Our First Recursive Function
45 Our Second Recursive Function
46 Writing Factorial Iteratively
47 Writing Factorial Recursively
48 Common Recursion Pitfalls
49 Helper Method Recursion
50 Pure Recursion
Recursion Problem Set
51 START HERE!
52 SOLUTIONS FOR THIS SECTION
Bonus CHALLENGING Recursion Problems
53 NOTE ON THIS SECTION
54 SOLUTIONS PART 1
55 SOLUTIONS PART 2
Searching Algorithms
56 PREREQUISITES
57 Intro to Searching
58 Intro to Linear Search
59 Linear Search Solution
60 Linear Search BIG O
61 Intro to Binary Search
62 Binary Search PseudoCode
63 Binary Search Solution
64 Binary Search BIG O
65 Naive String Search
66 Naive String Search Implementation
Bubble Sort
67 PREREQUISITES
68 Introduction to Sorting Algorithms
69 Built-In JavaScript Sorting
70 Bubble Sort Overview
71 Bubble Sort Implementation
72 Bubble Sort Optimization
73 Bubble Sort BIG O Complexity
Selection Sort
74 PREREQUISITES
75 Selection Sort Introduction
76 Selection Sort Implementation
77 Selection Sort Big O Complexity
Insertion Sort
78 PREREQUISITES
79 Insertion Sort Introduction
80 Insertion Sort Implementation
81 Insertion Sort BIG O Complexity
Comparing Bubble, Selection, and Insertion Sort
82 Comparing Bubble, Selection, and Insertion Sort
Merge Sort
83 PREREQUISITES
84 Intro to the Crazier Sorts
85 Merge Sort Introduction
86 Merging Arrays Intro
87 Merging Arrays Implementation
88 Writing Merge Sort Part 1
89 Writing Merge Sort Part 2
90 Merge Sort BIG O Complexity
Quick Sort
91 PREREQUISITES
92 Introduction to Quick Sort
93 Pivot Helper Introduction
94 Pivot Helper Implementation
95 Quick Sort Implementation
96 Quick Sort Call Stack Walkthrough
97 Quick Sort Big O Complexity
Radix Sort
98 PREREQUISITES
99 Radix Sort Introduction
100 Radix Sort Helper Methods
101 Radix Sort Pseudocode
102 Radix Sort Implementation
103 Radix Sort BIG O Complexity
Data Structures Introduction
104 Which Data Structure Is The Best
105 ES2015 Class Syntax Overview
106 Data Structures The Class Keyword
107 Data Structures Adding Instance Methods
108 Data Structures Adding Class Methods
Singly Linked Lists
109 PREREQUISITES
110 Intro to Singly Linked Lists
111 Starter Code and Push Intro
112 Singly Linked List Push Solution
113 Singly Linked List Pop Intro
114 Singly Linked List Pop Solution
115 Singly Linked List Shift Intro
116 Singly Linked List Shift Solution
117 Singly Linked List Unshift Intro
118 Singly Linked List Unshift Solution
119 Singly Linked List Get Intro
120 Singly Linked List Get Solution
121 Singly Linked List Set Intro
122 Singly Linked List Set Solution
123 Singly Linked List Insert Intro
124 Singly Linked List Insert Solution
125 Singly Linked List Remove Intro
126 Singly Linked List Remove Solution
127 Singly Linked List Reverse Intro
128 Singly Linked List Reverse Solution
129 Singly Linked List BIG O Complexity
Doubly Linked Lists
130 PREREQUISITES
131 Doubly Linked Lists Introduction
132 Setting Up Our Node Class
133 Push
134 Push Solution
135 Pop
136 Pop Solution
137 Shift
138 Shift Solution
139 Unshift
140 Unshift Solution
141 Get
142 Get Solution
143 Set
144 Set Solution
145 Insert
146 Insert Solution
147 Remove
148 Remove Solution
149 Comparing Singly and Doubly Linked Lists
Stacks + Queues
150 PREREQUISITES
151 Intro to Stacks
152 Creating a Stack with an Array
153 Writing Our Own Stack From Scratch
154 BIG O of Stacks
155 Intro to Queues
156 Creating Queues Using Arrays
157 Writing Our Own Queue From Scratch
158 BIG O of Queues
Binary Search Trees
159 PREREQUISITES
160 Introduction to Trees
161 Uses For Trees
162 Intro to Binary Trees
163 POP QUIZ!
164 Searching A Binary Search Tree
165 Our Tree Classes
166 BST Insert
167 BST Insert Solution
168 BST Find
169 BST Find Solution
170 Big O of Binary Search Trees
Tree Traversal
171 PREREQUISITES
172 Intro To Tree Traversal
173 Breadth First Search Intro
174 Breadth First Search Solution
175 Depth First PreOrder Intro
176 Depth First PreOrder Solution
177 Depth First PostOrder Intro
178 Depth First PostOrder Solution
179 Depth First InOrder Intro
180 Depth First InOrder Solution
181 When to Use BFS and DFS
Binary Heaps
182 PREREQUISITES
183 Intro to Heaps
184 Storing Heaps
185 Heap Insert Intro
186 Heap Insert Solution
187 Heap ExtractMax Intro
188 Heap ExtractMax Solution
189 Priority Queue Intro
190 Priority Queue Pseudocode
191 Priority Queue Solution
192 BIG O of Binary Heaps
Hash Tables
193 PREREQUISITES
194 Intro to Hash Tables
195 More About Hash Tables
196 Intro to Hash Functions
197 Writing Our First Hash Function
198 Improving Our Hash Function
199 Handling Collisions
200 Hash Table Set and Get
201 Hash Table Set Solution
202 Hash Table Get Solution
203 Hash Table Keys and Values
204 Hash Table Keys and Values Solution
205 Hash Table Big O Complexity
Graphs
206 PREREQUISITES
207 Intro to Graphs
208 Uses for Graphs
209 Types of Graphs
210 Storing Graphs Adjacency Matrix
211 Storing Graphs Adjacency List
212 Adjacency Matrix Vs. List BIG O
213 Add Vertex Intro
214 Add Vertex Solution
215 Add Edge Intro
216 Add Edge Solution
217 Remove Edge Intro
218 Remove Edge Solution
219 Remove Vertex Intro
220 Remove Vertex Solution
Graph Traversal
221 PREREQUISITES
222 Intro to Graph Traversal
223 Depth First Graph Traversal
224 DFS Recursive Intro
225 DFS Recursive Solution
226 DFS Iterative Intro
227 DFS Iterative Solution
228 Breadth First Graph Traversal
229 BFS Intro
230 BFS Solution
Dijkstra’s Algorithm!
231 PREREQUISITES
232 Intro to Dijkstra’s and Prerequisites
233 Who was Dijkstra and what is his Algorithm
234 Writing a Weighted Graph
235 Walking through the Algorithm
236 Introducing Our Simple Priority Queue
237 Dijkstra’s Pseudo-Code
238 Implementing Dijkstra’s Algorithm
239 Upgrading the Priority Queue
Dynamic Programming
240 Intro to Dynamic Programming
241 Overlapping Subproblems
242 Optimal Substructure
243 Writing A Recursive Solution
244 Time Complexity of Our Solution
245 The Problem With Our Solution
246 Enter Memoization!
247 Time Complexity of Memoized Solution
248 Tabulation A Bottom Up Approach
The Wild West
249 VERY IMPORTANT NOTE! PLEASE READ!
Resolve the captcha to access the links!