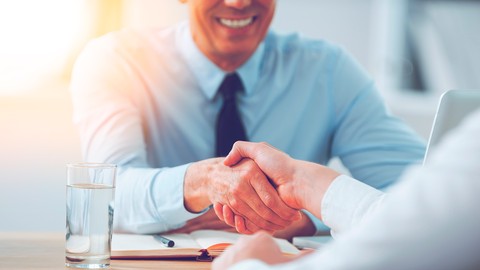
English | MP4 | AVC 1280×720 | AAC 48KHz 2ch | 6 Hours | Lec: 54 | 897 MB
200+ Java Interview Questions for Beginners
Preparing for Java Interview is tricky. You would need to get a good understanding of new features and revise concepts you used in your preparation. This course helps you prepare for Java Interview with hands-on code examples covering 200+ Java Interview Questions and Answers on varied range of topics listed below.
Table of Contents
01 – Introduction
02 – Three Things You need to Know
03 – Course Overview
04 – Java Popularity and Platform Independence
05 – Compare JDK vs JVM vs JRE
06 – Differences between C++ and Java
07 – Java Classloaders
08 – Wrapper Classes
09 – String, StringBuffer and StringBuilder
10 – Object Basics – Class, Object, State and Behavior
11 – toString method
12 – equals and hashCode methods
13 – Inheritance, Method overloading and Method overriding
14 – Interface
15 – Abstract Class
16 – Constructors – this() and super()
17 – Do not forget to do Exercises
18 – Polymorphism and instanceof
19 – What is Coupling
20 – What is Cohesion
21 – What is Encapsulation
22 – Inner Class and Static Inner Class
23 – What is an anonymous class
24 – Access Modifiers – public, private, protected and default
25 – Final method, variable and class
26 – Static variables and methods
27 – Simple Puzzles on conditions and loops
28 – Exception Handling – try, catch and finally
29 – Checked and Unchecked Exceptions
30 – Throwing an Exception
31 – Creating Custom Exceptions
32 – Arrays
33 – Enum
34 – Variable Arguments
35 – Asserts and Garbage Collection
36 – Static and Member Initializers
37 – Serialization
38 – Collection Interface Hierarchy
39 – Collection & List Interface methods and classes – ArrayList, Vector & LinkedList
40 – Set interfaces and implementations – HashSet, LinkedHashSet and TreeSet
41 – Map interfaces and implementations – HashMap, LinkedHashMap and TreeMap
42 – Queue interfaces and implementations – Deque and BlockingQueue
43 – Collection Code Examples
44 – Concurrent Collections – CopyOnWriteArrayList
45 – CompareAndSwap, Locks and AtomicOperations
46 – Generics
47 – MultiThreading – Need for Threads and Creating Threads
48 – Thread states, priority, ExecutorService and Callable
49 – Synchronization of Threads. join, wait, notify and notifyAll methods
50 – Functional Programming Examples – Streams and Lambda Expressions
51 – Functional Programming Questions and Answers
52 – Java New Features – Java 5, 6, 7 and 8
53 – Conclusion
54 – Bonus Lecture Our Best Courses with 66000 Students.html
Resolve the captcha to access the links!