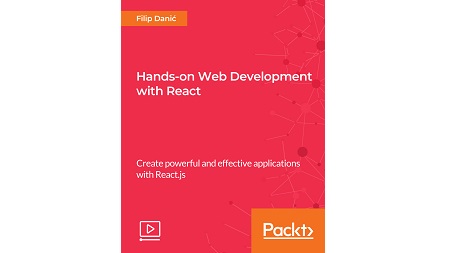
English | MP4 | AVC 1920×1080 | AAC 48KHz 2ch | 4h 25m | 906 MB
Boost your JavaScript skills by building a real-world SPA with React
The best way to explore React is to dive in, build realistic interfaces, and unravel its secrets one by one. This video course is designed to take you on a journey from a fascinated newbie to an expert in advanced concepts and design patterns.
You will work on an SPA project from start to finish and learn how to implement features and interfaces based on real-world requirements. Facing every challenge head-on, you will slowly dissect React and expose its features. Each section will take you through one web development feature with React. You will write components that are maintainable, reusable, stylish (based on CSS-in-JS concepts), and easy to compose. You will tie together all the concepts by turning your small React app into a fully featured Single-Page Application. You will also understand how to add routing, networking, and session management to your project.
By the end of the course, you will be well on your way to becoming a React expert, armed with strong fundamentals and clear conceptual knowledge of advanced patterns.
Through a series of videos, we explore React by diving in and building UI components and implementing realistic features. There is no need to get stuck explaining concepts before they’re needed; we will unravel the complexity behind React (and its supporting tools) only when we need them.
This step-by-step process allows you to follow along with full commitment. As you build your own app alongside these videos, you will enjoy a feeling of accomplishment and insight with every new trick you gradually add to your skill set.
What You Will Learn
- The secret behind crafting reusable UI components with React
- Everything you need to know about the inner workings of React
- Core concepts you’ll need to build complex interfaces—such as lifecycle methods, PropTypes, event handling, state and props, and reconciliation
- Create beautiful interfaces with styled components
- Create an SPA based on real-world requirements
- Leverage functional programming concepts to build composable components
- Simple, yet powerful methods for testing components with Jest
- Various tips and tricks in the field of professional front-end engineering
Table of Contents
Get a Head Start By Creating a React App
1 The Course Overview
2 What Is React Anyway
3 Using Package Managers and the Command Line
4 Your First React App
5 Understanding the Basis of the CRA Magic
6 React, JSX, and Your First Component
Building Components with React
7 Creating a Reusable List Component
8 Forms, Inputs, and Handling Events
9 Understanding Local State in React
10 Validating User Input
11 Examining Code with React Developer Tools
Understanding the Core Concepts
12 Understanding Lifecycle Methods by Example
13 Prop Drilling and Lifting State
14 Type Checking with PropTypes
15 Reconciliation and the Virtual DOM
16 Functional (Stateless) Components
Crafting Styled Components
17 Styling React Components with Good Ol‘ CSS
18 Why is CSS-in-JS Growing in Popularity
19 Getting Started with Styled Components
20 Modern Layouts with Flexbox
21 Working with Animations
22 Building Dynamic Themes
Building a Full React SPA
23 Routing with React Router
24 Working with an API
25 Be Offline-Ready with Mocked APIs
26 Adding a Login System to Our App
27 Session Management
Multi-Role SPAs with React
28 Adding Public Content
29 Handling Different User Roles in Our System
30 Not Just Routes – Granular Feature Control!
31 Working with Third-Party Libraries
32 Session Management
Advanced Patterns and Concepts
33 Functional Programming – You’re Already Doing It!
34 Higher Order Components
35 It’s Just Functions!
36 Simple Component Testing with Jest
37 Safeguard Your App with Snapshot Tests
38 Where to Go From Here
Resolve the captcha to access the links!