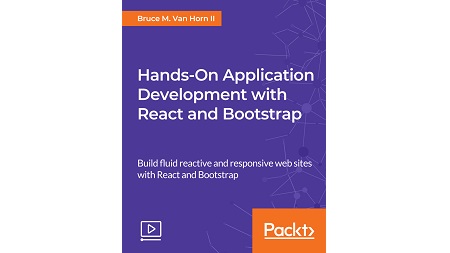
English | MP4 | AVC 1920×1080 | AAC 48KHz 2ch | 6h 53m | 1.62 GB
Fast frontend development with React and Bootstrap; build fluid reactive and responsive websites
React is one of the most popular front-end JavaScript library for interactive web applications. Bootstrap 4 is a free HTML, CSS, and JavaScript framework that allows developers to build responsive, mobile-first websites. Integrating Bootstrap with React allows web developers to write much cleaner code, thus reducing the time spent on the frontend. In this course, the author, Bruce Van Horn, will help you gain a thorough understanding of the Bootstrap framework and will show you how to build impressive web apps. You will build a website with UI elements such as image galleries and custom pricing/shopping tools along with Bruce. He will show you how to use HTML, ES6, CSS, React, and Bootstrap 4 to build your own dynamic website.
By the end of the course, you will be able to build real-time responsive web apps using React and Bootstrap and will have learned to use the ES6 Syntax.
This course will step you through creating a full site using HTML, JavaScript (ES6), CSS, React, and Bootstrap 4. When we’re done, you will have a project that showcases the most popular component in the Bootstrap 4 library. You will learn to actually code and work on projects rather than just see commands in a REPL.
What You Will Learn
- Install the required dependencies for a React App that uses Bootstrap 4
- Use JSX to code your markup in a very natural way (as opposed to the usual approach)
- Create your own React user login and registration component containing elements from the Bootstrap 4 library
- Build a user interface out of components instead of the traditional jQuery and Document Object Model
- Work with an integrated testing server running on Node.js to create a production build
- Publish your web application using a production build script
Table of Contents
Getting Started With Create-React-App
1 Course Overview
2 Prerequisites and Software
3 Exercise Files
4 Previewing the Finished App
Laying the Foundation Building Out the Navigation
5 Install and Run Create-React-App
6 Introduction to JSON Server
7 Using Faker to Generate Random Test Data
8 React Component State
9 React’s Component Life Cycle
10 Creating a React Component with ES6
11 Fetching Data with Axios
12 Testing Our Code
13 Reviewing the Site’s Structure
14 Adding the Navbar Placeholder
15 Stubbing the Home Component
16 Stubbing the Image Carousel
17 Stubbing the Vehicle Browser
18 Creating the Footer
19 Introduction to React Router
20 Working with React Router
Creating Your Own React ES6 Components
21 Reactstrap Overview
22 Adding a ReactstrapNavbar
23 Changing the Navbar Sample Code
24 Adding Font Awesome
25 Introduction to React Properties
26 Making the Navbar Data-Driven
27 Adding an Image Carousel
28 Copying the Asset Files
29 Using React Router with Properties
30 Data-Driven Site Carousel
31 Using Layout Components to Build the Vehicle Browser
32 Making the Vehicle Browser Data-Driven
UX Made Easy with Nested Components
33 Forms versus Input Groups
34 Stubbing the Dealer Locator Component
35 Working with Input Groups
36 Input Group Events Handlers
37 The Table Component
38 Working with Lists
39 List Items with Badges
40 Adding an Inquiry Form – Part 1
41 Adding an Inquiry Form – Part 2
42 Posting with Axios –Part 1
43 Posting with Axios –Part 2
Building and Publishing Your ES6 React App with Babel
44 Create the Vehicle Detail Component – Part 1
45 Create the Vehicle Detail Component – Part 2
46 The Build and Price Component (Tabs) – Part 1
47 The Build and Price Component (Tabs) – Part 2
48 Collapsing Media Container – Part 1
49 Collapsing Media Container – Part 2
50 Pick a Model – Part 1
51 Pick a Model – Part 2
52 Color Picker
53 Engine Picker Dropdown – Part 1
54 Engine Picker Dropdown –Part 2
55 Modals
56 Cleaning Up Warnings
57 Production Build and Conclusion
Resolve the captcha to access the links!