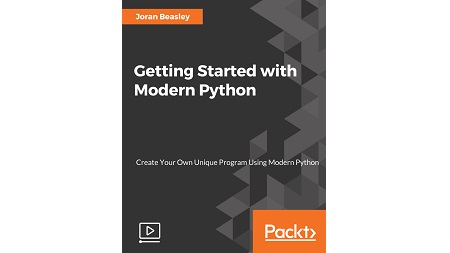
English | MP4 | AVC 1920×1080 | AAC 48KHz 2ch | 3h 16m | 550 MB
Learn Python like a Professional! Start from the basics and go all the way to create your program in it.
In this course, you will start by setting up your development environment, including downloading Python and setting up your IDE (PyCharm); you’ll then be introduced to Python lists and list comprehensions. The course will then show you what a generator is and why you might want to use one. Further on, you will be introduced to functions and decorators, and see how you can use them. You will then learn how to create a very simple, single-file Python program, and how to execute it both from the command line and from within the IDE. You’ll also get a very brief introduction to debugging.
By the end of the course, you’ll have learned how to manipulate strings, parsing and printing them.
This practical course takes you through the tools Modern Python has to offer for your environment. It is packed with step-by-step instructions and working examples. This comprehensive course is divided into clear bite-size chunks so you can learn at your own pace and focus on the areas of most interest to you.
What You Will Learn
- Set up your development environment in Python
- Run and execute Python both from the command line and from within the IDE
- Run Python from inside PyCharm
- Install and manage different Python versions
- Write and debug your own Python programs
- Work with strings and file-like interfaces
- Harness the power of list comprehensions
- Explore generators and itertools and get an introduction to creating your own functions classes and modules
Table of Contents
Getting Started with Python Development
1 The Course Overview
2 Setting Up Your Development Environment
3 Writing Your First Python Program
4 Writing Your Second Python Program
5 Debugging Your Second Python Program
Working with Strings
6 Printing and Formatting Strings
7 Parsing Simple Strings
8 Working with the Re Module
9 Working with Dates
10 Ensuring Data Integrity and Security
11 Working with Files, and File-like Interfaces
Harnessing the Power of List Comprehensions
12 Introduction to Lists and for Loops
13 Working with List Comprehensions
14 Filtering Data in List Comprehensions
Generators and Itertools
15 Some Problems with List Comprehensions
16 Working with Generators
17 Going to Infinity and Beyond with Itertools
Functions, Because Sometimes One Line Isn’t Enough
18 Introduction to Functions
19 Various Concepts of Functions
20 Exploring Function Decorators
21 Document Code and Verify Code Correctness
22 Classes, Modules – When and Why to Use Them
Resolve the captcha to access the links!