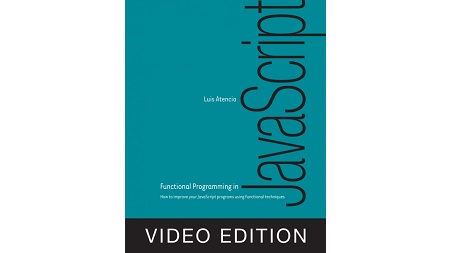
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 6h 37m | 1.09 GB
Functional Programming in JavaScript teaches you techniques to improve your web applications: their extensibility, modularity, reusability, and testability, as well as their performance. This easy-to-read book/course uses concrete examples and clear explanations to show you how to use functional programming in real life. If you’re new to functional programming, you’ll appreciate this guide’s many insightful comparisons to imperative or object-oriented programming that help you understand functional design. By the end, you’ll think about application design in a fresh new way, and you may even grow to appreciate monads!
In complex web applications, the low-level details of your JavaScript code can obscure the workings of the system as a whole. As a coding style, functional programming (FP) promotes loosely coupled relationships among the components of your application, making the big picture easier to design, communicate, and maintain.
Inside:
- High-value FP techniques for real-world uses
- Using FP where it makes the most sense
- Separating the logic of your system from implementation details
- FP-style error handling, testing, and debugging
- All code samples use JavaScript ES6 (ES 2015)
Created for developers with a solid grasp of JavaScript fundamentals and web application design.
Table of Contents
01 Becoming functional
02 What is functional programming
03 Pure functions and the problem with side effects
04 Referential transparency and substitutability
05 Benefits of functional programming
06 Reacting to the complexity of asynchronous applications
07 Higher-order JavaScript
08 Functional vs. object-oriented programming
09 Managing the state of JavaScript objects
10 Deep-freezing moving parts
11 Functions
12 Types of function invocation
13 Closures and scopes
14 JavaScript’s function scope
15 Practical applications of closures
16 Few data structures, many operations
17 Understanding lambda expressions
18 Gathering results with _.reduce
19 Reasoning about your code
20 SQL-like data – functions as data
21 Learning to think recursively
22 Recursively defined data structures
23 Toward modular, reusable code
24 Requirements for compatible functions
25 Curried function evaluation
26 Partial application and parameter binding
27 Composing function pipelines
28 Composition with functional libraries
29 Managing control flow with functional combinators
30 Fork (join) combinator
31 Design patterns against complexity
32 Building a better solution – functors
33 Functors explained
34 Functional error handling using monads
35 Error handling with Maybe and Either monads
36 Interacting with external resources using the IO monad
37 Monadic chains and compositions
38 Bulletproofing your code
39 Challenges of testing imperative programs
40 Testing functional code
41 Separating the pure from the impure with monadic isolation
42 Capturing specifications with property-based testing
43 Measuring effectiveness through code coverage
44 Measuring the complexity of functional code
45 Functional optimizations
46 Currying and the function context stack
47 Deferring execution using lazy evaluation
48 Implementing a call-when-needed strategy
49 Taking advantage of currying and memoization
50 Recursion and tail-call optimization (TCO)
51 Managing asynchronous events and data
52 Falling into a callback pyramid
53 First-class asynchronous behavior with promises
54 Future method chains
55 Lazy data generation
56 Functional and reactive programming with RxJS
57 RxJS and promises
Resolve the captcha to access the links!