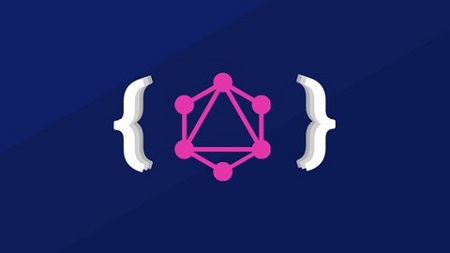
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 30 Hours | 12.5 GB
So you want to finally learn GraphQL and use it with your React apps ?. This is it.
So you want to finally learn GraphQL and use it with your React apps ?. This is the course for you.
We will start from the very beginning, from “I don’t even know what GraphQL is ….and why should use it ?“ to actually understand how GraphQL works and make it work with your server and client.
You will learn all the logic and you will practice GraphQL in different modules.
To sum everything up, you will learn in this course:
- What is GraphQL and why use it
- Understand the tools to make queries and mutations
- Make it work on a server with Express or Apollo server
- Link your GraphQL server to a real DB like MONGO.
- Make it communicate with a client like REACT
- Put you new knowledge to the test by creating an app.
Don’t know how to use React.? Don’t panic, at the end of the course you get a full React mini course, so you get two courses for the price of one.
What you’ll learn
- GraphQL + React. But mainly GraphQL
Table of Contents
Introduction
1 Introduction
2 Installing NODE
3 IDE
4 GITHUB CODE
The basics
5 How we will learn GraphQL
6 The idea behind it
7 Starting with GraphQL + Yoga
8 Scalar types
9 Other types
10 Using json server
11 Arguments and arrays part one
12 Arguments and arrays part two
13 Relationships part one
14 Relationships part two
15 Relationships part three
16 A bit of structure
17 Mutations
18 Mutations – removing posts
19 Mutations – removing users
20 Mutations – Updating
21 Input types
22 Enum types
23 Union types
24 Interfaces
25 Many and alias
26 Fragments
27 Operation name and variables
28 Directives
29 Playground features
MONGO + Express + Apollo + React
30 Creating server and client
31 GraphQL and express
32 MongoDB
33 Making GraphQL work with Mongo
34 Queries from React
35 Apollo server and Apollo server Express
Taking the logic to practice
36 INTRO and installing
37 SERVER Mongo
38 SERVER Sign in Up mutations
39 SERVER Sign up users part one
40 SERVER Sign up users part two
41 SERVER Sign in user
42 SERVER User by ID and Middleware
43 SERVER User by ID and Middleware part two
44 SERVER Auth user and middleware update
45 SERVER Update name and lastname
46 SERVER Update email and password
47 SERVER Create post model and mutation
48 SERVER Category model and mutation
49 SERVER Relationships part one
50 SERVER Relationships part two
51 SERVER Getting posts
52 SERVER Reusing the SORT input
53 SERVER Update post and delete post
54 SERVER Updating and deleting categories
55 CLIENT Setup
56 CLIENT Header and sign in routes
57 CLIENT Sign in register form
58 CLIENT Register users
59 CLIENT Register users 2
60 CLIENT Sign in user
61 CLIENT Auto sign in
62 CLIENT Logout user
63 CLIENT Creating the user area
64 CLIENT Guarding routes
65 CLIENT Updating email and pass
66 CLIENT Getting user stats
67 CLIENT User stats layout
68 CLIENT Adding post
69 CLIENT Adding post 2
70 CLIENT User articles
71 CLIENT User articles 2
72 CLIENT Updating post status
73 CLIENT Remove post
74 CLIENT Fetching home posts
75 CLIENT Home layout
76 CLIENT Creating the article view
77 CLIENT Finishing the article view
Apollo client [OPTIONAL]
78 The template
79 Starting with Apollo client
80 Getting data
81 Getting data on demand
82 Polling and refetching
83 Network changes
84 Mutations 1
85 Mutations 2
86 More to come.
REACT MINI [ OPTIONAL ]
87 Installing node and using a CLI
88 The bundle
89 Starting with the code
90 JSX Behind the scenes
91 Importing components
92 Types of components
93 Adding styles
94 Events
95 The STATE
96 Using props
97 Using props 2
98 Props to class
99 Childrens
100 More with styles
101 Styles plugins
102 Filter the news
103 ROUTES installing
104 ROUTES how they work
105 ROUTESUsing them
106 ROUTES Linking
107 ROUTES Working with params
108 ROUTESOther features
109 ROUTESSwitch
110 ROUTESRedirections
111 ROUTES404 and WITHROUTER
112 PRACTICE 1 Install
113 PRACTICE 1 Adding a header
114 PRACTICE 1 Home list
115 PRACTICE 1 Artists view
116 Component lifecycle
117 Component lifecycle 2
118 Conditional rendering
119 Pure components
120 Adjacent elements
121 HOC’s
122 HOC’s continued
123 Transitions
124 Transitions continued
125 CSS Transitions
126 Transitions group
127 Proptypes
128 Proptypes 2
129 Controlles component
130 PRACTICE.PROJ1 Installation
131 PRACTICE.PROJ1 Routes,Footer and header
132 PRACTICE.PROJ1 Adding a slider
133 PRACTICE.PROJ1Adding subs
134 PRACTICE.PROJ1 Adding subs …continued
135 PRACTICE.PROJ1 Home blocks
136 PRACTICE.PROJ1 Adding a poll
137 PRACTICE.PROJ1 Finishing a poll
138 PRACTICE.PROJ1 Adding the teams section
139 PRACTICE.PROJ1 Finishing the teams section
140 PRACTICE.PROJ1 Creating the team view
141 REDUX intro
142 REDUX Creating a store
143 REDUX The redux flow
144 REDUX Combining reducers
145 REDUX Creating a valid reducer
146 REDUX Creating an action
147 REDUX Mapstate to props and connect
148 REDUX Dispatch
149 REDUX Types
150 REDUX Using middleware
151 PRACTICE.PROJ2 Installations
152 PRACTICE.PROJ2 Adding redux
153 PRACTICE.PROJ2 Home data
154 PRACTICE.PROJ2 Latest news
155 PRACTICE.PROJ2 Other news
156 PRACTICE.PROJ2 Article page
157 PRACTICE.PROJ2 Adding likes
158 PRACTICE.PROJ2 Finishing likes
159 PRACTICE.PROJ2 Clearing data
160 HOOKS introduction
161 HOOKS UseState
162 HOOKS UseState 2
163 HOOKS UseEffect
164 HOOKS UseReducer
165 HOOKS The context API
166 HOOKS Context and useContext
Resolve the captcha to access the links!