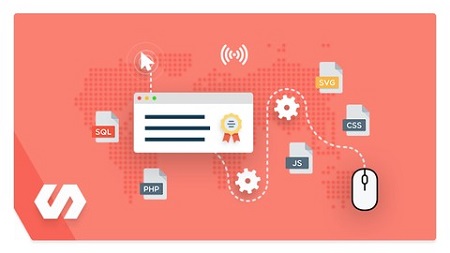
English | MP4 | AVC 1280×720 | AAC 48KHz 2ch | 21.5 Hours | 11.7 GB
Build, test, and deploy Docker applications with Kubernetes while learning production-style development workflows
If you’re tired of spinning your wheels learning how to deploy web applications, this is the course for you.
CI+CD Workflows? You will learn it. AWS Deployment? Included. Kubernetes in Production? Of course!
This is the ultimate course to learn how to deploy any web application you can possibly dream up. Docker and Kubernetes are the newest tech in the Dev Ops world, and have dramatically changed the flow of creating and deploying web apps. Docker is a technology that allows applications to run in constructs called ‘containers’, while Kubernetes allows for many different ‘containers’ to run in coordination.
Docker from Scratch!
In this course you’ll learn Docker from absolute fundamentals, beginning by learning the answer to basic questions such as “What is a container?” and “How does a container work?”. From the very first few lectures, we will do a deep dive on the inner workings of containers, so you get a core understanding of exactly how they are implemented. Once you understand what a container is, you’ll learn how to work with them using basic Docker CLI commands. After that, you’ll apply your new-found mastery of the Docker CLI to build your own custom images, effectively ‘Dockerizing’ your own personal applications.
CI + CD Pipelines
Of course, no course on Docker would be complete without a full understanding of common Continuous Integration and Continuous Deployment patterns. You will learn how to implement a full CI + CD workflow using Github, Travis CI, and Amazon Web Services, creating a pipeline that automatically deploys your code every time you push your latest changes to Github!
Multi-Container Deployments on AWS!
After building a deployment pipeline, you’ll apply it to master both single-container and multi-container deployments on Amazon Web Services. You will construct a multi-container application utilizing Node, React, Redis, and Postgres, and see the amazing power of containers in action (Note: all Javascript coding in this course is optional, the full source code is provided if you don’t want to write JS).
Kubernetes!
Finally, you will tackle Kubernetes, a production-grade system for managing complex applications with many different running containers. You will learn the right way to build a Kubernetes Cluster – this course doesn’t have any of those annoying “don’t do this in production” comments! You will first build a Kubernetes Cluster on your local machine, then eventually move it over to a cloud provider. You’ll even learn how to set up HTTPS on Kubernetes, which is harder than it sounds!
Here’s what you’ll do:
- Learn Docker from scratch, no previous experience required
- Build your own custom images tailored to your applications
- Master the Docker CLI to inspect and debug running containers
- Understand how Docker works behind the scenes, and what a container is
- Build a CI + CD pipeline from scratch with Github, Travis CI, and AWS
- Automatically deploy your code when it is pushed to Github!
- Build a complex multi-container application from scratch and deploy it to AWS
- Understand the purpose and theory of Kubernetes
- Deploy a production-ready Kubernetes Cluster to Google Cloud
Table of Contents
Dive Into Docker
1 Finished Code and Diagrams
2 Why Use Docker
3 What is Docker
4 Docker for MacWindows
5 Installing Docker on MacOS
6 Installing Docker for Windows Home users
7 Installing Docker for Windows Profressional
8 More Windows Professional Setup
9 One Last Piece of Windows Professional Setup
10 Installing Docker on Linux
11 Using the Docker Client
12 But Really…Whats a Container
13 Hows Docker Running on Your Computer
Manipulating Containers with the Docker Client
14 Docker Run in Detail
15 Overriding Default Commands
16 Listing Running Containers
17 Container Lifecycle
18 Restarting Stopped Containers
19 Removing Stopped Containers
20 Retrieving Log Outputs
21 Stopping Containers
22 Multi-Command Containers
23 Executing Commands in Running Containers
24 The Purpose of the IT Flag
25 Getting a Command Prompt in a Container
26 Starting with a Shell
27 Container Isolation
Building Custom Images Through Docker Server
28 Creating Docker Images
29 Buildkit for Docker Desktop v2.4.0 and Edge
30 Building a Dockerfile
31 Dockerfile Teardown
32 Whats a Base Image
33 The Build Process in Detail
34 A Brief Recap
35 Rebuilds with Cache
36 Tagging an Image
37 Quick Note for Windows Users
38 Manual Image Generation with Docker Commit
Making Real Projects with Docker
39 Project Outline
40 Node Server Setup
41 A Few Planned Errors
42 Resolving npm ERR could not detect node name from path or package
43 Base Image Issues
44 A Few Missing Files
45 Copying Build Files
46 Reminder for Windows Home Docker Toolbox Students
47 Container Port Mapping
48 Specifying a Working Directory
49 Unnecessary Rebuilds
50 Minimizing Cache Busting and Rebuilds
Docker Compose with Multiple Local Containers
51 App Overview
52 App Server Starter Code
53 Assembling a Dockerfile
54 Introducing Docker Compose
55 Docker Compose Files
56 Networking with Docker Compose
57 Docker Compose Commands
58 Stopping Docker Compose Containers
59 Container Maintenance with Compose
60 Automatic Container Restarts
61 Container Status with Docker Compose
Creating a Production-Grade Workflow
62 Development Workflow
63 Flow Specifics
64 Dockers Purpose
65 Project Generation
66 Create React App Generation
67 More on Project Generation
68 Necessary Commands
69 Creating the Dev Dockerfile
70 Duplicating Dependencies
71 React App Exits Immediately with Docker Run Command
72 Starting the Container
73 Docker Volumes
74 Windows not Detecting Changes – Update
75 Bookmarking Volumes
76 React App Exited With Code 0
77 Shorthand with Docker Compose
78 Overriding Dockerfile Selection
79 Windows not Detecting Changes – Docker Compose
80 Do We Need Copy
81 Executing Tests
82 Live Updating Tests
83 Docker Compose for Running Tests
84 Tests Not Re-running on Windows
85 Attaching to Web container
86 Shortcomings on Testing
87 Need for Nginx
88 Multi-Step Docker Builds
89 Named Builders and AWS
90 Implementing Multi-Step Builds
91 Running Nginx
Continuous Integration and Deployment with AWS
92 Services Overview
93 Github Setup
94 Travis CI Setup
95 Travis YML File Configuration
96 Required Travis Updates
97 A Touch More Travis Setup
98 Automatic Build Creation
99 Important info about AWS Platform Versions
100 AWS Elastic Beanstalk
101 More on Elastic Beanstalk
102 Travis Config for Deployment
103 Travis Keys Update
104 Automated Deployments
105 Exposing Ports Through the Dockerfile
106 AWS Build Still Failing
107 Workflow With Github
108 Redeploy on Pull Request Merge
109 Deployment Wrapup
110 Environment Cleanup
111 AWS Configuration Cheat Sheet
112 Finished Project Code with Updates Applied
Building a Multi-Container Application
113 Single Container Deployment Issues
114 Application Overview
115 A Quick Note
116 Application Architecture
117 Worker Process Setup
118 Important note about PG module version
119 Express API Setup
120 Important Update for Table Query
121 Connecting to Postgres
122 More Express API Setup
123 Create React App Generation
124 Generating the React App
125 Fetching Data in the React App
126 Rendering Logic in the App
127 Exporting the Fib Class
128 Routing in the React App
Dockerizing Multiple Services
129 Checkpoint Files
130 Checkpoint Catchup
131 Reminder on -it flag
132 Dockerizing a React App – Again
133 Important Node Image Version Update
134 Dockerizing Generic Node Apps
135 Adding Postgres as a Service
136 Docker-compose Config
137 Postgres Database Required Fixes and Updates
138 Environment Variables with Docker Compose
139 Required Client Service Update and Worker Environment Variables
140 The Worker and Client Services
141 Nginx Path Routing
142 Routing with Nginx
143 Building a Custom Nginx Image
144 Starting Up Docker Compose
145 Nginx connect() failed – Connection refused while connecting to upstream
146 Troubleshooting Startup Bugs
147 Opening Websocket Connections
A Continuous Integration Workflow for Multiple Images
148 Production Multi-Container Deployments
149 Important Node Image Version Update
150 Production Dockerfiles
151 Multiple Nginx Instances
152 Nginx fix for React Router
153 Altering Nginxs Listen Port
154 Cleaning Up Tests
155 Github and Travis CI Setup
156 Fix for Failing Travis Builds
157 Travis Configuration Setup
158 Pushing Images to Docker Hub
159 Successful Image Building
Multi-Container Deployments to AWS
160 Multi-Container Definition Files
161 Finding Docs on Container Definitions
162 Adding Container Definitions to DockerRun
163 More Container Definitions
164 Forming Container Links
165 AWS Configuration Cheat Sheet – Updated for new UI
166 Creating the EB Environment
167 Managed Data Service Providers
168 Overview of AWS VPCs and Security Groups
169 RDS Database Creation
170 ElastiCache Redis Creation
171 Creating a Custom Security Group
172 Applying Security Groups to Resources
173 Setting Environment Variables
174 IAM Keys for Deployment
175 Travis Keys Update
176 Travis Deploy Script
177 Container Memory Allocations
178 Verifying Deployment
179 A Quick App Change
180 Making Changes
181 Cleaning Up AWS Resources
182 AWS Configuration Cheat Sheet
183 Finished Project Code with Updates Applied
Onwards to Kubernetes
184 The Whys and Whats of Kubernetes
185 Kubernetes in Development and Production
186 Updated Minikube Install and Setup Info – macOS
187 Minikube Setup on MacOS
188 Minikube Setup on Windows Pro
189 Minikube Setup on Windows Home
190 Minikube Setup on Linux
191 Docker Desktops Kubernetes instead of Minikube
192 Mapping Existing Knowledge
193 Quick Note to Prevent an Error
194 Adding Configuration Files
195 Object Types and API Versions
196 Running Containers in Pods
197 Service Config Files in Depth
198 Connecting to Running Containers
199 The Entire Deployment Flow
200 Imperative vs Declarative Deployments
Maintaining Sets of Containers with Deployments
201 Updating Existing Objects
202 Declarative Updates in Action
203 Limitations in Config Updates
204 Running Containers with Deployments
205 Quick Note to Prevent an Error
206 Deployment Configuration Files
207 Walking Through the Deployment Config
208 Applying a Deployment
209 Why Use Services
210 Scaling and Changing Deployments
211 Updating Deployment Images
212 Rebuilding the Client Image
213 Triggering Deployment Updates
214 Imperatively Updating a Deployments Image
215 Reminder for Docker Desktops Kubernetes Users
216 Multiple Docker Installations
217 Reconfiguring Docker CLI
218 Why Mess with Docker in the Node
A Multi-Container App with Kubernetes
219 The Path to Production
220 Checkpoint Files
221 A Quick Checkpoint
222 Recreating the Deployment
223 NodePort vs ClusterIP Services
224 The ClusterIP Config
225 Applying Multiple Files with Kubectl
226 Express API Deployment Config
227 Cluster IP for the Express API
228 Combining Config Into Single Files
229 The Worker Deployment
230 Reapplying a Batch of Config Files
231 Creating and Applying Redis Config
232 Important Note about Expected Postgres Error
233 Last Set of Boring Config
234 The Need for Volumes with Databases
235 Kubernetes Volumes
236 Volumes vs Persistent Volumes
237 Persistent Volumes vs Persistent Volume Claims
238 Claim Config Files
239 Persistent Volume Access Modes
240 Where Does Kubernetes Allocate Persistent Volumes
241 Designating a PVC in a Pod Template
242 Applying a PVC
243 Defining Environment Variables
244 Adding Environment Variables to Config
245 Creating an Encoded Secret
246 Postgres Environment Variable Fix
247 Passing Secrets as Environment Variables
248 Environment Variables as Strings
Handling Traffic with Ingress Controllers
249 Load Balancer Services
250 A Quick Note on Ingresses
251 One Other Quick Note
252 Behind the Scenes of Ingress
253 More Behind the Scenes of Ingress
254 Optional Reading on Ingress Nginx
255 Docker Driver and Ingress – IMPORTANT
256 Update on Mandatory Commands
257 Setting up Ingress Locally with Minikube
258 Setting up Ingress with Docker Desktops Kubernetes
259 Ingress Update this.state.seenIndexes.map is not a function 404 errors
260 Creating the Ingress Configuration
261 Testing Ingress Locally
262 Indexes I Have Seen is Empty
263 The Minikube Dashboard
264 Docker Desktops Kubernetes Dashboard
Kubernetes Production Deployment
265 The Deployment Process
266 Google Cloud vs AWS for Kubernetes
267 Creating a Git Repo
268 Linking the Github Repo to Travis
269 Free Google Cloud Credits
270 Creating a Google Cloud Project
271 Linking a Billing Account
272 Kubernetes Engine Init
273 Creating a Cluster with Google Cloud
274 Dont Forget to Cleanup
275 Kubernetes Dashboard on Google Cloud
276 Travis Deployment Overview
277 Installing the Google Cloud SDK
278 Generating a Service Account
279 Ruby Version Fix
280 Running Travis CLI in a Container
281 Fixes for Travis iv undefined orrepository not known
282 Encrypting a Service Account File
283 More Google Cloud CLI Config
284 Fix For Failing Travis Builds
285 Running Tests with Travis
286 Custom Deployment Providers
287 Unique Deployment Images
288 Unique Tags for Built Images
289 Updating the Deployment Script
290 Configuring the GCloud CLI on Cloud Console
291 Creating a Secret on Google Cloud
292 Helm v3 Update
293 Helm v2 Update
294 Helm Setup
295 Kubernetes Security with RBAC
296 Assigning Tiller a Service Account
297 Ingress-Nginx with Helm
298 The Result of Ingress-Nginx
299 Finally – Deployment
300 Did I Really Type That
301 Verifying Deployment
302 A Workflow for Changing in Prod
303 Merging a PR for Deployment
304 Thats It Whats Next
HTTPS Setup with Kubernetes
305 HTTPS Setup Overview
306 Domain Purchase
307 Domain Name Setup
308 Fix for Cert Manager Breaking Changes
309 Cert Manager Install
310 How to Wire Up Cert Manager
311 Issuer Config File
312 Certificate Config File
313 Deploying Changes
314 Verifying the Certificate
315 Ingress Config for HTTPS
316 It Worked
317 Google Cloud Cleanup
318 Local Environment Cleanup
Local Development with Skaffold
319 Awkward Local Development
320 Installing Skaffold
321 The Skaffold Config File
322 Live Sync Changes
323 Automatic Shutdown
324 Testing Live Sync with the API Server
Extras
325 Bonus
Resolve the captcha to access the links!