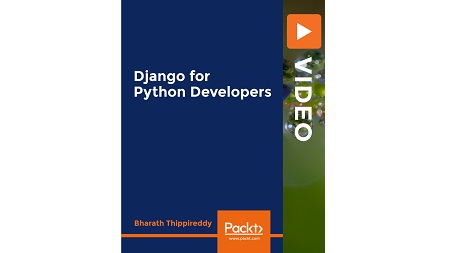
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 8h 46m | 5.23 GB
Master Django and create Python web applications in simple steps
Are you a Python developer, and do you want to create Python web applications by mastering Django? Are you an experienced Django developer and do you want to fill in any gaps in your knowledge about creating a web application using Django? If so, this course is for you too?
Django is the most widely used web application development framework in the industry today. Django makes it super-easy to create production-ready web applications. You will start this course by learning what Django is, and you’ll get to know the different features that are a part of every Django application. You will be working (hands-on) with one feature at a time. You will then create a web application using all the knowledge you’ve gained.
Learn
- Learn the fundamentals of web application development
- Understand how Django makes it easy to build web apps
- Master the Model View template pattern that Django uses
- See Django in action
- Create Django Views to process a user request and send a response
- Implement templates and use them in views
- Create models and use Django migrations to create database tables from models
- Master the fundamentals of Django Object Relational Mapping (ORM), which makes it super-easy to work with databases without writing any SQL
- Use Django forms to collect and process data while learning how to use the inbuilt validators (and create custom validators)
Table of Contents
Introduction
1 Course and Instructor Introduction
2 How to use this course
The Basics
3 Web Application Basics
4 Server-Side Programming
5 What and Why Django
6 MVT Pattern
7 Django Projects and Applications
Software Setup
8 Install Django
9 Install ATOM IDE
10 Download MySql and MySql Workbench
11 Launch MySql workbench
12 Install python mysqlclient
13 Completed projects for download
First Django Web Application
14 Create a DJango Project
15 Run the project
16 Create a DJango App
17 Create a View
18 Configure URLs and Run the app
19 Create another view
20 Multiple applications in a project
21 Application level URLs
Templates
22 Introduction
23 Hands on Steps
24 Create the Project with Template Settings
25 Create a Template and View
26 Configure the URL and TEST
27 Template Tags
28 Using template tags
29 Rendering Employee Information
30 Using Static Files
31 Insert an Image
32 Use a CSS
33 Create Product Templates Project
34 Create the templates
35 Create the Views
36 Map the URLs and Test
Models
37 Introduction
38 Hands on Steps
39 Create a Project
40 Using MySql Database
41 Create the Model
42 Converting Model to DB Tables
43 Use the model in view
44 Create the template
45 Run and Test
46 Django Admin UI
47 Adding Model to the Admin UI
48 Displaying Model fields on the UI
49 Using SqlLite DB
Django ORM
50 The Fundamentals
51 Filtering Data
52 Using Logical Operators
53 Selective Columns
54 Aggregate Functions
55 Create
56 Bulk Create
57 Delete
58 Update
59 Order By
Forms
60 Introduction
61 Hands on Steps
62 Create the Project
63 Create the Form
64 CSRF Token
65 Use the form in the View
66 Create the template
67 Configure the CSRF Token and URLs
68 Forms in action
69 Processing the form data
70 Different Types of form fields
71 Default Django Validations
72 Writing Custom Clean Methods
73 Single clean method
74 In-Built Validators
Model Forms
75 Introduction
76 Hands on Steps
77 Create the project and model
78 Create the Model Form
79 Create the Views
80 Create the Add Project View
81 Create the Index Template
82 Create the List Projects Template
83 Create the Add Project Template
84 Configure URLs
85 Configure MYSQL
86 Run Migrations
87 Refactor and Run the Application
88 Django tables from migrate
CRUD Using Function Based Views
89 Using DJango form for Update
90 Hands on Steps
91 Create the Project
92 Implement READ
93 Run the Migrations
94 Test READ
95 Implement Create
96 Test Create
97 Delete
98 Update View
99 Update Template
100 Test Update
Class Based Views
101 CBV in Action
102 Setting Attributes on a CBV
103 Hands on Steps
104 Create Project
105 Create List View
106 Create List Template
107 Test List View
108 Implement Student Details
109 Create
110 Test Create
111 Update
112 Delete
More about Templates
113 Use Template Inheritance on CBV
114 Filters in Action
115 User Defined Filters
116 Using Custom Filter
117 Registering a Filter using a decorator
118 Passing Arguments
Session Management
119 Statelessness of HTTP
120 Session Management
121 Django Session Management
122 Create the Project
123 Checking Cookie Support
124 Run the Test
125 Page Hit Count using Cookies
126 Cookies in action
127 Shopping Cart – Create Form and Views
128 Shopping Cart – Create the Add Item View
129 Shopping Cart – Implement Templates
130 Shopping Cart – Display Items Template
131 Shopping Cart – Configure the URLs and Run
132 Create Session API Project
133 Page Count Using Session API
134 Implement Shopping Cart
135 Configure URLs and Test
136 Other methods on session
137 Introduction
Middleware
138 Introduction
139 Custom Middleware
140 Create Custom Middleware
141 Exception handling middleware
Security
142 Introduction
143 Steps
144 The first two steps
145 Secure the views
146 Create Users
147 Test
148 Logout redirect
149 Add login and logout links
150 Implement Authorization
151 User Groups
ORM Relationships
152 Introduction
153 Implement ManyToMany
154 ManyToMany in action
155 Implement ManyToOne
156 ManyToOne in action
157 Implement OneToOne
158 OneToOne In Action
Clinical Data Reporting Project
159 Usecase
160 Project Workflow
161 Create Project
162 Create Model
163 Update Model
164 Create Forms
165 Create Views
166 Create List Template
167 Test List View
168 Implement and test create
169 Test Update
170 Test Delete
171 Implement Add Clinical Data View
172 Create Clinical Data Template and Test
173 Implement Analyze View
174 Calculate BMI
175 Create the Report Template and Test
176 Apply Styles
Resolve the captcha to access the links!