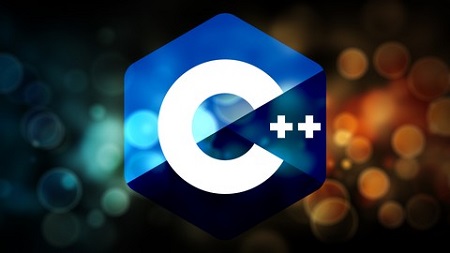
English | MP4 | AVC 1280×720 | AAC 48KHz 2ch | 12.5 Hours | 1.64 GB
Discover the modern implementation of design patterns with С++
This course provides a comprehensive overview of Design Patterns in Modern C++ from a practical perspective. This course in particular covers patterns with the use of:
- The latest versions of the C++ programming language
- Use of modern programming approaches: dependency injection, use of coroutines, and more!
- Use of modern developer tools such as CLion and ReSharper C++
- Discussions of pattern variations and alternative approaches
This course provides an overview of all the Gang of Four (GoF) design patterns as outlined in their seminal book, together with modern-day variations, adjustments, discussions of intrinsic use of patterns in the language.
What are Design Patterns?
Design Patterns are reusable solutions to common programming problems. They were popularized with the 1994 book Design Patterns: Elements of Reusable Object-Oriented Software by Erich Gamma, John Vlissides, Ralph Johnson and Richard Helm (who are commonly known as a Gang of Four, hence the GoF acronym).
The original book was written using C++ and Smalltalk as examples, but since then, design patterns have been adapted to every programming language imaginable: Swift, C#, Java, PHP and even programming languages that aren’t strictly object-oriented, such as JavaScript.
The appeal of design patterns is immortal: we see them in libraries, some of them are intrinsic in programming languages, and you probably use them on a daily basis even if you don’t realize they are there.
What Patterns Does This Course Cover?
This course covers all the GoF design patterns. In fact, here’s the full list of what is covered:
- SOLID Design Principles: Single Responsibility Principle, Open-Closed Principle, Liskov Substitution
- Principle, Interface Segregation Principle and Dependency Inversion Principle
- Creational Design Patterns: Builder, Factories (Factory Method and Abstract Factory), Prototype and Singleton
- Structrural Design Patterns: Adapter, Bridge, Composite, Decorator, Façade, Flyweight and Proxy
- Behavioral Design Patterns: Chain of Responsibility, Command, Interpreter, Iterator, Mediator, Memento,
- Null Object, Observer, State, Strategy, Template Method and Visitor
Who Is the Course For?
This course is for C++ developers who want to see not just textbook examples of design patterns, but also the different variations and tricks that can be applied to implement design patterns in a modern way.
Presentation Style
This course is presented as a (very large) series of live demonstrations being done in JetBrains CLion. Most demos are single-file, so you can download the file attached to the lesson and run it in CLion, XCode or another IDE of your choice (or just on the command line).
This course does not use UML class diagrams; all of demos are live coding.
What you’ll learn
- Recognize and apply design patterns
- Refactor existing designs to use design patterns
- Reason about applicability and usability of design patterns
- Learn how to use different aspects of Modern C++
Table of Contents
Introduction
1 Introduction
SOLID Design Principles
2 Overview
3 Single Responsibility Principle
4 Open-Closed Principle
5 Liskov Substitution Principle
6 Interface Segregation Principle
7 Dependency Inversion Principle
8 Summary
Builder
9 Gamma Categorization
10 Overview
11 Life Without Builders
12 Builder
13 Fluent Builder
14 Groovy-Style Builder
15 Builder Facets
16 Summary
Factories
17 Overview
18 Point Example
19 Factory Method
20 Factory
21 Inner Factory
22 Abstract Factory
23 Functional Factory
24 Summary
Prototype
25 Overview
26 Record Keeping
27 Prototype
28 Prototype Factory
29 Prototype via Serialization
30 Summary
Singleton
31 Overview
32 Singleton Implementation
33 Testability Issues
34 Singleton in Dependency Injection
35 Singleton Lifetime in DI Container
36 Monostate
37 Multiton
38 Summary
Adapter
39 Overview
40 VectorRaster Demo
41 Adapter Caching
42 Summary
Bridge
43 Overview
44 Pimpl Idiom
45 Bridge Implementation
46 Summary
Composite
47 Overview
48 Geometric Shapes
49 Neural Networks
50 Array-Backed Properties
51 Summary
Decorator
52 Overview
53 Dynamic Decorator
54 Static Decorator
55 Functional Decorator
56 Summary
Façade
57 Overview
58 Façade
59 Summary
Flyweight
60 Overview
61 Handmade Flyweight
62 Boost.Flyweight
63 Text Formatting
64 Summary
Proxy
65 Overview
66 Smart Pointers
67 Property Proxy
68 Virtual Proxy
69 Communication Proxy
70 Proxy vs Decorator
71 Summary
Chain of Responsibility
72 Overview
73 Pointer Chain
74 Broker Chain
75 Summary
Command
76 Overview
77 Command
78 Undo Operations
79 Composite Command (Macro)
80 Summary
Interpreter
81 Overview
82 Handmade Interpreter Lexing
83 Handmade Interpreter Parsing
84 Building Parsers with Boost.Spirit
85 Summary
Iterator
86 Overview
87 Iterators in the Standard Library
88 Binary Tree Iterator
89 Tree Iterator with Coroutines
90 Boost Iterator Façade
91 Summary
Mediator
92 Overview
93 Chat Room
94 Event Broker
95 Summary
Memento
96 Overview
97 Memento
98 Undo and Redo
99 Automatic Memento
100 Summary
Observer
101 Overview
102 Observer
103 Observable
104 Observable with Boost.Signals
105 The Problem of Dependencies
106 Thread Safety and Reentrancy
107 Summary
State
108 Overview
109 Classic State Implementation
110 Handmade State Machine
111 State Machine with Boost.MSM
112 Summary
Strategy
113 Overview
114 Dynamic Strategy
115 Static Strategy
116 Summary
Template Method
117 Overview
118 Template Method
119 Summary
Visitor
120 Overview
121 Intrusive Visitor
122 Reflective Visitor
123 Classic Visitor (Double Dispatch)
124 Acyclic Visitor
125 Multimethods
126 Variant and stdvisit
127 Summary
Course Summary
128 End of Course
129 Bonus Lecture Other Courses at a Discount
Resolve the captcha to access the links!