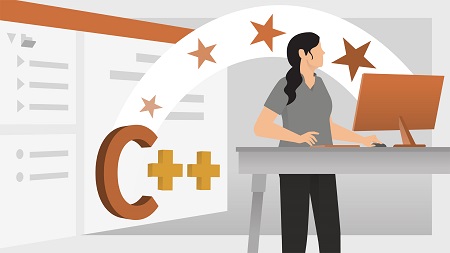
English | MP4 | AVC 1280×720 | AAC 48KHz 2ch | 0h 50m | 120 MB
There have been several updates to C++ over the years. In this course, learn best practices that can help you produce higher-quality C++ code by leveraging tools and new features. To begin, instructor Troy Miles provides a high-level overview of the major features introduced in the 2011, 2014, and 2017 updates to the C++ language. Next, he goes over a few tools that can help you improve your code, including how to boost your code’s quality and reliability using static analyzers. Troy also shares practical tips for using the new C++ features correctly, as well as rules and techniques for managing objects.
Topics include:
- Major new C++ features
- Turning on modern C++
- Letting the compiler help
- Using the new features correctly
- Using auto type deduction
- Using lambdas
- Range-based for loops
- How C++ and the Standard Template Library name things
- Making the best of the filesystem library
- Managing objects
Table of Contents
Introduction
1 Write better C++ code
2 What you should know
What Is Modern C++
3 New C++ features
4 Old code compatibility
5 File naming conventions
Tools That Improve Your Code
6 Turning on modern C++
7 Let the compiler help
8 Static analyzers
9 Cppcheck
10 Challenge – Finding issues with Cppcheck
11 Solution – Finding issues with Cppcheck
Using the New Features Correctly
12 Auto type deduction and initialization
13 Range-based for loops
14 Strongly typed enums
15 Using lambdas
16 The magic of variadic template functions
17 Challenge – Writing an integer sum method
18 Solution – Writing an integer sum method
Don t Reinvent the Wheel
19 How C++ and the STL name things
20 Map filter and reduce
21 Be careful with string view
22 size t and auto
23 The filesystem library
24 Challenge – Writing strings to a file
25 Solution – Writing strings to a file
Managing Objects
26 Which pointer when
27 The rules of zero and three
28 const is your friend
29 Resource Acquisition Is Initialization RAII
30 Challenge – RAII
31 Solution – RAII
Conclusion
32 Next steps
Resolve the captcha to access the links!