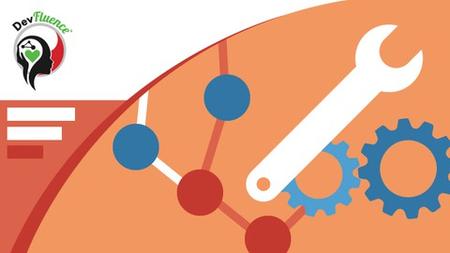
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 101 lectures (9h 4m) | 5.41 GB
This course is for developers who want to improve their ability to write good, clean, maintainable code
The course is for developers who are looking to improve their ability to write good, clean, maintainable code. In it, we cover key coding principles that help to develop a language for discussion within the team. These include coupling and cohesion, the SOLID principles, DRY.
Note: the course examples and exercises are in C#, but the theory covered is applicable in all OO languages.
Goals –
- To get developers thinking about code quality
- To provide developers with the tools they need to discuss and evaluate code quality
- To get developers to build alignment about what “good” code is
- To provide developers with some guidelines about how to go about writing better code
Course Structure –
The following topics are covered:
- Preparation – what is the team’s existing understanding of good code?
- Lenses for Thinking about Code Quality – Thinking about programming like Kent Beck:
- Core Values of Software Development
- Key Principles
- The Four Rules of Simple Design
- Cohesion and Coupling
- DRY
- SOLID Principles:
- Single Responsibility Principle
- Open-Closed Principle
- Liskov Substitution Principle
- Interface Segregation Principle
- Dependency Inversion Principle
- The Practice of Writing Clean Code:
- Coding Standards
- Meaningful naming
- Functions
- Comments
Learning Outcomes
At the end of this course developers will be able to:
- Understand why code quality is important and how it enables effective software development
- Describe key coding principles such as cohesion & coupling, DRY, and the SOLID principles
- Reason about code quality using a variety of lenses
- Discuss code quality ideas among a team and be able to, as a team, work towards alignment and agreement on the team’s coding standards
What you’ll learn
- Understand why code quality is important and how it enables effective software development
- Describe key coding principles such as cohesion & coupling, DRY, and the SOLID principles
- Improve your reasoning ability about code quality by using a variety of lenses
- Write cleaner, more readable, more communicative, more flexible code than before
- Discuss code quality ideas amongst a team and be able to, as a team, work towards agreement on a team’s coding standards
- Write cleaner, more readable, more communicative, more flexible code than before
Table of Contents
Welcome to Core Coding Principles
1 Introduction
2 Welcome to Core Coding Principles
Principle SOLID
3 Introduction
4 Single Responsibility Principle
5 Exercise Single Responsibility Priciple
6 OpenClosed Principle
7 Exercise OpenClosed Principle
8 Liskov Substitution Principle
9 LSP Example Invariants
10 LSP Example Pre and Post Conditions
11 LSP Furthur Thoughts
12 Exercise Liskov Substitution Principle
13 Interface Segregation Principle
14 Dependency Inversion Principle
15 Summary and Final Thoughts
16 Notes Further Reading and an Exercise
Rule Clean Code
17 Comments When to use When not to Use
18 Notes Further Reading and Exercise
19 A Word on Clean Code
20 What is Clean Code
21 The Scout Camp Rule
22 Guidelines for Cleaning Code
23 Coding Standards
24 Naming Introduction
25 Naming A Four Stage Process of Naming
26 Naming Guidelines General
27 Naming Guidelines Classes
28 Naming Guidelines MethodsFunctions
29 Naming Finding Names
30 Naming is Hard ChilliTalk Podcast Episode
31 Functions Introduction
32 Functions Principles
33 Functions Keep them Small
34 Functions Naming and One Level of Abstraction
35 Functions Parameters
36 Functions More General Principles and Recap
37 Comments Intro
38 Comments Why are they Dangerous
Axioms and Lenses
39 Notes Further Reading and Exercises
40 Axioms What are they why talk about them
41 Axiom 1 Managing Complexity is at the Heart of Software Development
42 Axiom 2 Code is Required for any Sufficiently Complex Problem
43 Axiom 3 Coding is High Read Low Write System
44 Lemma 1 Code Quality Matters
45 Axiom 4 We can use Lenses to Evaluate Code
46 House of Lenses How Lenses Relate to One Another
Values
47 Introduction What are Values
48 Communication
49 Simplicity
50 Flexibility
51 End Note
52 Notes Further Reading and Exercises
Concept Coupling Cohesion
53 Introduction
54 Coupling Overview
55 Types of Coupling Semantic and Data Coupling
56 Semantic Coupling 1 Content Coupling
57 Semantic Coupling 2 Common Coupling
58 Semantic Coupling 3 Subclass Coupling
59 Semantic Coupling 4 Temporal Coupling
60 Semantic Coupling 5 Control Coupling
61 Semantic Coupling 6 External Coupling
62 Data Coupling 1 Data Stamp Coupling
63 Data Coupling 2 Simple Object Coupling
64 Data Coupling 3 Simple Data Coupling
65 Cohesion
66 Encapsulation
67 End Note
68 Notes and Further Reading
Concept Refactoring
69 What is Refactoring
70 The Practice of Refactoring
71 Refactoring Demo 1 Rename
72 Refactoring Demo 2 Move
73 Refactoring Demo 3 Delete
74 Refactoring Demo 4 Extract and Inline
75 Refactoring Demo 5 Summary and Thoughts
76 Notes Further Reading and Exercises
77 Worked Example Tennis Refactoring Kata Intro
78 Worked Example Tennis Refactoring Kata Extended
Concept Cyclomatic Complexity
79 Cyclomatic Complexity and Nesting
80 Demo Part 1 Refactoring a Sample Project to Reduce Cyclomatic Complexity
81 Demo Part 2 Move Parameter to the Constructor Introduce Better Types
82 Demo Part 3 Further Reduce Parameters by Introducing a Class
83 Demo Part 4 Final Test Refactorings Introducing a SUT Builder
84 Further Reading and Extercises
Principle Kent Becks Development Principles
85 Introduction
86 Local Consequences
87 Minimize Repetition
88 Symmetry
89 Declarative Expression
90 Rates of Change
91 Summary
92 Further Reading and Exercises
Heuristic DRY
93 What is DRY and what is it not
94 Common Duplication Forms
95 Pitfalls of DRY
96 Notes and Further Reading
Heuristic Four Rules of Simple Design
97 Introduction Kent Becks Formulation
98 Martin Fowlers Formulation
99 JB Rainsbergers Formulation
100 Applying the Four Rules of Simple Design
101 Notes Further Reading and an Exercise
Resolve the captcha to access the links!