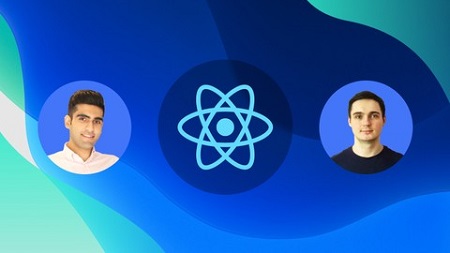
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 16.5 Hours | 8.63 GB
Become an advanced ReactJS developer. Build and deploy three production-ready apps with React Hooks, ContextAPI, ES2020.
Created with up-to-date versions of React, React Hooks, Node.js, JavaScript, and Firebase.
This course is about React – a library that helps developers to create user interfaces on the web. But React is not limited only to user interfaces, there is much more to that.
Have you ever wondered how Facebook, Twitter, or Netflix websites are built and why they don’t feel like websites at all? React can answer all of that. In this course, we show how to create mobile-feel-like websites (Single Page Apps) where React is the foundation.
First, we will guide you through the basics of web development before jumping into React. Here we will talk about the latest JavaScript, Node.JS, Git, APIs, and essential tools to make you feel as comfortable as possible at any stage of the development process.
Then we slightly move to React with a small portion of theory. At this point, you will get to know what React is made of and how it works.
Our first project is a Tic-Tac-Toe game. Here you will get to know React basics, hooks and core functionality. By the end of this project, you will be able to create a simple web app that shows off your strong React basics. Here you will experience the development flow of a React app in general.
The second project is a movie search web-app called Box Office. With this project, we move towards more complex React use-cases and we start working with external APIs, dynamic layout and pages combined with different approaches for styling a React app. Here we will work with more advanced and custom React Hooks. At the end of this project we will analyse and optimize the app with React hooks to make it even more faster and reliable. We will even turn it into a Progressive Web App that works offline!
The final project is going to be a Chat app. It will include the following features: social media logins, account management, role-based permissions, real-time data, and lots of others. In this project we will combine React with Firebase – a backend solution in the cloud, powered by NoSQL database. You will master global state management with Context API and sharpen your knowledge of React hooks. At the final stage we will extend the app with custom backend in form of serverless.
All of our projects will have a user-friendly and well-designed user interface that is responsive and optimized for all devices.
Is this course exactly what are you looking for?
If …
- … you are eager to learn front-end development with React from scratch …
- … you have some experience with React, but you don’t feel confident …
- … you only started to learn web development and want to move towards modern tools and technologies …
- … you feel that you stuck doing only HTML, CSS and some JS …
… then this course is definitely for you!
What do you need to succeed in this course?
- HTML and CSS is absolutely required
- General/basic understanding of programming or JavaScript
- No prior experience with React or JS frameworks
- Passion to learn new things
After this course, you will have:
- Three real-world React projects of different complexity that can be put into your resume
- Total React comprehension
- Experience with popular React libraries
- Knowledge of how to create and deploy React apps
- Knowledge of custom serverless backend and Firebase
Topics that will be covered and explained:
- React basics (syntax, core concepts, theory)
- Scaffolding templates (create-react-app, nano-react-app /w Parcel)
- Styling of React apps (CSS, SASS, UI components library, CSS-IN-JS /w Styled components)
- Conditional rendering (dynamic content and styles)
- State management, local + global (/w React Hooks, Context API)
- Components analysis and optimization (/w React hooks)
- Complex layout management
- Dynamic pages with React Router
- Progressive Web Apps and service workers
- Real-time subscriptions in React
- Using external APIs to fetch remote data
- Deployment of React apps
- Serverless backend with cloud functions
- Latest and modern JavaScript (ES6 – ES2020)
Not part of React, but included:
- Git, Node.js, APIs, ESLint and Prettier quick guides
- Firebase (/w NoSQL realtime database, cloud functions, cloud messaging, cloud storage)
- Serverless cloud computing idea and concept + explanation about docker containers
What you’ll learn
- Build and deploy responsive and production-ready React apps from scratch
- Setup and develop a React app with firebase (cloud functions, cloud messaging, realtime database, storage, auth)
- Master React Hooks and latest React syntax
- Master state management with React Hooks and Context API
- Learn and practice bleeding-edge JavaScript (ES2020)
Table of Contents
Introduction
1 Welcome To The Course
2 ProShop Project Demo
3 Resources & Environment
Starting The Front End
4 React Setup & Git Initialize
5 React-Bootstrap Setup, Header & Footer Components
6 HomeScreen Product Listing
7 Rating Component
8 Implementing React Router
9 Product Details Screen
Serving & Fetching Data From Express
10 Front End Back End Workflow & Explanation
11 Serving Products – Back End Routes
12 Fetching Products From React (useEffect)
13 Nodemon & Concurrently Setup
14 Environment Variables
15 ES Modules In Node.js
Getting Started With MongoDB
16 MongoDB Atlas & Compass Setup
17 Connecting To The Database
18 Adding Colors To The Console (Optional)
19 Modeling Our Data
20 Preparing Sample Data
21 Data Seeder Script
22 Fetching Products From The Database
23 Getting Started With Postman
24 Custom Error Handling
Implementing Redux For State Management
25 An Overview Of Redux
26 Create a Redux Store
27 Product List Reducer & Action
28 Bringing Redux State Into HomeScreen – useDispatch & useSelector
29 Message & Loader Components
30 Product Details Reducer & Action
Adding The Shopping Cart
31 Qty Select & Add To Cart Button
32 Cart Reducer & Add To Cart Action
33 Add To Cart Functionality
34 Cart Screen
35 Remove Items From Cart
Back End User Authentication
36 Clean Up By Using Controllers
37 User Authentication Endpoint
38 Brief Explanation of JWT (JSON Web Tokens)
39 Generate a JSON Web Token
40 Custom Authentication Middleware
41 Saving The Token In Postman
42 User Registration & Password Encryption
Front End User Authentication & Profile
43 User Login Reducer & Action
44 User Login Screen & Functionality
45 Show User In Navbar & Logout
46 User Register Reducer, Action & Screen
47 Update Profile Endpoint
48 Profile Screen & Get User Details
49 Update User Profile
Checkout Process – Part 1
50 Shipping Screen & Save Address
51 Checkout Steps Component
52 Payment Screen & Save Payment Method
53 Place Order Screen
54 Order Controller & Route
55 Create Order
Checkout Process – Part 2
56 Get Order by ID Endpoint
57 Order Details Reducer & Action
58 Order Screen
59 Update To Paid Endpoint
60 Order Pay Reducer & Action
61 Adding PayPal Payments
62 Show Orders On Profile
63 User Details & Orders Reset
Admin Screens – Part 1
64 Admin Middleware & Get Users Endpoint
65 Admin User List
66 Admin Screen Access Security
67 Admin User Delete
68 Get User By ID & Update User Endpoints
69 User Edit Screen & Get User Details
70 Update User Functionality
Admin Screens – Part 2
71 Admin Product List
72 Admin Delete Products
73 Create & Update Product Endpoints
74 Admin Create Product
75 Edit Product Screen
76 Admin Update Product
77 Image Upload Config & Endpoint
78 Front End Image Upload
79 Admin Order List
80 Mark Order As Delivered
Product Reviews, Search & More
81 Morgan & Create Review Endpoint
82 Front End Product Reviews
83 Product Search
84 Product Pagination
85 Top Products Carousel
86 Custom Page Titles & Meta
App Deployment
87 Prepare For Deployment
88 Deploy To Heroku
Introduction
1 What is This Course All About
2 Requirements
Before We Start
3 Introduction
4 Installing Git (+ Git Bash)
5 Installing Node.js
6 Installing VSCode
7 VSCode Configuration & Extensions
8 Client Side Rendering (SPA) VS. Server Side Rendering (dynamic and static)
9 What are Server, JSON, REST API and GraphQL
10 Github Note
11 Working With Git – Part 1
12 Working With Git – Part 2
13 What are Node.js and NPM
14 What are ESLint and Prettier
15 VS Code Shortcuts
Look Through Modern JavaScript
16 Introduction
17 Var VS. Let VS. Const
18 What is Array.map used for
19 What is Array.reduce used for
20 Function Declaration VS. Expression
21 Arrow Functions and Default Function Arguments
22 String Interpolation
23 Object and Array Destructuring
24 Spread and Rest Operators
25 Async and Sync Code – Promises and Async Await
What is React Exactly
26 What is React
27 Component-based Approach
28 Functions vs. Classes
29 React Under the Hood
30 Check how good you undestand React
Starting up With React (Parcel) Tic Tac Toe Game
31 Introduction
32 Required Assets
33 Scaffolding a React App With Parcel – Installing Development Dependencies.
34 Installing Dev Dependencies
35 Creating New Components & Props
36 Children Props
37 Styles note
38 Styling React Components – Our Style Structure
39 Creating a Game Board State – React States and Events
40 Component Lifecycle & Lifecycle Events
41 Winner Calculation – State Sharing Across Components
42 Adding Game History – Part 1
43 Adding Game History – Part 2
44 Conditional Rendering
45 Highlighting Winning Combination
46 Styling The Entire App
47 Deployment to Surge
48 Summary
49 Final Project
Box Office Project – CRA
50 Introduction
51 Required Assets
52 Scaffolding the Project
53 Creating Pages in React – React Router (Part 1)
54 Creating Navigation Bar & Page Components
55 Multiple Layout Management – Creating Main Layout
56 Creating Search Bar – Working With an API
57 Displaying API Results – Custom Render Function
58 Adding Actors Search With Radio Buttons
59 Creating Preview Cards for Show and Actors
60 Styled-components – An Alternative for Styling
61 Creating Show Page – UseEffect Hook and Dynamic URLs
62 Displaying Loading and Error Messages – Part 2
63 Reducer Concept for State Management
64 Creating Show Page – Part 3
65 Creating Show Page – Part 4
66 Styling Show Page – Part 5
67 Creating Custom Hook For Data Persistence
68 Dynamic Styles With Styled-Components
69 Displaying Starred Shows
70 Extract any Logic you want – Custom Hook
71 Styling The Entire App – Part 1
72 Styling The Entire App – Part 2
73 Add Animation to Elements
74 Deployment to GitHub Pages
75 Component Optimization With Hooks
76 Creating a Progressive Web App (PWA)
77 Final Project
Firebase
78 What is Firebase
79 Overview of Firebase Services
80 Firebase Security
81 Firebase Pricing
Chat Application Project
82 Project Overview
83 Required Assets
84 Scaffolding the Project
85 Create and Configure the Firebase Project
86 Creating Pages – Private and Public Routes
87 Sign-in Page – Interaction with Firebase
88 Creating Profile Context – Context API and Global State Management
89 Global Profile State Management With Context
90 Start Building the Sidebar and Dashboard
91 Responsive Sidebar Using Media Query
92 Creating Dashboard – Part 1
93 Creating Dashboard – Reusable and Editable Components (Part 2)
94 Creating Dashboard – Update User Nickname (Part 3)
95 Creating Dashboard – Link Social Media Providers (Part 4)
96 Creating Dashboard – Creating Avatar (Part 5)
97 Creating Dashboard – Uploading the Avatar to Firebase (Part 6)
98 Creating Dashboard – Displaying User Avatar and Names Initials (Part 7)
99 Add Create-Room Button and Functionality
100 Creating Chat Rooms Lists – Part 1
101 Creating Chat Rooms List – Rooms’ context (Part 2)
102 Creating Chat Rooms List – Show rooms and use them as links (Part 3)
103 Creating Nested Layout for Homepage
104 Creating Chat Page LayoutComponent
105 Context API Problem and a Potential Solution
106 Context API Problem in Practice – Creating Current Room Context
107 Creating Initial Chat – Top Part
108 Denormalizing Data – Creating Chat Bottom
109 Display Last Message in Room List
110 Working With Denormalized Data
111 Displaying Chat Messages
112 Display User Profile Data
113 Adding Real-time Presence – Part 1
114 Adding Real-time Presence – Part 2
115 Adding Edit Room Drawer
116 Role-based Access & Security Rules
117 Role-based Access Management
118 Adding Programmatical Hover With Hooks
119 Creating IconControl Component – Likes (Part 1)
120 Likes Functionality (Part 2)
121 Handle Delete Operation
122 Adding the Upload Component – Part 1
123 Store Uploaded Files in Database – Part 2
124 Display and Download Uploaded Files – Part 3
125 Record and Upload Audio Messages – Part 4
126 Display Audio and Delete File – Part 5
127 Group Chat Feed by Dates
128 Pagination and Control of Scrolled Position
129 Deployment to Firebase Hosting
Custom Chat Backend
130 Firebase Project Plan
131 What are Serverless and Containers
132 Cloud Messaging – How is Everything Connected
133 Storing Device Tokens in the Database
134 Adding Service Worker
135 Setup Cloud Functions and Node Version Manager (NVM)
136 Notifications Flow in our app – Types of Cloud Functions
137 Creating FCM Cloud Function
138 Fix Cloud Function Errors
139 Sending and Displaying Notifications Using Cloud Functions
140 Managing FCM Users
141 Security rules note
142 Final Project
Key Features of React
143 Introduction
144 React Portals
145 Error Boundaries
146 Code Splitting
147 Conclusion
What is the Next Move
148 Summary – Knowledge you Have Gained
149 Your Future Moves
Bonus Lecture
150 Bonus Lecture
Resolve the captcha to access the links!