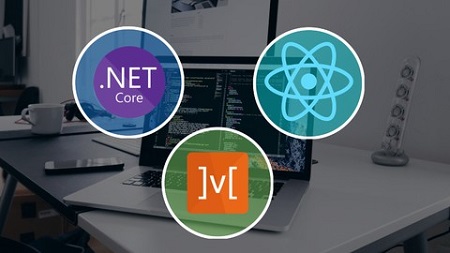
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 37.5 Hours | 17.5 GB
The complete guide to building an app from start to finish using ASP.NET Core, React (with Typescript) and Mobx
Course has now been updated for .Net Core 3.0
Have you learnt the basics of ASP.NET Core and React? Not sure where to go next? This course should be able to help with that. In this course we learn how to build a multi-project ASP.NET Core solution that is built using Clean Architecture and the CQRS and Mediator pattern that makes our code easy to understand, reason about and extend.
Both ASP.NET Core and React are hot topics and this course will enhance your knowledge of both, simply by building an application from start to finish. In each module we learn something new, whilst incrementally adding features to the application. Building an application is significantly more rewarding than building yet another Todo List from the documentation!
Every line of code is demonstrated and explained and by the end of this course you will have the skills and knowledge to build your own application using the techniques taught in this course.
Here are some of the things you will learn about in this course:
- Setting up the developer environment
- Creating a multi-project solution using the the ASP.NET Core WebAPI and the React app using the DotNet CLI and the create-react-app utility.
- Clean Architecture and the CQRS + Mediator pattern
- Setting up and configuring ASP.NET Core identity for authentication
- Using React with Typescript
- Adding a Client side login and register function to our React application
- Using React Router
- Using AutoMapper in ASP.NET Core
- Building a great looking UI using Semantic UI
- Adding Photo Upload widget and creating user profile pages
- Using React Final Form to create re-usable form inputs with validation
- Paging, Sorting and Filtering
- Using SignalR to enable real time web communication to a chat feature in our app
- Publishing the application to both IIS and Linux
- Getting an ‘A’ rating for security from a well known security scanning site.
- Many more things as well
Tools you need for this course
In this course all the lessons are demonstrated using Visual Studio Code, a free (and fantastic) cross platform code editor. You can of course use any code editor you like and any Operating system you like… as long as it’s Windows, Linux or Mac
Is this course for you?
This course is very practical, about 90%+ of the lessons will involve you coding along with me on this project. If you are the type of person who gets the most out of learning by doing, then this course is definitely for you.
On this course we will build an example social network application that allows users to sign up to events (similar to MeetUp or Facebook), completely from scratch. All we start with is an empty terminal window or command prompt.
All you will need to get started is a computer with your favourite operating system, and a passion for learning how to build an application using ASP.NET Core and React
What you’ll learn
- Learn how to build an application from start to publishing with .Net Core (v2.2), React (with Typescript) and Mobx
- How to build a Web API in .Net Core with Clean Architecture using the CQRS + Mediator pattern
- How to use AutoMapper and MediatR packages in the .Net projects
- How to build a multi-project solution with .Net Core
- How to use Entity Framework Core as the Object Relational Mapper
- How to integrate ASPNET Core SignalR into an application for real time web communication
- How to add Identity and Authentication using .Net Core Identity
- How to build a Client side application for the API with React
- How to use MobX as a state management library
- How to build our own Photo upload widget with a Dropzone and a Cropper to resize images
Table of Contents
Introduction
1 Course Introduction
2 Setting up the dev environment
3 Visual Studio Code extensions
4 Source code and resources for this course
Walking Skeleton Part 1 – API
5 Section 2 introduction
6 Creating the ASP.NET Core solutions and projects using the DotNet CLI
7 Creating the project references using the DotNet CLI
8 Reviewing the Project files
9 Running the application
10 Creating a Domain entity
11 Creating the DbContext and service
12 Adding our first Entity Framework code first migration
13 Creating the database
14 Seeding data using Entity Framework fluent configuration
15 Using Dependancy Injection
16 Introduction to Postman
17 Saving our changes into Source control using Git
18 Section 2 summary
Walking Skeleton Part 2 – Client
19 Section 3 introduction
20 Using create-react-app to create our React application
21 Reviewing the React project files
22 Introduction to React – Concepts
23 Introduction to React – Components
24 Introduction to Typescript
25 Typescript basics demo
26 Using Typescript with React
27 Adding React dev tools
28 React Class Components
29 Using React State
30 Fetching data from the API
31 Adding CORS support to the API
32 Adding Semantic UI to our app
33 Clean up and saving our code to source control
34 Summary of section 3
Building a CRUD application in .Net Core using the CQRS + Mediator pattern
35 Section 4 introduction
36 Adding the Activity entity
37 Seeding Activity data
38 Commands and Queries – CQRS
39 Introduction to MediatR
40 Creating our first Query handler
41 Creating the Activities API Controller
42 Adding the Details Handler
43 Cancellation Tokens
44 Adding the Create handler
45 Dealing with boilerplate code in our handlers
46 Adding an Edit handler
47 Adding a Delete handler
48 Summary of section 4
Building a CRUD application in React
49 Section 5 introduction
50 Introduction to React Hooks
51 Folder structure in React
52 Getting a list of activities from the API
53 Adding an Activity interface in Typescript
54 Refactoring our class component to use React Hooks
55 Adding the Navigation bar
56 Styling React components
57 Adding the Activity Dashboard
58 Creating the Activity List
59 Adding the Activity Details component
60 Adding the Activity Form component
61 Selecting an individual Activity
62 Adding an edit mode to display the form
63 Adding a create activity mode
64 Initialising the form with data
65 Controlled components in React
66 Handling form submission
67 Fixing issues with the dates in the form
68 Adding the delete functionality
69 Summary of section 5
Axios
70 Section 6 introduction
71 Setting up the agent.ts file
72 Listing our activities
73 Updating an Activity
74 Adding a delay to our API methods
75 Adding a loading component
76 Adding a loading indicator for submitting data
77 Isolating the loading indicator on the delete button
78 Summary of section 6
MobX
79 Section 7 introduction
80 Introduction to MobX
81 Setting up a MobX store
82 Refactoring the activity list to use the store
83 Refactoring the select activity function
84 Using Async Await in our methods
85 Refactoring the create activity method
86 MobX computed properties
87 Using an Observable Map
88 Adding the edit activity action
89 Adding the delete activity action
90 Cleaning up our code
91 Enabling MobX strict mode
92 Adding MobX dev tools
93 Summary of section 7
React Router
94 Section 8 introduction
95 Setting up React Router
96 Setting up our Routes
97 Adding Links and NavLinks
98 Adding the Details link
99 Getting an Activity from the API
100 Using Route params
101 Navigating via code
102 Routing to the edit form
103 Using a Fully Uncontrolled Component with a key to reset component state
104 Navigating after submission
105 Moving the home page outside of our navigation routes
106 Scrolling to the top of the page on navigation
107 More code clean up
108 Summary of section 8
Adding some style
109 Section 9 introduction
110 Styling the activity list
111 Grouping activities by date
112 Styling the activity list items
113 Creating the Activity Details page
114 Styling the Activity Detailed Page Header
115 Styling the Activity Detailed Info
116 Styling the Activity Detailed Chat and Sidebar components
117 Styling the Activity Form
118 Styling the Home page
119 Section 9 summary
Error handling and validation
120 Section 10 introduction
121 Introduction to validation in the API
122 Adding validation in the API using Data Annotations
123 Adding validation in the API using Fluent Validation
124 Error handling concepts in our application
125 Error handling strategy
126 Creating a derived Exception class for Rest exceptions
127 Adding Error handling middleware
128 Using our Error handling middleware
129 Using Axios interceptors to catch errors
130 Throwing errors in the client
131 Adding routing functionality to Axios
132 Handling an invalid GUID on a get request
133 Adding toast notifications
134 Handling network errors in Axios
135 Summary of section 10
Forms
136 Section 11 introduction
137 Setting up React Final Form
138 Creating a reusable Text input field
139 Refactoring the form to use React Final Form
140 Creating a reusable Text Area Input field
141 Creating a reusable Select input field
142 Setting up React Widgets
143 Creating a reusable Date Input field
144 Formatting the dates using Date-FNS
145 Creating separate Date and Time fields
146 Combining Date and Time inputs
147 Initialising the form with data
148 Submitting data to the server
149 Form error handling
150 Form validation
151 Summary of section 11
ASP.NET Core Identity
152 Section 12 introduction
153 Introduction to ASP.NET Core Identity
154 Adding the Identity User Entity
155 Configuring Identity in our startup class
156 Seeding users to the database
157 Adding a Login Handler
158 Adding a Base API controller
159 Adding a User API controller
160 Adding a User object
161 JSON Web Tokens introduction
162 Adding the Infrastructure project
163 Adding the JWT Generator interface and class
164 Generating a JWT Token
165 Returning the JWT Token on successful login
166 Securing our app with Authorization
167 Dotnet user secrets
168 Adding an authorisation policy
169 Adding a Register Handler
170 Testing user registration
171 Adding a Fluent Validator extension for password validation
172 Retrieving the Username from the token in the Http Context
173 Getting the currently logged in user
174 Summary of section 12
Client side login and register
175 Section 13 introduction
176 Creating the Typescript interfaces and Axios methods
177 Creating a Mobx user store
178 Creating a MobX root store
179 Creating the Login form
180 Hooking up the Login form to the API
181 Dealing with submission errors
182 Adding home page and NavBar user information
183 Creating a MobX common store
184 Using Axios request interceptor to automatically send the JWT token
185 Persisting login on refresh
186 Adding Modals to our application
187 Adding better error messages to our form
188 Adding a Register form
189 Displaying server validation errors in our form
190 Summary of section 13
Entity Framework Core Relationships
191 Section 14 introduction
192 Adding a class to join our Users and Activities
193 Updating the Create Activity handler
194 Testing in Postman
195 Loading related data using Eager loading
196 Adding DTOs to shape our data
197 Adding AutoMapper
198 Adding AutoMapper profiles
199 Configuring AutoMapper mappings
200 Using Lazy Loading to load related data
201 Adding the Join activity feature
202 Adding the remove attendance feature
203 Creating a custom Auth policy
204 Updating our Seed data
205 Section 14 summary
Adding Client side attendances
206 Section 15 introduction
207 Adding attendees to our list items
208 Adding attendees to the Detailed view sidebar
209 Adding the IsGoing and IsHost properties to the Activity interface
210 Conditionally rendering the activity detailed header buttons
211 Adding the cancel attendance function
212 Hooking it all up to the API
213 Loose ends
214 Summary of section 15
Photo Upload Part 1 – API
215 Section 16 introduction
216 Cloudinary settings
217 Adding the interface and class for our Photo Accessor
218 Adding the AddPhoto handler
219 Adding the Domain Entity
220 Adding the Add Photo Handler
221 Adding the Photo API Controller
222 Adding User Profiles feature
223 Adding the Delete photo handler
224 Adding the set main photo functionality
225 Adding the Mapping Profile configuration for User images
226 Summary of section 16
Photo Upload Part 2 – Client
227 Section 17 introduction
228 Adding links and a profile page component
229 Adding the Profile Header component
230 Adding the Profile content component
231 Getting the profile data from the API
232 Displaying User images on the profile page
233 Conditionally rendering the Photo Upload Widget
234 Creating a Photo Upload Widget
235 React Dropzone
236 Styling our Dropzone
237 React Cropper Part 1
238 React Cropper Part 2
239 Adding the Photo Upload methods to the store
240 Adding the photo upload functionality to the Profile component
241 Adding the set main photo functionality
242 Isolating our loading indicators
243 Adding Delete photo functionality
244 Summary of section 17
Challenge
245 Challenge Introduction
246 Challenge Solution
SignalR
247 Section 19 Introduction
248 Adding the Comment entity
249 Adding the Comment DTO and mapping
250 Adding the Create comment handler
251 Adding a SignalR hub
252 Configuring Auth for SignalR
253 Adding the SignalR hub connection to the client
254 Connecting to the SignalR hub from our client
255 Adding the Comment functionality to the client
256 Formatting Dates in words using Date-FNS
257 Using SignalR Groups in the API
258 Using SignalR Groups in the client
259 Section 19 summary
FollowingFollower feature
260 Section 20 introduction
261 Adding the UserFollower Entity
262 Adding the Add Follower handler
263 Adding the Delete Following handler
264 Adding a Following API Controller
265 Adding a Profile reader helper class and interface
266 Getting a List of Followings for a user
267 Adding a Custom value resolver for AutoMapper
268 Adding the UI components to show followed users
269 Adding the Follow Unfollow methods
270 Adding the Follow Unfollow UI components
271 Getting a list of followings from the API
272 Using MobX reactions to get followers or followings on tab change
273 Section 20 summary
Paging, Sorting and Filtering
274 Section 21 introduction
275 Paging our Activity list
276 Refactoring the Activity store list method for paging
277 Adding vertical paging to our activity dashboard
278 Adding infinite scrolling to our activity dashboard
279 Updating the dashboard with Filter component
280 Filtering our Activity List in the API
281 Adding the Filtering methods in the client
282 Updating the Activity Filters UI to allow filtering
283 Adding User Activities filter in the API
284 Adding User Activities filter in the client
285 Summary of section 21
Final touches and publishing
286 Section 22 introduction
287 Swapping our loading indicator for Placeholders
288 Adding a loading indicator to index.html
289 Adding private routes on the client
290 Adding logout for token expiry
291 Safari bug fix
292 Preparing the client application for publishing
293 Building a production version of the React app
294 Serving the production React app from the API server
295 Adding MySQL and configuring a user
296 Adding additional DB providers for Entity Framework
297 Swapping the DB for MySQL
298 Publishing the app to Linux – Part 1
299 Publishing the app to Linux – Part 2
300 Configuring Apache to use an HTTPS SSL certificate
301 Adding Security headers to our API
302 Adding Content Security Policy to our API
303 Getting an A rating from a SecurityHeaders site scan
304 Setting up Azure for publishing our app
305 Publishing our App to Azure
306 Tweaking the Azure deployment
307 End of course summary
Bonus – Identity Cookbook
308 Intro to the bonus section
309 FB Login – Setting up the app in Facebook
310 FB Login – Creating a new Git branch to work from
311 FB Login – Adding a Facebook login button to the client app
312 FB Login – Adding the API configuration to user secrets
313 FB Login – Adding a facebook accessor service to the infrastructure project
314 FB Login – Adding the Facebook login handler
315 FB Login – Adding the API Controller method and tying it together with the clien
316 FB Login – Adding loading indicator and merging changes with master branch
317 Refresh Tokens – Introduction
318 Refresh Tokens – Updaing the Domain and adding a new Migration
319 Refresh Tokens – Updating the Token Validation Expriry
320 Refresh Tokens – Updating the Application layer classes
321 Refresh Tokens – Adding the Refresh Token Handler
322 Refresh Tokens – Adding the API Endpoint
323 Refresh Tokens – Testing in Postman
324 Refresh Token – Updating the MobX Stores
325 Refresh Tokens – Testing it works
326 Email Verification – Introduction
327 Email Verification – Setting up SendGrid
328 Email verification – Identity settings
329 Email verification – Updating the register handler
330 Email verification – Adding a confirm email handler
331 Email verification – Testing email verification in postman
332 Email verification – Resend verification email handler
333 Email verification – Client agent methods
334 Email Verification – Create a register success component
335 Email verification – creating a verify email component
336 Email verification – making sure it works
Bonus – Updating the React client packages to latest versions
337 Updating the client packages
Resolve the captcha to access the links!