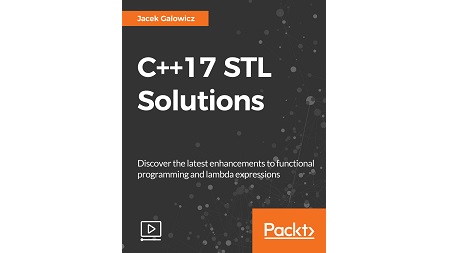
English | MP4 | AVC 1920×1080 | AAC 48KHz 2ch | 4h 55m | 1.08 GB
Over 25 videos that leverage the powerful features of the Standard Library in C++17.
solve many problems. The upcoming version of C++ will see programmers change the way they code. If you want to grasp the practical usefulness of the C++17 STL in order to write smarter, fully portable code, then this course is for you.
Beginning with new language features, this course will help you understand the language’s mechanics and library features, and offers insight into how they work. Unlike other courses, ours takes an implementation-specific, problem-solution approach that will help you quickly overcome hurdles. You will learn the core STL concepts, such as containers, algorithms, utility classes, lambda expressions, iterators, and more, while working on practical real-world videos. These videos will help you get the most from the STL and show you how to program in a better way.
By the end of the course, you will be up to date with the latest C++17 features and save time and effort while solving tasks elegantly using the STL.
This solution-based guide will show you how to make the best use of C++ together with the STL to squeeze more out of the standard language.
What You Will Learn
- Learn about the new core language features and the problems they were intended to solve.
- Understand the inner workings and requirements of iterators by implementing them.
- Explore algorithms, functional programming style, and lambda expressions.
- Leverage the rich, portable, fast, and well-tested set of well-designed algorithms provided in the STL.
- Work with strings the STL way instead of handcrafting C-style code.
- Understand standard support classes for concurrency and synchronization, and how to put them to work.
Table of Contents
The Course Overview
Operations on std::vector
Advance Operations on std::map
Filtering Duplicates from User Input and Implementing a Simple RPN Calculator
Implementing Higher Order Applications
Building Your Own Iterators
Implementing High End Algorithms
Advance Operations with Iterators
Experimenting with Functions Using Lambda Expressions
Playing Around with Logical Expressions
Operations on Containers
Working with Vectors
Locating Patterns in Strings and Sampling Large Vectors
Generating Permutations and Implementing a Dictionary
Implementation Using Trie
Calculating Error Sum, Implementing Mandelbrot Renderer, and Building Split
Code Optimization Using High End Algorithms
Creating, Concatenating, and Transforming Strings
Operations with File
Playing Around with Iterators
Applications
Conversion and Safely Signalizing Function
Applications of Tuples
Working with Smart Pointers
Random Numbers
Executing Sleeping Time and Exploring Threads
Getting to Know about the Locking Mechanism
Having Fun Around Thread Synchronization
Simultaneous Execution Using Future Class
Resolve the captcha to access the links!