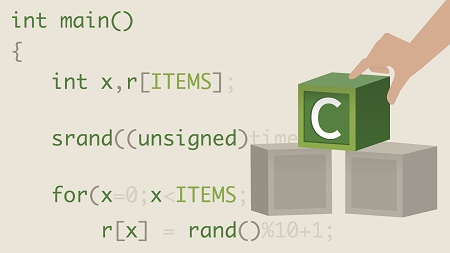
English | MP4 | AVC 1280×720 | AAC 48KHz 2ch | 2h 31m | 381 MB
C has been around for nearly half a century, but it’s still as essential as ever. This powerful language lies at the heart of many modern languages—including JavaScript and Objective-C—and is an up-and-coming language for embedded systems. Whether you’re taking your very first steps into programming with C, or you just want to brush up on the basics, this course can help to acquaint you with the fundamentals of this mid-level programming language. Instructor Dan Gookin dissects the anatomy of C, from data types and variables to simple functions. Plus, he shows how these basic elements fit together in control structures like loops—where the real logic behind your code comes into play.
Topics include:
- Working the C development cycle
- Writing a simple program
- Adding comments to code for clarity
- C language data types
- Declaring variables
- Specifying characters and strings
- Working with math operators
- Creating a for loop
- Prototyping a function
- Creating recursive functions
Table of Contents
1 Start your C essential training
2 Using the exercise files
3 Obtaining and configuring the IDE
4 Getting into the C language
5 Working the C development cycle
6 Writing a simple program
7 Challenge Write your own code
8 Solution Write your own code
9 Reviewing code structure
10 Exploring the preprocessor
11 Understanding header files and libraries
12 Adding comments
13 Challenge Comment code
14 Solution Comment code
15 Understanding C language data types
16 Using constants
17 Declaring variables
18 Working with variables
19 Challenge Make variables and constants
20 Solution Make variables and constants
21 Understanding variable scope
22 Making new variables
23 Using typedef with structures
24 Specifying characters and strings
25 Specifying integers and real numbers
26 Typecasting a variable
27 Working with math operators
28 Putting math operators to work
29 Challenge Do some math
30 Solution Do some math
31 Using assignment operators
32 Obeying the order of precedence
33 Challenge Get the order correct
34 Solution Get the order correct
35 Working with relational operators
36 Using logical operators
37 Understanding bitwise operators
38 Shifting bits
39 Exploring unary operators
40 Making a decision
41 Exploring the possibilities
42 Challenge Select an item
43 Solution Select an item
44 Using the ternary operator
45 Working with the switch-case structure
46 Creating a for loop
47 Setting up a while loop
48 Challenge Repeat some text
49 Solution Repeat some text
50 Nesting loops
51 Breaking out of a loop
52 Avoiding the goto keyword
53 Understanding functions
54 Prototyping a function
55 Challenge Writing a function
56 Solution Writing a function
57 Returning a value from a function
58 Challenge Returning a value
59 Solution Returning a value
60 Passing arguments to a function
61 Challenge Passing values
62 Solution Passing values
63 Retaining values in a function
64 Creating recursive functions
65 Next steps
Resolve the captcha to access the links!