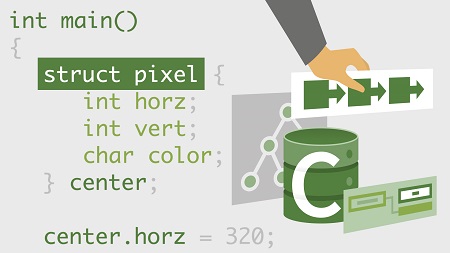
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 2h 57m | 462 MB
C is a foundational language for a variety of other programming languages, as well as an up-and-coming language for embedded systems. If you’re interested in broadening your knowledge of C, then this course is for you. Join instructor Dan Gookin as he takes a deep dive into data structures, pointers, and other key topics in this essential programming language. Dan digs into a variety of programming concepts such as arrays, structures, characters, and strings. He also goes over pointers—variables that hold a memory location—explaining how to use pointers to manipulate data, do pointer math, and more. He wraps up the course by sharing a few special techniques, including how to solve math puzzles with C.
Topics include:
- Working with arrays
- Building a structure
- Creating an array of structures
- Testing characters
- Working with strings in C
- Using pointers to manipulate data
- Manipulating files
- Using command-line arguments
- Working with time functions
Table of Contents
Introduction
1 Learn data structures and pointers in C
2 Using the exercise files
3 Configuring the IDE
Arrays and Structures
4 Understanding arrays
5 Working with arrays
6 Modifying arrays
7 Passing an array to a function
8 Working multi-dimensional arrays
9 Building a structure
10 Nesting structures
11 Creating an array of structures
12 Sending a structure to a function
13 Understanding a union
14 Challenge Presidents of the U.S.A.
15 Solution Presidents of the U.S.A.
Characters and Strings
16 Using single character IO
17 Testing characters
18 Working with character conversions
19 Understanding strings in C
20 Fetching string input
21 Sending string output
22 Reviewing printf() placeholders
23 Using printf() placeholders
24 Exploring string functions
25 Manipulating strings
26 Avoiding string problems
27 Challenge String IO and manipulation
28 Solution String IO and manipulation
Pointers
29 Understanding pointers
30 Using pointers to manipulate data
31 Doing pointer math
32 Comparing arrays and pointers
33 Allocating storage
34 Working with a pointer array
35 Using pointers in structures
36 Passing pointers to functions
37 Returning pointers
38 Challenge Create a pointer array
39 Solution Create a pointer array
Files and the Operating System
40 Reading from a file
41 Writing to a file
42 Working with raw data
43 Using random file access
44 Manipulating files
45 Exploring the path
46 Reading a directory
47 Getting file information
48 Using command line arguments
49 Calling the operating system
50 Challenge Read and store filenames
51 Solution Read and store filenames
The Interesting and Extraordinary
52 Solving math puzzles
53 Creating random numbers
54 Working with time functions
55 Sorting data
56 Debugging your code
57 Using a debugger
58 Writing your own header file
59 Mixing multiple source code files
60 Challenge Lotto simulation
61 Solution Lotto simulation
Conclusion
62 Next steps
Resolve the captcha to access the links!