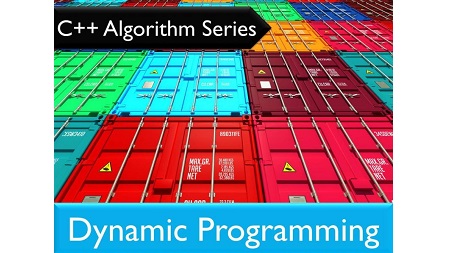
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 2h 14m | 358 MB
Know the basics of C++ and want to further sharpen your skills? Then follow along with C++ expert Advait Jayant in this next course in the C++ Algorithm Series, and master Dynamic Programming (DP).
The following six topics will be covered through a combination of lecture and hands-on to further your knowledge of dynamic programming in C++:
- Dynamic Programming Overview and the Top Down Approach. This first topic in the C++ Dynamic Programming course explains in detail the concept of dynamic programming. Follow along with Advait in this hands-on session, and apply dynamic programming to creating the Fibonacci Series in C++. Also practice the top down approach in C++ to dynamic programming using the Memoization optimization technique.
- Bottom Up Approach to Dynamic Programming. This second topic in the C++ Dynamic Programming course explains how to apply the bottom up approach to Dynamic Programming. Follow along with Advait in this hands-on session and apply dynamic programming to creating the Fibonacci Series using the bottom up approach in C++.
- One-Dimensional Approach to Dynamic Programming. This third topic in the C++ Dynamic Programming course explains how to apply the one-dimensional approach to Dynamic Programming. Follow along with Advait in this C++ hands-on session.
- Dynamic Programming in Practice: The Wine Problem. This fourth topic in the C++ Dynamic Programming course explains how to apply the two-dimensional approach in Dynamic Programming. Follow along with Advait in this hands-on session and solve the Wine Problem (a challenge in maximizing profit) using a combination of the bottom up approach and the Memoization optimization technique in C++.
- Dynamic Programming in Practice: The Grid Problem. This fifth topic in the C++ Dynamic Programming course explains how to apply dynamic programming to the Grid Problem. Follow along with Advait in this hands-on session and solve the Grid Problem using the bottom up approach in C++.
- Dynamic Programming in Practice: The Rod Cutting Problem. This sixth topic in the C++ Dynamic Programming course explains how to apply dynamic programming to the Rod Cutting Problem. Follow along with Advait in this hands-on session and solve the Rod Cutting Problem using dynamic programming in C++.
Table of Contents
1 Dynamic Programming Overview and the Top Down Approach
2 Bottom Up Approach to Dynamic Programming
3 One-Dimensional Approach to Dynamic Programming
4 Dynamic Programming in Practice – The Wine Problem
5 Dynamic Programming in Practice – The Grid Problem
6 Dynamic Programming in Practice – The Rod Cutting Problem
Resolve the captcha to access the links!