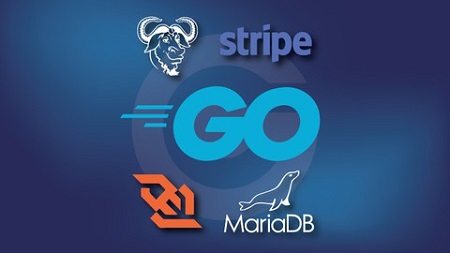
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 173 lectures (18h 41m) | 7.35 GB
Learn how to build a secure ecommerce application with Go (Golang)
This course is the followup to Building Modern Web Applications in Go. In this course, we go further than we did the first time around. We will build a sample E-Commerce application that consists of multiple, separate applications: a front end (which services content to the end user as web pages); a back end API (which is called by the front end as necessary), and a microservice that performs only one task, but performs it extremely well (dynamically building PDF invoices and sending them to customers as an email attachment).
The application will sell individual items, as well as allow users to purchase a monthly subscription. All credit card transactions will be processed through Stripe, which is arguably one of the most popular payment processing systems available today, and for good reason: developers love it. Stripe offers a rich API (application programming interface), and it is available in more than 35 countries around the world, and works with more than 135 currencies. Literally millions of organizations and businesses use Stripe’s software and APIs to accept payments, send payouts, and manage their businesses online with the Stripe dashboard. However, in many cases, developers want to be able to build a more customized solution, and not require end users to log in to both a web application and the Stripe dashboard. That is precisely the kind of thing that we will be covering in this course.
We will start with a simple Virtual Terminal, which can be used to process so-called “card not present” transactions. This will be a fully functional web application, built from the ground up on Go (sometimes referred to as Golang). The front end will be rendered using Go’s rich html/template package, and authenticated users will be able to process credit card payments from a secure form, integrated with the Stripe API. In this section of the course, we will cover the following:
- How to build a secure, production ready web application in Go
- How to capture the necessary information for a secure online credit card transaction
- How to call the Stripe API from a Go back end to create a paymentIntent (Stripe’s object for authorizing and making a transaction)
Once we have that out of the way, we’ll build a second web application in the next section of the course, consisting of a simple web site that allows users to purchase a product, or purchase a monthly subscription. Again, this will be a web application built from the ground up in Go. In this section of the course, we’ll cover the following:
- How to allow users to purchase a single product
- How to allow users to purchase a recurring monthly subscription (a Stripe Plan)
- How to handle cancellations and refunds
- How to save all transaction information to a database (for refunds, reporting, etc).
- How to refund a transaction
- How to cancel a subscription
- How to secure access to the front end (via session authentication)
- How to secure access to the back end API (using stateful tokens)
- How to manage users (add/edit/delete)
- How to allow users to reset their passwords safely and securely
- How to log a user out and cancel their account instantly, over websockets
Once this is complete, we’ll start work on the microservice. A microservice is a particular approach to software development that has the basic premise of building very small applications that do one thing, but do it very well. A microservice does not care in the slightest about what application calls it; it is completely separate, and completely agnostic. We’ll build a microserivce that does the following:
- Accepts a JSON payload describing an individual purchase
- Produces a PDF invoice with information from the JSON payload
- Creates an email to the customer, and attaches the PDF to it
- Sends the email
All of these components (front end, back end, and microservice) will be built using a single code base that produces multiple binaries, using Gnu Make.
What you’ll learn
- How to build a front end website using Go
- How to build a back end API using Go
- How to build multiple applications from a single code base
- How to build microservices in Go
- User authentication in Go
- API authentication using stateful tokens
- How to allow users to reset a password in a safe, secure manner
- How to integrate Stripe credit card processing with a Go back end
- Make one time or recurring payments with Stripe
- Best practices for making secure credit card transactions
Table of Contents
Introduction
1 Introduction
2 A bit about me
3 Mistakes. We all make them
4 How to ask for help
Setting up our environment
5 Install Go
6 Install an IDE
7 Get a free Stripe account
8 Install make
9 Install MariaDB
10 Getting a database client
Building a virtual credit card terminal
11 What we’re going to build
12 Setting up a (trivial) web application
13 Setting up routes and building a render function
14 Displaying one page
15 A better extension for Go templates and VS Code
16 Creating the form
17 Connecting our form to stripe.js
18 Client side validation
19 Getting the paymentIntent – setting up the back end package
20 Getting the paymentIntent – starting work on the back end api
21 Getting the paymentIntent – setting up a route and handler, and using make
22 Getting the paymentIntent – finishing up our handler
23 Updating the front end JavaScript to call our paymentIntent handler
24 Getting the payment intent, and completing the transaction
25 Generating a receipt
26 Cleaning up the API url and Stripe Publishable Key on our form
Selling a product online
27 What are we going to build
28 Create the database
29 Connecting to the database
30 Creating a product page
31 Creating the product form
32 Moving JavaScript to a reusable file
33 Modifying the handler to take a struct
34 Update the Widget page to use data passed to the template
35 Creating a formatCurrency template function
36 Testing the transaction functionality
37 Creating a database table for items for sale
38 Running database migrations
39 Creating database models
40 Working on database functions
41 Inserting a new transaction
42 Inserting a new order
43 An aside fixing a problem with calculating the amount
44 Getting more information about a transaction
45 Customers
46 Getting started saving customer and transaction information
47 Create the save customer database method
48 Saving the customer, transaction, and order from the handler
49 Running a test transaction
50 Fixing a database error, and saving more details
51 Redirecting after post
52 Simplifying our PaymentSucceeded handler
53 Revising our Virtual Terminal
54 Fixing a mistake in the formatCurrency template function
Setting up and charging a recurring payment using Stripe Plans
55 What are we going to build in this section
56 Creating a Plan on the Stripe Dashboard
57 Creating stubs for the front end page and handler
58 Setting up the form
59 Working on the JavaScript for plans
60 Continuing with the Javascript for subscribing to a plan
61 Create a handler for the POST request after a user is subscribed
62 Create methods to create a Stripe customer and subscribe to a plan
63 Updating our handler to complete a subscription
64 Saving transaction & customer information to the database
65 Saving transaction & customer information II
66 Displaying a receipt page for the Bronze Plan
Authentication
67 Introduction
68 Creating a login page
69 Writing the stub javascript to authenticate against the back end
70 Create a route and handler for authentication
71 Create a writeJSON helper function
72 Starting the authentication process
73 Creating an invalidCredentials helper function
74 Creating a passwordMatches helper function
75 Making sure that everything works
76 Create a function to generate a token
77 Generating and sending back a token
78 Saving the token to the database
79 Saving the token to local storage
80 Changing the login link based on authentication status
81 Checking authentication on the back end
82 A bit of housekeeping
83 Creating stub functions to validate a token
84 Extracting the token from the authorization header
85 Validating the token on the back end
86 Testing out our token validation
87 Challenge Checking for expiry
88 Solution to challenge
89 Implementing middleware to protect specfic routes
90 Trying out a protected route
91 Converting the Virtual Terminal post to use the back end
92 Changing the virtual terminal page to use fetch
93 Verifying the saved transaction
Protecting routes on the Front End and improving authentication
94 Writing middleware on the front end to check authentication
95 Protecting routes on the front end
96 Logging out from the front end
97 Saving sessions in the database
Mail and Password Resets
98 Password resets
99 Sending mail Part I
100 Mailtrap.io
101 Sending mail Part II
102 Creating our mail templates and sending a test email
103 Implementing signed links for our email message
104 Using our urlsigner package
105 Creating the reset password route and handler
106 Setting up the reset password page
107 Creating a back end route to handle password resets
108 Setting an expiry for password reset emails
109 Adding an encryption package
110 Using our encryption package to lock down password resets
Building Admin pages to manage purchases
111 Improving our front end and setting up an Admin menu
112 Setting up stub pages for sales and subscriptions
113 Updating migrations and resetting the database
114 Listing all sales database query
115 Listing all sales database function
116 Listing all sales writing the API handler and route
117 Listing all sales front end javascript
118 Displaying our results in a table
119 Making our table prettier, and adding some checks in JavaScript
120 Challenge Listing all Bronze Plan subscribers
121 Solution to challenge
122 Displaying a sale part 1
123 Displaying a sale part 2
124 Displaying a subscription
Refunds
125 Refunds from the Stripe Dashboard
126 Adding a refund function to our cards package
127 Creating an API handler to process refunds
128 Update the front end for refunds
129 Improving the front end
130 Adding UI components to the sales page
131 Updating status to refunded in the database
Cancelling Subscriptions
132 Capturing the subscription id
133 Adding a CancelSubscription function to our cards package
134 Creating a handler to cancel a subscription
135 Modifying the front end
136 Finishing up the front end
Paginating Data
137 Creating a database method to paginate all orders
138 Modifying the AllSales handler to use paginated data
139 Updating the all-sales.page.gohtml template
140 Improving pagination on the front end
141 Adding listeners to page navigation buttons
142 Taking user to correct page of data on click
143 How I implemented pagination on the all subscriptions page
Managing Users
144 Setting up templates to manage users
145 Adding routes and handlers on the front end
146 Writing the database functions to manage users
147 Creating a handler and route for all users on the back end
148 Updating the front end to call AllUsers
149 Displaying the list of users
150 Creating a user addedit form
151 Call the api back end to get one user
152 Populating the user form, and a challenge
153 Solution to challenge
154 Saving an edited user – part one
155 Saving an edited user – part two
156 Deleting a user
157 Removing the deleted users token from the database
158 Setting up websockets
159 Connecting to WebSockets from the browser
160 Logging the deleted user out over websockets
Microservices
161 What are microservices
162 Setting up a simple microservice
163 Receiving data with our micrsoservice
164 Generating an invoice as a PDF
165 Testing our PDF
166 Mailing the invoice
167 Call the microservice when a Widget is sold
168 Challenge
169 Solution
Validation
170 Setting up a validation package
171 Adding validation on the API back end
172 Modifying the front end javascript
Where to go next
173 gRPC, SOA, and more
Resolve the captcha to access the links!