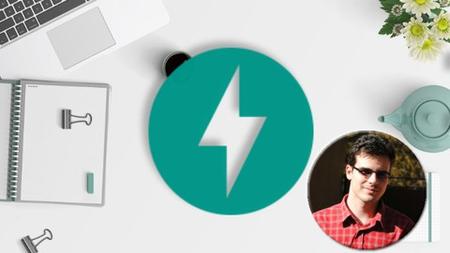
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 34 lectures (7h 1m) | 3.45 GB
Design a Restful API with FastAPI, write unit-tests, use MongoDB. Learn about containerization, middleware & auth.
Join this course in order to learn to build a Movie Tracking API from scratch using Python with FastAPI and MongoDB. During the course you will learn web application development basics, how to structure a Python project, how to apply design patterns and to write unit tests for your API.
You will also learn how to use the tools that professional Python developers use in their day to day work and to improve your professional workflow.
The course uses Python 3.10 and Fast API.
What you’ll learn in this course:
- How to build a real world Movie Tracking API.
- How to write unit tests with Pytest.
- How to structure a Python project.
- How to improve your development workflow with Docker and compose plugin.
- How apply design patterns such as the Repository.
- How to code an in-memory database from scratch.
- How to work with MongoDB from Python.
- How to write and think about the applications RESTful endpoints.
- How to handle pagination.
- How to work with Fast API’s dependency injection feature.
- How to handle configuration for your Fast API project.
- How to containerize a Python project.
- How to deploy the solution on Kubernetes.
Table of Contents
Introduction
1 Introduction
Environment Setup
2 Installing Python & Configuring IDE
3 Installing Docker and Docker-Compose
4 Installing Insomnia
5 Recap
Docker Basics
6 Docker Basics
7 Docker Recap
MongoDB Basics
8 Find and Insert
9 Object Ids
10 Update, Count and Delete
Web API Project Structure
11 Project Setup
12 Basic API Endpoints
Storage Layer
13 CRUD and Repository Pattern
14 In Memory Repository
15 Unit Testing the In Memory Repository
16 MongoDB Repository Pattern
17 Unit Testing the MongoDB Repository
18 Wrap-up
Movie Tracker API
19 Movie Tracker Create Endpoint
20 Movie Tracker Read Endpoint
21 Movie Tracker Update Endpoint
22 Movie Tracker Delete Endpoint
23 Implementing Pagination
24 Unit Testing the endpoints
Fast API Middleware
25 What is Middleware
26 CORS Middleware
27 Prometheus Middleware
28 Writing Custom Middleware
Authentication
29 Basic Authenticatioon
30 Authentication JWT
Deployment
31 Dockerizing the application
32 Deployment on Kubernetes
33 Visualizing Metrics with Grafana
Wrap Up
34 Wrap-Up
Resolve the captcha to access the links!