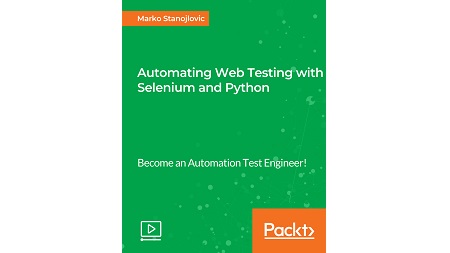
English | MP4 | AVC 1920×1080 | AAC 48KHz 2ch | 3h 47m | 811 MB
Developing reliable and accurate web testing automation with Selenium WebDriver and Python
Selenium WebDriver is one of the most sought-after skills on this planet, and you can learn it today. One of the worst drags in the application development process is the testing phase. And the pressure to launch an application as early as possible may force you to cut corners in the time-consuming manual testing phase, resulting in half-baked products and end-users impeded by many glitches.
If you’re drowning in a never-ending collection of regression test cases and need to automate them, you’re in the right place. This course will make your life as a developer easier and using Selenium will change the mundane way in which you run the exact same tests day in and day out.
Test automation with Selenium and Python, or developing scripts for running automated test commands against a range of browsers, will be more cost-effective, accurate, and faster than manual testing. The lack of manual intervention will diminish the possibility of errors and you will be able to find bugs at an early stage, making the process more reliable. By automating your web testing, you will be able to run tests on multiple devices simultaneously, which is impossible with manual testing.
By the end of this course, you will be well on the way to becoming a test automation specialist. Automating your testing with Python and Selenium will offer a highly efficient way to generate test scripts, validate their functionality, and reuse such scripts in an automated framework.
This comprehensive course is packed with step-by-step instructions, examples, and points to remember. You will create a Selenium WebDriver framework from scratch by using the Page Object Model design pattern and taking advantage of Python unittest and reap the benefits that Selenium has to offer in automating the tedious web testing phase.
What You Will Learn
- Create a Selenium WebDriver framework from scratch by using the unittest and PyTest frameworks
- Make unit and functional tests of web applications more efficient and reliable
- Automate various UI elements such as buttons, links, radio buttons, checkbox and tables
- Create robust tests with Selenium WebDriver and run tests on a Selenium Grid with Docker containers
- Implement the POM (Page Object Model) design pattern and model a web page with it
- Work with the SOLID, KISS, DRY, YAGNI design patterns to enhance the functionality of your code from beginner to professional
- Scale your tests and make them run in parallel by using different methods like Selenium Grid or Python multiprocessing module
- Configure Selenium WebDriver for Chrome, Firefox, and PhantomJS and run and manage tests in all browsers and headless
- Develop a test suite of an example web application in a stable, reliable, and efficient manner
Table of Contents
Setting Up Your Environment
1 The Course Overview
2 Selenium as a Testing Tool
3 Web Page Structure, DOM, and Finding Elements
4 Setting Up a Python 3 Test Environment and Visual Studio Code IDE
5 Installing ChromeDriver and Creating and Running Selenium Tests
6 Troubleshooting a Failed Test and Examining Results
Caching DOM Elements
7 Selecting Locators in the DOM
8 Handcrafting CSS and XPath Locators
9 Automating Buttons, Checkboxes, and Input Text Areas
10 Automating Drop-Down Menus and Lists
11 Automating Radio Buttons and Radio Groups
12 Automating Tables
13 Assertions
14 Implicit and Explicit Wait
Automating Browser Related Actions
15 Executing JavaScript Code
16 Capturing Screenshots
17 Browser Window and Navigation
18 Handling Cookies
19 WebDriver Events
Implementing the Page Object Model Design Pattern
20 POM Design Pattern
21 Test Example Without Using POM
22 Modeling a Web Page Testing with POM
23 Refactoring Test Code to Use POM
Other Design Patterns and Principles
24 Implementing SOLID Principles
25 KISS, DRY, YAGNI, and Other Principles
26 Working with Generic Software Design Patterns
Execution Scaling
27 Working with the Python unittest Module
28 Making Test Runs in Parallel
29 Selenium Grid
30 Setting Up a Selenium Grid with Docker Containers
Cross-Browser Testing
31 Configuring WebDriver for Headless Browsers
32 Running Tests in All Browsers
33 Managing the Test Results
34 Creating a Test Report
Creating a Test Suite
35 Suite Preparation
36 Login Page Test Cases
37 Submitting the Form Test Cases
38 Data Scraping Example
39 Putting It All Together
Resolve the captcha to access the links!