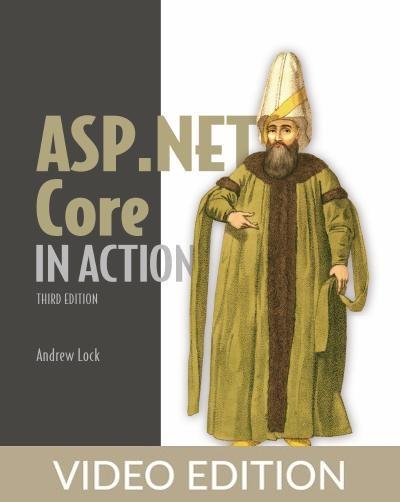
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 33h 15m | 4.62 GB
Build professional-grade full-stack web applications using C# and ASP.NET Core.
In ASP.NET Core in Action, Third Edition you’ll learn how to:
- Build minimal APIs for serving JSON to client-side applications
- Create dynamic, server-side rendered applications using Razor Pages
- User authentication and authorization
- Store data using Entity Framework Core
- Unit and integration tests for ASP.NET Core applications
- Write custom middleware and components
Fully updated to ASP.NET Core 7.0! In ASP.NET Core in Action, Third Edition Microsoft MVP Andrew Lock teaches you how you can use your C# and .NET skills to build amazing cross-platform web applications. This revised bestseller reveals the latest .NET patterns, including minimal APIs and minimal hosting. Even if you’ve never worked with ASP.NET, you’ll start creating productive cross-platform web apps fast. Illustrations and annotated code make learning visual and easy.
The ASP.NET Core web framework delivers everything you need to build professional-quality web applications. With productivity-boosting libraries for server-side rendering, secure APIs, easy data access and more, you’ll spend your time implementing features instead of researching syntax and tracking down bugs. This book is your guide.
ASP.NET Core in Action, Third Edition shows you how to create production-grade web applications with ASP.NET Core 7.0. You’ll learn from hands-on examples, insightful illustrations, and nicely explained code. Updated coverage in this Third Edition includes creating minimal APIs, securing APIs with bearer tokens, WebApplicationBuilder, and more.
What’s inside
- Minimal APIs for serving JSON
- Server-side rendering using Razor Pages
- Data access with Entity Framework Core
- Write custom middleware and components
Table of Contents
1 Getting started with ASP.NET Core
2 What types of applications can you build
3 Choosing ASP.NET Core
4 How does ASP.NET Core work
5 What you’ll learn in this book
6 Summary
7 Part 1 Getting started with minimal APIs
8 Understanding ASP.NET Core
9 Why ASP.NET Core was created
10 Understanding the many paradigms of ASP.NET Core
11 When to choose ASP.NET Core
12 Summary
13 Your first application
14 Creating your first ASP.NET Core application
15 Running the web application
16 Understanding the project layout
17 The .csproj project file Declaring your dependencies
18 Program.cs file Defining your application
19 Adding functionality to your application
20 Summary
21 Handling requests with the middleware pipeline
22 Combining middleware in a pipeline
23 Handling errors using middleware
24 Summary
25 Creating a JSON API with minimal APIs
26 Defining minimal API endpoints
27 Generating responses with IResult
28 Running common code with endpoint filters
29 Organizing your APIs with route groups
30 Summary
31 Mapping URLs to endpoints using routing
32 Endpoint routing in ASP.NET Core
33 Exploring the route template syntax
34 Generating URLs from route parameters
35 Summary
36 Model binding and validation in minimal APIs
37 Binding simple types to a request
38 Binding complex types to the JSON body
39 Arrays Simple types or complex types
40 Making parameters optional with nullables
41 Binding services and special types
42 Custom binding with BindAsync
43 Choosing a binding source
44 Simplifying handlers with AsParameters
45 Handling user input with model validation
46 Summary
47 Part 2. Building complete applications
48 An introduction to dependency injection
49 Creating loosely coupled code
50 Using dependency injection in ASP.NET Core
51 Adding ASP.NET Core framework services to the container
52 Using services from the DI container
53 Summary
54 Registering services with dependency injection
55 Registering services using objects and lambdas
56 Registering a service in the container multiple times
57 Understanding lifetimes When are services created
58 Resolving scoped services outside a request
59 Summary
60 Configuring an ASP.NET Core application
61 Building a configuration object for your app
62 Using strongly typed settings with the options pattern
63 Configuring an application for multiple environments
64 Summary
65 Documenting APIs with OpenAPI
66 Testing your APIs with Swagger UI
67 Adding metadata to your minimal APIs
68 Generating strongly typed clients with NSwag
69 Adding descriptions and summaries to your endpoints
70 Knowing the limitations of OpenAPI
71 Summary
72 Saving data with Entity Framework Core
73 Adding EF Core to an application
74 Managing changes with migrations
75 Querying data from and saving data to the database
76 Using EF Core in production applications
77 Summary
78 Part 3. Generating HTML with Razor Pages and MVC
79 Creating a website with Razor Pages
80 Exploring a typical Razor Page
81 Understanding the MVC design pattern
82 Applying the MVC design pattern to Razor Pages
83 Summary
84 Mapping URLs to Razor Pages using routing
85 Convention-based routing vs. explicit routing
86 Routing requests to Razor Pages
87 Customizing Razor Page route templates
88 Generating URLs for Razor Pages
89 Customizing conventions with Razor Pages
90 Summary
91 Generating responses with page handlers in Razor Pages
92 Selecting a page handler to invoke
93 Accepting parameters to page handlers
94 Returning IActionResult responses
95 Handler status codes with StatusCodePagesMiddleware
96 Summary
97 Binding and validating requests with Razor Pages
98 From request to binding model Making the request useful
99 Validating binding models
100 Organizing your binding models in Razor Pages
101 Summary
102 Rendering HTML using Razor views
103 Creating Razor views
104 Creating dynamic web pages with Razor
105 Layouts, partial views, and ViewStart
106 Summary
107 Building forms with Tag Helpers
108 Creating forms using Tag Helpers
109 Generating links with the Anchor Tag Helper
110 Cache-busting with the Append Version Tag Helper
111 Using conditional markup with the Environment Tag Helper
112 Summary
113 Creating a website with MVC controllers
114 Your first MVC web application
115 Comparing an MVC controller with a Razor Page PageModel
116 Selecting a view from an MVC controller
117 Choosing between Razor Pages and MVC controllers
118 Summary
119 Creating an HTTP API using web API controllers
120 Applying the MVC design pattern to a web API
121 Attribute routing Linking action methods to URLs
122 Using common conventions with [ApiController]
123 Generating a response from a model
124 Choosing between web API controllers and minimal APIs
125 Summary
126 The MVC and Razor Pages filter pipeline
127 The Razor Pages filter pipeline
128 Filters or middleware Which should you choose
129 Creating a simple filter
130 Adding filters to your actions and Razor Pages
131 Understanding the order of filter execution
132 Summary
133 Creating custom MVC and Razor Page filters
134 Understanding pipeline short-circuiting
135 Using dependency injection with filter attributes
136 Summary
137 Part 4. Securing and deploying your applications
138 Authentication Adding users to your application with Identity
139 What is ASP.NET Core Identity
140 Creating a project that uses ASP.NET Core Identity
141 Adding ASP.NET Core Identity to an existing project
142 Customizing a page in ASP.NET Core Identity’s default UI
143 Managing users Adding custom data to users
144 Summary
145 Authorization Securing your application
146 Authorization in ASP.NET Core
147 Using policies for claims-based authorization
148 Creating custom policies for authorization
149 Controlling access with resource-based authorization
150 Hiding HTML elements from unauthorized users
151 Summary
152 Authentication and authorization for APIs
153 Understanding bearer token authentication
154 Adding JWT bearer authentication to minimal APIs
155 Using the user-jwts tool for local JWT testing
156 Describing your authentication requirements to OpenAPI
157 Applying authorization policies to minimal API endpoints
158 Summary
159 Monitoring and troubleshooting errors with logging
160 Adding log messages to your application
161 Controlling where logs are written using logging providers
162 Changing log verbosity with filtering
163 Structured logging Creating searchable, useful logs
164 Summary
165 Publishing and deploying your application
166 Publishing your app to IIS
167 Hosting an application in Linux
168 Configuring the URLs for your application
169 Summary
170 Adding HTTPS to an application
171 Using the ASP.NET Core HTTPS development certificates
172 Configuring Kestrel with a production HTTPS certificate
173 Enforcing HTTPS for your whole app
174 Summary
175 Improving your application’s security
176 Protecting from cross-site request forgery (CSRF) attacks
177 Calling your web APIs from other domains using CORS
178 Exploring other attack vectors
179 Summary
180 Part 5. Going further with ASP.NET Core
181 Building ASP.NET Core apps with the generic host and Startup
182 The Program class Building a Web Host
183 The Startup class Configuring your application
184 Creating a custom IHostBuilder
185 Understanding the complexity of the generic host
186 Choosing between the generic host and minimal hosting
187 Summary
188 Advanced configuration of ASP.NET Core
189 Using DI with OptionsBuilder and IConfigureOptions
190 Using a third-party dependency injection container
191 Summary
192 Building custom MVC and Razor Pages components
193 View components Adding logic to partial views
194 Building a custom validation attribute
195 Replacing the validation framework with FluentValidation
196 Summary
197 Calling remote APIs with IHttpClientFactory
198 Creating HttpClients with IHttpClientFactory
199 Handling transient HTTP errors with Polly
200 Creating a custom HttpMessageHandler
201 Summary
202 Building background tasks and ser vices
203 Creating headless worker services using IHost
204 Coordinating background tasks using Quartz.NET
205 Summary
206 Testing applications with xUnit
207 Creating your first test project with xUnit
208 Running tests with dotnet test
209 Referencing your app from your test project
210 Adding Fact and Theory unit tests
211 Testing failure conditions
212 Summary
213 Testing ASP.NET Core applications
214 Unit testing API controllers and minimal API endpoints
215 Integration testing Testing your whole app in-memory
216 Isolating the database with an in-memory EF Core provider
217 Summary
218 Preparing your development environment
219 Choosing an IDE or editor
Resolve the captcha to access the links!