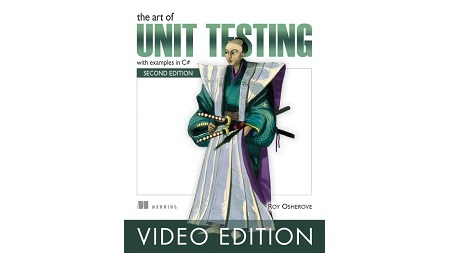
English | MP4 | AVC 1280×720 | AAC 44KHz 2ch | 8h 39m | 1.59 GB
The Art of Unit Testing, Second Edition guides you step by step from writing your first simple tests to developing robust test sets that are maintainable, readable, and trustworthy. You’ll master the foundational ideas and quickly move to high-value subjects like mocks, stubs, and isolation, including frameworks such as Moq, FakeItEasy and Typemock Isolator. You’ll explore test patterns and organization, working with legacy code, and even “untestable” code. Along the way, you’ll learn about integration testing and techniques and tools for testing databases and other technologies.
Inside:
- Create readable, maintainable, trustworthy tests
- Fakes, stubs, mock objects, and isolation (mocking) frameworks
- Simple dependency injection techniques
- Refactoring legacy code
The examples in the book/course use C#, but will benefit anyone using a statically typed language such as Java or C++.
Table of Contents
01 The basics of unit testing
02 Properties of a good unit test
03 Drawbacks of nonautomated integration tests compared to automated unit tests
04 What makes unit tests good
05 Test-driven development
06 The three core skills of successful TDD
07 A first unit test
08 Introducing the LogAn project
09 Loading up the solution
10 Writing your first test
11 Refactoring to parameterized tests
12 More NUnit attributes
13 Checking for expected exceptions
14 Testing results that are system state changes instead of return values
15 Using stubs to break dependencies
16 Determining how to easily test LogAnalyzer
17 Refactoring your design to be more testable
18 Dependency injection – inject a fake implementation into a unit under test
19 Simulating exceptions from fakes
20 Variations on refactoring techniques
21 Overcoming the encapsulation problem
22 Interaction testing using mock objects
23 The difference between mocks and stubs
24 A simple handwritten mock example
25 One mock per test
26 The problems with handwritten mocks and stubs
27 Isolation (mocking) frameworks
28 Dynamically creating a fake object
29 Simulating fake values
30 Testing for event-related activities
31 Advantages and traps of isolation frameworks
32 Digging deeper into isolation frameworks
33 How profiler-based unconstrained frameworks work
34 Values of good isolation frameworks
35 Ignored arguments by default
36 Isolation framework design antipatterns
37 Test hierarchies and organization
38 Anatomy of a build script
39 Triggering builds and integration
40 Mapping out tests based on speed and type
41 Ensuring tests are part of source control
42 Cross-cutting concerns injection
43 Using test class inheritance patterns
44 Creating test utility classes and methods
45 The pillars of good unit tests
46 Writing trustworthy tests
47 Avoiding logic in tests
48 Testing only one concern
49 Assuring code review with code coverage
50 Writing maintainable tests
51 Removing duplication
52 Enforcing test isolation
53 Avoiding multiple asserts on different concerns
54 Comparing objects
55 Writing readable tests
56 Asserting yourself with meaning
57 Integrating unit testing into the organization
58 Identify possible entry points
59 Ways to succeed
60 Aiming for specific goals
61 Realizing that there will be hurdles
62 Tough questions and answers
63 Why is the QA department still finding bugs
64 Working with legacy code
65 Writing integration tests before refactoring
66 Important tools for legacy code unit testing
67 Read Michael Feathers’s book on legacy code
68 Design and testability
69 Make classes nonsealed by default
70 Pros and cons of designing for testability
71 Alternatives to designing for testability
72 Example of a hard-to-test design
73 Summary
Resolve the captcha to access the links!